Integrating JSP/JSF and XML/XSLT: The Best of Both Worlds
The long awaited releases of JavaServer Faces TM (JSF) version 1.0 and JavaServer Pages TM (JSP) version 2.0 promise to transform the way J2EE developers build Web applications. Meanwhile, Extensible Stylesheet Language Transformations (XSLT) version 2.0 is in the final stages of specification, and many developers are seriously considering XML-based presentation layers.
Introduction
Orbeon's engineers constantly work with bleeding-edge XML and J2EE technologies and frequently publish papers and articles. They are the developers of Model 2X and XPL. These technologies are implemented in OXF, an advanced XML processing framework freely available for non-commercial use. Orbeon also provides consulting services. Visit Orbeon at www.orbeon.com |
The long awaited releases of JavaServer Faces TM (JSF) version 1.0 and JavaServer Pages TM (JSP) version 2.0 promise to transform the way J2EE developers build Web applications. Meanwhile, Extensible Stylesheet Language Transformations (XSLT) version 2.0 is in the final stages of specification, and many developers are seriously considering XML-based presentation layers, especially when the applications target multiple devices or require look and feel customization.
This article shows that JSF 1.0 and JSP 2.0 are the result of a logical evolution that started in the early days of servlet programming. With milestones such as JSP 1.1, Struts 1.0 and JSTL 1.0, server-side Java technology has progressively integrated many successful techniques from real-world development. The article then shows that the introduction of Model 2X, a combination of JSP technology and XML processing, is a continuation of this evolution and improves the flexibility of the presentation layer.
To better understand the concepts discussed in this article, you should understand the basics of JSP, XML, and Web application architecture. Some familiarity with the concepts of JSF is helpful.
NOTE: The version of the JSF specification used during the writing of this article is the JavaServer Faces 1.0 Specification Early Access Draft dated September 6, 2002. The specification may change in the future. The JSF implementation used is the Sun Reference implementation version 1.0 Early Access 2 (EA2).
All associated source code for this article can be downloaded at http://www.orbeon.com/model2x/tss
These are exciting times for Java developers. At the time of writing (January 2003):
- The JavaServer Pages 2.0 and Java Servlet TM 2.4 specifications are proposed final drafts
- The JavaServer Pages Standard Tag Library TM specification has been final since June 2002
- JavaServer Faces 1.0, the specification generating the most anticipation, is well under development
Even if you stay up to date, the abundance of specifications and acronyms may appear daunting. The good news is that all those technologies are related and designed to complement each other:
- Java Servlets are the foundation of the Java Web technologies. The Java Servlet specification addresses how applications are packaged and deployed and describes server-side components, known as servlets, used to generate dynamic content.
- JavaServer Pages (JSP) build on top of the servlet technology and provide a page template approach for generating textual content such as HTML.
- The JavaServer Pages Standard Tag Library (JSTL) is a set of standard JSP actions (also called tags) simplifying the development of JSP pages. Page authors use JSTL to build advanced page logic without knowing Java or other scripting languages.
- JavaServer Faces (JSF) provides an extensible, component-based, tools-friendly server-side UI framework that integrates nicely with JSP and JSTL.
The following figure illustrates the dependencies between the technologies:
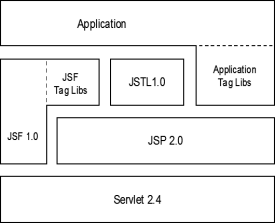
Figure 1: Technology Stack
Once Upon a Time...
Before the first JSP implementations, server-side Java applications generated HTML by embedding strings in Java code. Example 1 illustrates the generation of a simple HTML table from a servlet.
