How to use Java's conditional operator ?:
What is the conditional Java ternary operator?
The Java ternary operator provides an abbreviated syntax to evaluate a true or false condition, and return a value based on the Boolean result.
The Java ternary operator can be used in place of if..else statements to create highly condensed and arguably unintelligible code.
Experienced developers love the brevity and conciseness the Java ternary operator brings to their code.
Junior developers, however, often find the Java ternary operator’s symbols and syntax confusing and difficult to understand.
What is the syntax of the Java ternary operator?
The syntax of the Java ternary operator is as follows:
(condition) ? (return if true) : (return if false);
You often see the Java ternary operator symbols ( ? : ) used in texts and tutorials as an abbreviation for the construct.
How do you use Java’s conditional operator?
To use the Java ternary operator, follow these steps:
- In round brackets, provide a condition that evaluates to true or false.
- Place a question mark after the round brackets.
- After the question mark, state the value to return if the condition is true.
- Add a colon.
- After the colon, specify the value to return if the condition is false.
Java ternary operator example
Here is a simple example of the Java ternary operator in action:
var result = ( Math.random() < 0 ) ? "negative" : "positive"; System.out.print("The random number is " + result); // Java ternary example output: The random number is positive
Ternary operator example explained
Here is an explanation of how the Java ternary operator example above works:
- The program declares a variable named result, and assigns it to the value returned by the Java ternary operator.
- The ternary operator evaluates to see if a randomly generated number is less than zero.
- If the number is less than zero, the condition is true and the program returns a “negative” text String.
- If the number is greater than zero, the condition is false and the program returns a “positive” text String.
Math.random() always generates a positive number, so this Java ternary operator example always returns this result: “The random number is positive”.
Java ternary if comparison
Note that the Java ternary operator example above could just have easily been written using an if…else statement.
The code below performs that exact same logic as the Java ternary operator example above.
var result = "";
if (Math.random() < 0) {
result = "negative";
} else {
result = "positive";
}
System.out.print("The random number is " + result);
How do you create a nested ternary operator in Java?
To create a nested Java ternary operator, set the statement to be evaluated on a true or false condition to be a new, independent ternary operator.
See if you can figure out the logic behind the following nested ternary operator example:
var players = 9;
var result = (players==11)? "baseball" : ((players==9) ? "footie" : "darts");
Nested ternary operator for 3 conditions example
The logic of the nested Java ternary operator example works like this:
- If there are 11 players, then play baseball
- If there are not 11 players, then:
- If there are nine players, play footie.
- Otherwise, just play darts.
Java’s ternary conditional operator return
The Java ternary operator must return a value. The statement evaluated in the body of the Java ternary operator cannot return void.
For example, the following code places a print statement after question mark, and causes a compile error: Type mismatch: cannot convert from void to String.
var result = ( Math.random() < 0 ) ? System.out.print("negative") : "positive" ;
Can Java’s conditional ternary operator return null?
The Java ternary operator return cannot be void. However, it can be null.
There are many instances where it makes sense to have a Java ternary operator return null. That is allowed, but void is not.
Left-hand side of a ternary operator assignment
You must use the ternary operator to assign a value to a variable, or pass its result as an argument to a method.
For example, the code below generates a compile error: The left-hand side of an assignment must be a variable.
( Math.random() < 0 ) ? "negative" : "positive";
If you assign the result of this Java ternary operator to a variable, it compiles successfully:
var result = (Math.random() < 0) ? "negative" : "positive";
The above line of code compiles without error because a variable named result has been declared to store the returned Java ternary operator’s result.
The Java ternary operator can also be used to pass an argument to a method. In the following example, the result of the Java ternary operator is not assigned to a variable; instead, the result is passed as an argument to the print() method:
System.out.print(( Math.random() < 0 ) ? "negative" : "positive");
if else and conditional operators
Any logic performed by a Java ternary operator could also be performed by an if..else statement.
Often in enterprise software development, developers with a wide range of experience maintain the code. That’s why I eschew the the Java ternary operator and prefer the use of if…else statements instead.
I appreciate the brevity and conciseness the Java ternary operator brings to the table, but the syntax can be intimidating to new developers. Nested ternary operators can become downright unwieldly.
If you have to choose between the Java ternary operator and if..else statements, go with the if..else.
Your fellow developers will appreciate it.
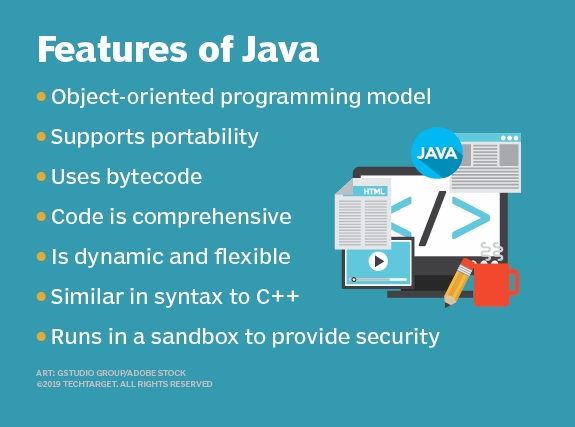
The Java ternary operator is just one of many constructs that help you write clean, concise and non-verbose Java code.
Java 8 ternary operator
A bug in a Java 8 support release caused the ternary operator to behave incorrectly in certain corner-cases. The bug has been fixed, and should not be a problem today.
More to the point, Java 8 is no longer supported by Oracle. Java 11 and Java 17 are the new LTS Java releases. The next LTS release, Java 21, is coming in 10 months.
If you are using Java 8, you must upgrade. There’s no excuse to run Java code on a JDK that is more than 10 years old.