Jenkins shared libraries in pipelines example
Jenkins shared libraries
You never want to pollute your Jenkins pipelines with superfluous Groovy code.
In fact, one of the reasons there’s been a push away from scripted towards declarative pipelines is to stop developers from coding complex Groovy routines where they shouldn’t be. A little bit of logic in a pipeline is okay. Complex programming? That should go in either a plugin or a Jenkins shared library.
For this Jenkins shared library example, I’ve created a simple script that checks to see if the UAT team has placed a file named approved.txt in a local folder of the Jenkins server. If that file is there, the Groovy logic returns true. If it isn’t, the logic returns false. When we make this Groovy script part of a Jenkins shared library, a declarative or scripted pipeline can use this logic and behave accordingly:
/* * jenkins-shared-library/src/com/mcnz/uatInput.groovy * https://github.com/learn-devops-fast/jenkins-shared-library.git */ package com.mcnz public class uatInput { def buildIsUatApproved() { def file = new File("C:/_tools/approved.txt") if (file.exists()){ return true; } else { println "Approval file does not exist." } return false; } }
With the Jenkins pipeline’s Groovy script saved to GitHub, the Jenkins shared library needs to be configured. This is done under the Global Pipeline Libraries section under the Manage Jenkins page of the admin console. The following properties need to be set for the shared pipeline library:
Your intro to GitHub Actions training course |
---|
Here’s how to get started with GitHub Actions:
Follow these tutorials and you’ll learn GitHub Actions fast. |
Name: shared-library Default version (branch): master GitHub URL: https://github.com/learn-devops-fast/jenkins-shared-library.git
Note that if you use a new GitHub repository for this Jenkins shared library example, your default branch may be named main, not master.
Test the Jenkins shared library
To test the Jenkins shared library from GitHub, create a new pipeline job and add a simple scripted pipeline that references the shared library, imports the class, creates an instances and calls one of the methods.
Be sure to uncheck the Use Groovy Sandbox option, or you may get an error such as the following, as sandbox security forbids certain Groovy operations:
MissingPropertyException: No such property: groovy.lang.Binding
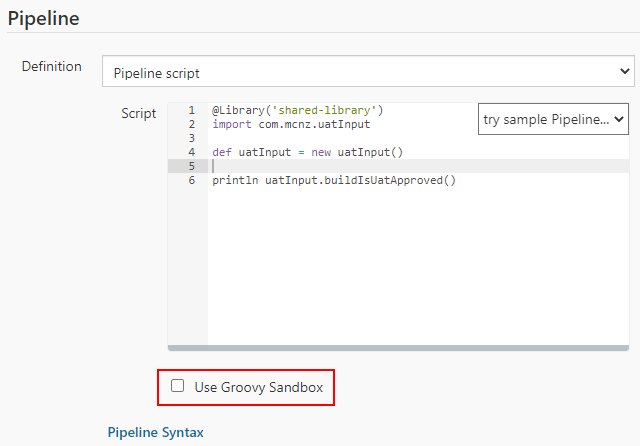
Test your shared Jenkins library with a simple scripted pipeline.
Declarative pipelines and shared libraries
This Jenkins shared library in GitHub is intended to be used in a conditional statement inside a declarative pipeline. Essentially, if the approval file exists, a build is allowed to continue. If not, the build stops.
This is just a simple example, but to implement such a process, a conditional declarative pipeline would look as follows:
@Library('shared-library') import com.mcnz.uatInput def uatInput = new uatInput() pipeline { agent any stages { stage ('Run only if approval exists') { when { expression { uatInput.buildIsUatApproved() } } steps { echo "The build has been approved!!!" } } } }
When this declarative pipeline runs, the build will be conditional upon whether the Groovy code from the Jenkins shared library indicates the approval file exists or not.
Jenkins shared pipeline example review
In summary, the steps to use a create a GitHub based, Jenkins shared library for pipelines are:
- Create GitHub repository with the Groovy scripts you wish to share
- Set up a Global Pipeline Library in the Configure System page of the Manage Jenkins tab
- Declare the shared library and import the classes at the start of your Jenkins pipeline scripts
- Disable the Groovy sandbox
- Run your CI/CD pipelines that use your shared Jenkins library
Never pollute your build pipelines with excessive amounts of logic and code. When you need to add some complex logic to your builds, a shared Jenkins library is the right way to make intricate logic available to your scripted and declarative pipelines.