This code:
MyTableData tableData = MyDAO.queryData(); PrintWriter writer = response.getWriter(); writer.println("<table border="1">"); for (int i = 0; i < tableData.getData().length; i++) { writer.println("<tr>"); writer.println("<td>"); writer.println(tableData.getData()[i]); writer.println("</td>"); writer.println("</tr>"); } writer.println("</table>");
Generates this result:
Item 0 |
Item 1 |
Item 2 |
Item 3 |
Example 1: A Servlet Generating HTML
This model has the following problems:
- The inclusion of HTML strings in Java code is heavy and difficult to read
- Java code needs to be recompiled, packaged and deployed every time the static strings are changed
Many pages contain a large amount of static, non-generated text that needs to be editable, and a smaller amount of dynamically generated content. Because of this, most real-world solutions to these problems are based on the mechanisms of page templates. A template system makes it more natural to interleave fixed template text and dynamic actions. The most popular template system currently used in the Java world is JSP.
The Early Days of JSP
With JSP, HTML and Java code are written in a JSP file, also called a JSP page. A JSP engine loads and compiles JSP pages on the fly. This makes the development process easier. In addition, no Java code is required to generate static template text. Only dynamic actions require Java.
Example 1 can be rewritten as the following JSP 1.1 fragment:
<% MyTableData tableData = MyDAO.queryData(); %> <table border="1"> <% for (int i = 0; i < tableData.getData().length; i++) { %> <tr> <td> <%= tableData.getData()[i] %> </td> </tr> <% }%> </table>
Example 2: Displaying a Table with JSP
The HTML code is written as template text. The Java code is encapsulated in constructs known as scriptlets. The mix of languages is at best cryptic and out of reach for most non-Java developers. This makes it difficult to separate tasks and roles during development.
In Example 2, the business logic is called directly from the JSP page. Often developers even implement some or all the business logic in the JSP page. The way the page interacts with the business logic is not specified, much less enforced. For large projects this behavior leads to code that is not modular and is difficult to maintain unless developers are very disciplined.
Example 2 illustrates that the following issues must be addressed:
- The legibility of the JSP page itself
- The lack of model to separate business logic and presentation logic
The JSP 1.1 specification was finalized in November 1999. JSP best practices have significantly evolved since JSP 1.1, when they were virtually non-existent, but this style of JSP pages is still widespread. To resolve the issues of JSP 1.1, it is necessary to build frameworks on top of the JSP technology.
Struts and MVC
Struts is one of the first frameworks based on JSP that implements infrastructure addressing these issues:
- Providing tag libraries that help perform conditionals, iterations, and data selection that enhance the legibility of JSPs. The
bean
andlogic
tag libraries are used to accomplish these tasks. - Using the MVC (Model/View/Controller) architecture. With MVC, business logic and presentation logic are separate.
In Struts, the MVC architecture works on a page basis. It is implemented with a hybrid architecture, known as Model 2, involving the cooperation of a servlet and a JSP file.
The following figure explains the relationship between the different parts of MVC in Struts: the model, the view and the controller. Struts provides the controller servlet. The model is implemented in an action class that implements or calls business logic, and the view is implemented in a JSP page. You can still implement business logic in the JSP page, but the practice is discouraged.
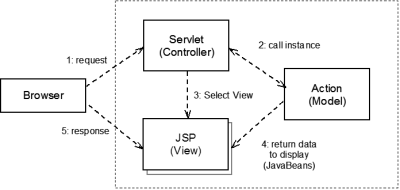
Figure 2: Struts, MVC and Model 2
The MVC architecture is important because it leads to a very natural modularization of the code and can benefit a group of developers or a single developer. When several developers work together, it is easier to separate the roles: one developer can concentrate on dealing with business logic, while another takes care of building the actual Web page. For more information about MVC, see the references at the end of this article.
Example 3 shows the JSP fragment from Example 2 rewritten using Struts:
<table border="1"> <logic:iterate id="data" name="tableData" property="data"> <tr> <td> <bean:write name="data"/> </td> </tr> </logic:iterate> </table>
Example 3: Displaying a Table with Struts
The code calling the business logic is now implemented in a separate action class (not shown) that passes a JavaBean named tableData
to the JSP page. Example 4 shows what the bean looks like:
public class TableData { private String[] data; public String[] getData() { return data; } ... }
Example 4: Simple JavaBean
The name
and property
attributes of the logic:iterate
tag specify an iteration on the elements of the bean's data
property. The property
attribute also supports nested and indexed references that constitute a real precursor to the Expression Language (EL) used in JSP 2.0 and JSTL. Example 3 illustrates that by implementing MVC and providing adequate tag libraries you can eliminate Java code in a JSP file.
Enter JSTL and JSP 2.0
The Struts tag libraries and expression language have limitations. Consider the following example code, which constructs an HTML table with an additional column and alternating row colors:
This code:
<% MyTableData tableData = MyDAO.queryData(); %> <table border="1"> <% for (int i = 0; i < tableData.size(); i++) { String cellColor = (i % 2 == 0) ? "gray" : "white"; %> <tr> <td bgcolor="<%= cellColor %>"> <%= i %> </td> <td bgcolor="<%= cellColor %>"> <%= tableData.get(i) %> </td> </tr> <% }%> </table>
Generates this result:
0 | Item 0 |
1 | Item 1 |
2 | Item 2 |
3 | Item 3 |
Example 5: Complex Example With Scriptlets
In Example 5, it is not possible to replace all scriptlets with Struts tags, because the iteration involves a data element, a position index, and a calculation that the Struts tag libraries are unable to handle.
Solutions to these limitations are introduced with JSTL and JSP 2.0. JSTL is essentially a better thought-out version of the Struts tag libraries. The set of tags is more consistent and complete. JSTL introduces an Expression Language (EL) loosely based on JavaScript and XPath. JSP 2.0 also natively supports this EL.
In addition to native EL support, JSP 2.0 presents the following new features:
Feature | Description |
---|---|
Expression Language API | Tag library implementers can easily leverage the JSP 2.0 EL implementation. |
New facilities to implement custom JSP actions |
|
Enhanced configurability | It is easy to explicitly disable the use of scriptlets in a single page or in a complete application. |
There are additional benefits associated with using JSTL and the EL:
Benefit | Description |
---|---|
Consistency with XML and HTML | The JSTL/EL combo produces JSP pages that look like XML documents. The result is not only easier to read, but also easier to process by development tools. Graphical tools can, for example, detect or insert an iterator tag, which is much more complex if the iteration is written in Java. |
Language constraints | The EL limits its scope to expressions. Tag libraries provide a well-defined set of actions. This can be beneficial because Java tempts Web page authors to break the MVC model by writing business logic in the page. |
Example 5 can be entirely rewritten using JSTL tag libraries and JSP 2.0 as shown in Example 6:
<table border="1"> <c:forEach items="${tableData.data}" var="currentData" varStatus="status"> <c:set var="cellColor"> <c:choose> <c:when test="${status.index % 2 == 0}">gray</c:when> <c:otherwise>white</c:otherwise> </c:choose> </c:set> <tr> <td bgcolor="${cellColor}"> ${status.index} </td> <td bgcolor="${cellColor}"> ${currentData} </td> </tr> </c:forEach> </table>
Example 6: Sample Written Using JSTL and JSP 2.0
The latest specifications clearly favor the writing of script-less pages and mark a turning point of JSP development. If you follow the best practices, JSP pages change from what purists considered a shapeless mix of HTML "tag soup" and Java scriptlets to almost pure namespace-aware XML files. The result is a more consistent syntax, less work for the page author, easier integration with tools and more control over the separation of business logic and presentation logic.
The Promise of JSF
For simple dynamic Web pages with limited user interaction, you may not need more functionality than that provided by JSP 2.0, JSTL, and MVC. But when it comes to developing complex Web-based user interfaces, you have to do a lot of work to extract request parameters, validate and process them, render them back to HTML controls, and so on. The process is tedious and error-prone.
Desktop applications have long used hierarchical component-based MVC frameworks such as Swing to alleviate the difficulty of building user interfaces. Such frameworks expose the elements of a user interface as components organized in container/containee relationships. This approach lends itself to using graphical tools to build user interfaces. It also allows component reuse and a better separation of roles during development.
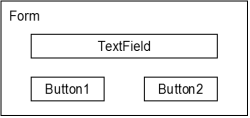
Figure 3: Form containing controls
Web applications usually have a reduced set of controls and limited interactivity, but otherwise do not differ much from desktop applications. It is natural to try to apply the model used on the desktop to the server-side world. This is the solution chosen by the JSF expert group. Server-side applications differ enough from desktop applications that JSF needs a new processing model and API instead of using the model and API from Swing.
Like Struts, JSF follows the MVC architecture. It provides a controller servlet, and allows the separation of the view, using renderers, and the model, using component classes and an event-based mechanism. With JSF, the MVC architecture works at the level of individual components.
Instead of writing Java code to assemble JSF components, as it is the case with Swing, developers can describe how components relate to each other using XML markup in JSP files. Using JSF in conjunction with JSP is optional, but it is expected that this will be the preferred technique. For example, JSF ships with a set of standard user interface components that can be used from JSP with the Standard JSF Tag Library. This allows web page authors who are not fluent in Java to tackle the task of developing complex user interfaces. It also makes it easier to implement graphical tools. An example is discussed later in this article.
When used with JSP, JSF can be considered a Model 2 architecture because of the use of both a controller servlet and JSP pages.
JSF promises reuse, separation of roles, easy to use tools to lower the barrier of entry and shorten the time needed to implement server-side user interfaces.
The rest of this article concentrates on specific rendering aspects of JSF. For more information about the JSF architecture, including its APIs and processing model, see the references at the end of this article.
JSF Rendering
Rendering a user interface consists of generating information to make it visible to the user. In server-side programming, the information usually consists of HTML, CSS and JavaScript. Rendering involves sending a stream of characters to a Web browser.
Rendering a JSF hierarchy is a recursive process in which each component, written in Java, has an opportunity to render itself. JSF provides a couple of options:
- Rendering directly from the components. It is not possible to modify the style of the rendering without modifying the code of the component itself.
- Delegating to separate renderer classes grouped in an entity called a RenderKit. The code of the component does not need modification, but changing the style still requires modifying Java code in the RenderKit.
Both of the rendering options are not template-based and, like in the days of the first servlets, require non-standard solutions or HTML generation from Java code. The renderers shipped with the early access version of JSF are implemented this way. Component developers have to be involved whenever any change in rendering is required. This is a serious and almost incomprehensible step back, given the separation of roles increasingly promoted by JSP.
HTML has a companion language, CSS, designed especially for styling. CSS could solve the JSF rendering limitations, provided CSS support is available in the HTML RenderKit for the Standard User Interface Components of JSF. For example, the background color of a button can be chosen with a style
attribute in a CSS-enabled browser:
<input type="button" value="Button 1" style="color: red"/> <input type="button" value="Button 2" style="color: blue"/>
Example 7: Styling With CSS
Using CSS solves part of the styling problem by allowing the rendering code of the components to be left unchanged even if different rendering aspects are changed. This approach has the following limitations:
- You cannot control every aspect of styling with CSS.
- Not every deployed Web browser supports all of the CSS specification.
To illustrate the first point, consider that you may want every table in an application to alternate row colors, as shown in Examples 5 and 6. CSS has no support for describing such behavior.
For Web browsers that do not support the latest versions of CSS, a popular HTML trick that does not use CSS consists in creating table borders by embedding tables.
The following code:
<table cellpadding="0" cellspacing="0" border="0" bgcolor="red"> <tr> <td> <!-- Original table --> <table width="100%"> <tr> <td bgcolor="white">1.1</td> <td bgcolor="white">1.2</td> </tr> <tr> <td bgcolor="white">2.1</td> <td bgcolor="white">2.2</td> </tr> </table> </td> </tr> </table>
Generates this result:
|
Example 8: Embedding Tables
To handle such cases with JSTL, code has to be duplicated in every JSP page of the application. If developers have to switch between different styles several times during development, for example to cope with changing requirements, changes have to be repeated throughout the application. With JSF, a single custom component can be built, but this means that you must write complex Java code. Neither technology helps much for implementing this type of style techniques.
At least two solutions are available:
- Implementing JSF renderers in template files similar to the JSP 2.0 tag files. Neither JSF nor JSP support such a model and it is unlikely that JSF rendering will change significantly before the release of the final version of JSF 1.0.
- Using XML processing. This solution, known as Model 2X, is discussed in the next section.
Model 2X
Architecture
The most important aspect of Model 2X is the use of XML transformation languages, such as XSL Transformations (XSLT), in conjunction with servlets and JSP. The following figure illustrates the basic Model 2X architecture:
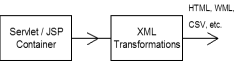
Figure 5: Model 2X Architecture
The output of a servlet or JSP is usually sent directly to a Web browser. With Model 2X, the output goes through a stage of XML transformations before reaching the Web browser. In order to understand this, it is important to look at the relationship of JSP to the XML world.
JSP and XML
JSP was designed to be able to produce any text format, such as HTML, Comma-Separated Values (CSV) and Cascading Style Sheets (CSS). For this reason you can use JSP to generate XML documents. The caveat is that JSP does not enforce well-formed XML output. To generate well-formed XML, you have to take the following precautions:
- Any template text markup present directly in the JSP must be well-formed
- Tag libraries must generate well-formed XML
Consider for example the following JSP fragment containing valid HTML tags:
<body> <hr> </body>
Because the hr
element is not closed, the output of the JSP page is not well-formed XML. It is easy to modify the code as shown below:
<body> <hr/> </body>
An easy way to make sure that template text is well-formed XML is to write, instead of regular JSP pages, what Sun calls JSP documents. A JSP document is simply the XML version of a JSP page. JSP constructs such as <% %>
and <%@ %>
are not XML compliant. In a JSP document, they are replaced with XML alternatives, such as <jsp:directive.include>
and <jsp:scriptlet>
tags. Writing JSP documents is not a requirement for generating well-formed XML from JSP, but because the JSP engine makes sure that the document is XML compliant, it signals many errors upfront.
Tag libraries often generate HTML. This is the case of the Struts html
tag library and of the Standard JSF Tag Library. When such tag libraries are used, they must be modified to generate XML instead of HTML. With JSF, this can be done easily by writing an XML renderer to replace the existing HTML renderer. This is discussed in more detail later in this article.
The result of executing a JSP page still results in a stream of characters, even when it is XML compliant. There is no provision to make sure that the output is valid or even well-formed XML. If tools need to process the JSP output as XML, as shown in Model 2X, a parsing step is required.
XSLT
Once XML is generated by a JSP page, it is ready for consumption by other tools. One such tool is XSLT, a functional language designed to perform transformations on XML documents.
A simple XSLT transformation can be represented this way:
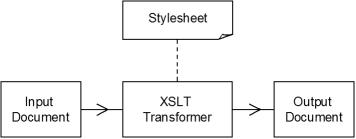
Figure 4: Simple XSLT Transformation
The result of an XSLT transformation can be XML, HTML, or any arbitrary text format.
Version 1.0 of XSLT was released in November 1999. Since then, a lot of work has gone into the XSLT 2.0 specification. XSLT 2.0 is based on XPath 2.0, a new version of the XML expression language, more consistent, and contains a number of features missing in version 1.0 such as grouping and user-defined XPath functions.
An XSLT program is called a stylesheet. The terminology is explained clearly in the XSLT 2.0 draft specification:
"The term stylesheet reflects the fact that one of the important roles of XSLT is to add styling information to an XML source document, by transforming it into a document consisting of XSL formatting objects (see [XSL Formatting Objects]), or into another presentation-oriented format such as HTML, XHTML, or SVG."
XSLT is therefore a language explicitly designed to address the style issues mentioned above.
JSF and Model 2X
You are now ready to understand how XML and XSLT technologies can interact with JSP and JSF in Model 2X. The following JSP code fragment uses JSF and is inspired by the "guessNumber" example from the current JSF reference implementation. It contains HTML template code and JSF tags that build a simple form:
<%@ taglib uri="http://java.sun.com/j2ee/html_basic/" prefix="faces"%> <html> <head><title>Hello</title></head> <body> <h1>Hello</h1> <faces:usefaces> <faces:form id="helloForm" formName="helloForm"> <table> <tr> <td> <faces:textentry_input id="userNo" modelReference="UserNumberBean.userNumber"/> </td> <td> <faces:command_button id="submit" commandName="submit"/><p> </td> </tr> </table> <faces:validation_message componentId="userNo"/> </faces:form> </faces:usefaces> </body> </html>
Example 9: JSF Example
A possible output of executing the fragment using an XML renderer is the following well-formed XML document:
<html> <head><title>Hello</title></head> <body> <h1>Hello</h1> <form method="post" action="/guessNumber/faces/greeting.jsp"> <table> <tr> <td> <input type="text" name="/helloForm/userNo"/> </td> <td> <input type="submit" name="submit" value="submit"/> </td> </tr> </table> </form> </body> </html>
Example 10: Output of the JSF Example
When the JSP output is parsed and fed to an XSLT transformer, you can easily apply styles. The following figure illustrates the processing model, a special case of Model 2X:
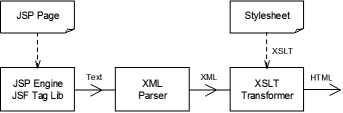
Figure 5: JSF and XSLT Rendering Model
For example, rendering every button in the application in red can be achieved using the following XSLT template:
<!-- Find all input elements with a "submit" type attribute --> <xsl:template match="input[@type = 'submit']"> <!-- Copy the element found --> <input> <!-- Copy all existing attributes --> <xsl:copy-of select="@*"/> <!-- Add a "style" attribute --> <xsl:attribute name="style">color: red</xsl:attribute> </input> </xsl:template>
Example 10: Adding Style Using an XSLT Template
XSLT templates are not limited to working on markup generated by JSF renderers. It is possible, for example, to generate the layout of a page using the same technique. Example 11 shows how you can insert a table containing a header row and a second row containing the original document body:
<!-- Match the body element --> <xsl:template match="body"> <!-- Copy the element found and set a background color --> <body bgcolor="white"> <table border="0"> <!-- Create a header row --> <tr> <td>Header</td> </tr> <tr> <td> <!-- Copy the actual body --> <xsl:apply-templates/> </td> </tr> </table> </body> </xsl:template>
Example 11: Creating a Page Layout
The benefits of Model 2X are:
Benefit | Description |
---|---|
Better modularity and flexibility | Styling can be centralized. A stylesheet can be written to handle all of an application's look and feel. The look and feel is reusable across applications. Different look and feels can be switched on demand at runtime within a same application. |
Quick turnaround | No modification to Java code is required. JSF renderers can be written once and for all. XSLT stylesheets can be reloaded while the application is running and changes are almost instant. |
Expressive power | XSLT is a language specifically adapted to manipulating XML and, consequently, XHTML. Possibilities of manipulations are endless. XPath is an expression language more evolved than the EL of JSP 2.0 and JSTL. |
Standard compliance | XSLT is a W3C standard and its adoption is widespread. This means that a lot of documentation and tools from multiple vendors are available, including several quality XSLT transformers for Java. XSLT transformations are supported by the Java API for XML Processing (JAXP). Xalan, a popular XSLT transformer, is bundled with J2SE 1.4, and all major application servers come with XSLT support. |
Pipelining | XSLT is adapted to processing in pipelines. XML pipelines are discussed in details below. |
Familiarity | Several JSTL constructs look like XSLT constructs, in particular conditionals and iterators. This makes XSLT easier to learn to somebody familiar with JSTL, and the other way around. |
The following limitations of Model 2X should be considered:
Limitation | Description |
---|---|
Additional processing | Model 2X introduces additional steps in the execution of the presentation logic. Most of the time, the performance hit is minimal and trading performance for flexibility is worth it. |
Learning curve | XSLT must be learned. For most purposes, XSLT is easy enough to learn that this should rarely be a problem. |
Discipline | Since JSP does not enforce well-formed XML output, it is up to the developer to do so. |
XML Pipelines
Using Model 2X introduces a new dimension to the JSP processing model because it involves several steps executed sequentially:
- The whole JSP page is processed. According to the regular JSP processing model, template text and tag libraries produce output.
- The output is parsed as XML.
- The XML processing is executed.
What is actually built is a two-stage pipeline. It is possible to assign well-defined roles to each stage:
- The first stage, based on JSP, builds the basic layout of the page, using simple HTML constructs, JSTL, other custom tag libraries, as well as JSF components.
- The second stage, based on XML transformation languages, applies all the styling information.
The pipeline concept makes the presentation layer of the Web application modular. Without changing the JSP pages, a pipeline can be extended to contain additional stages with different roles, for example:
Role | Description |
---|---|
Localization | Performing the localization in a separate pipeline stage allows localizing the output of JSP tag libraries, and advanced localization that changes the page layout. A Web page in Arabic may present its navigation bar on the right side of the screen instead of the left side. |
Multiple browser support | A stylesheet can generate output for Web browsers that support advanced rendering capabilities such as the latest versions of CSS, while another stylesheet can generate output for older browsers. |
Multiple device support | A separate pipeline can target completely different devices, such as PDAs or mobile phones. |
Inclusion | It is possible to build a page out of other JSP pages, including header, footer and main page body in a pipeline stage. |
A complete example of a pipeline is shown in Figure 6:
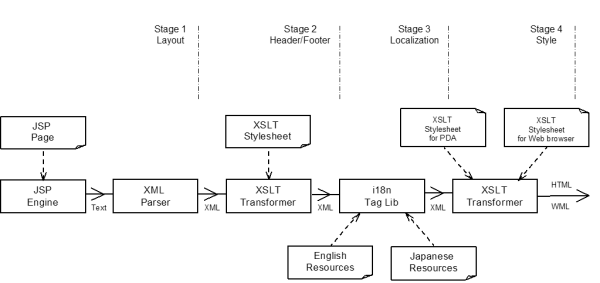
Figure 6: Example of a Pipeline
Implementing Model 2X
Implementing Model 2X with JSF requires:
- Creating an XML RenderKit for the JSF Standard User Interface Components.
- Hooking up an XML parser and an XSLT transformer (simple implementation) or XML pipeline (advanced implementation).
The following code illustrates the need for the first step. The JSF faces:textentry_input
tag does not generate well-formed XML output:
<INPUT TYPE="text" NAME="name" VALUE="value">
A JSF RenderKit that generates XML must generate well-formed XML elements and attributes. In addition it should follow the XHTML convention of creating elements in lowercase:
<input type="text" name="name" value="value"/>
The current reference implementation of JSF does not ship with an XML RenderKit, but it is simple for a Java developer familiar with JSF to write such a renderer. A sample RenderKit is included with this article.
The hookup of an XML parser and XSLT transformer can be done with the following methods:
- Servlet filters. JSP pages do not require any special attention besides generating well-formed XML.
- Tag libraries. A tag encapsulating the entire page must be inserted into every JSP page. You can use the JSP 2.0 prelude and coda mechanism to avoid the code duplication.
You can build the XML pipeline logic based on DOM or SAX. You can create custom code or use existing solutions such as OXF or Cocoon.
For more details, see the sample source code available with this article.
Conclusion
Over the last few years, JSP technology has matured. Best practices have emerged as a result of the use of JSP in real-life applications, leading to numerous enhancements to the core of JSP technology and to the development of new specifications such as JSTL. JSF introduces a long awaited user interface component model, but JSF exhibits limitations in its rendering architecture. Many developers may consider these limitations a conceptual step back.
Java developers are familiar with XML technologies, but they do not necessarily know how XML can be used to enhance a Web application's presentation layer. Model 2X, a simple architecture building on the strengths of JSP, JSF and XML technologies, alleviates the limitations of the JSF rendering model and provides a flexible presentation layer to J2EE applications.
In spite of the slow convergence of JSP towards XML, JSP 2.0 still shies away from fully integrating XML standards. In the future, JSP should complete this evolution by adding Model 2X to the core of the JSP engine.
References
JavaServer Pages (JSP)
http://java.sun.com/products/jsp/
Servlet Technology
http://java.sun.com/products/servlet/
JSTL specification
http://jcp.org/aboutJava/communityprocess/final/jsr052/
JavaServer Faces (JSF)
http://java.sun.com/j2ee/javaserverfaces/
JavaWorld article on Struts and XSLT
http://www.javaworld.com/javaworld/jw-02-2002/jw-0201-strutsxslt.html
A first look at JavaServer Faces, Part 1
http://www.javaworld.com/javaworld/jw-11-2002/jw-1129-jsf.html
XSLT 1.0 and XSLT 2.0 (Working Draft)
https://www.w3.org/TR/xslt, https://www.w3.org/TR/xslt20/
OXF
http://www.orbeon.com/oxf/
Model 2X
http://www.orbeon.com/model2x
Building the Pet Store with XML Pipelines
http://www.flashline.com/content/orbeon/pet_store.jsp
An Introduction to XML Pipelines
http://www.orbeon.com/oxf/whitepaper
Struts
http://jakarta.apache.org/struts/
The Model-View-Controller ('MVC') Design Pattern
http://jakarta.apache.org/struts/userGuide/introduction.html#mvc
Apache Xalan for Java
http://xml.apache.org/xalan-j/index.html
Saxon - The XSLT Processor
http://saxon.sourceforge.net/
Cocoon
http://xml.apache.org/cocoon/
About the authors
Erik Bruchez specializes in Web application architecture. He is co-architect of OXF, an XML-based application framework developed by Orbeon, Inc., a J2EE and XML consulting company he co-founded in 1999. Erik has led the design and implementation of several software platforms and applications, including Java middleware on set-top boxes and supply-chain management applications. Prior to Orbeon, Erik worked for Symantec Corp., where he contributed to the VisualCaf product line. Erik holds a MS/CS from the Swiss Institute of Technology (EPFL) in Lausanne, Switzerland.
Omar Tazi has held several senior management positions in high tech companies located in the heart of Silicon Valley including Symantec, Oracle and WebGain. Omar was a member of the Java Community Process Executive Committee, and was also actively involved in several JSRs (Java Specification Requests) as a member of the Expert Group. He is also a regular speaker at software event such as BEA eWorld and JavaOne. His main interests are Java 2 Platform, Enterprise Edition (J2EE), XML and Web services. Before crossing the Atlantic, Omar has held several research and teaching positions in the Swiss Federal Institute of Technology in Lausanne, specializing in Artificial Intelligence algorithms and Object Oriented Programming. He holds a M.S. in Computer Science and Electrical Engineering.