Create your first Java AWS Lambda function in minutes
AWS Lambda Java example
If you’re a Java developer and you haven’t built an AWS Lambda function before, you’ll be amazed by how easy it is to get your first serverless application up and running.
To build your first Java AWS Lambda function, just create a simple class with an instance method that performs the following functions:
- Takes a String as an argument.
- Returns a String.
- Writes to the AWS CloudWatch logs with with println calls.
How Java-based AWS Lambda calls work
The AWS Lambda framework passes any text or JSON based payload data to your function through your instance method’s String argument.
The String your method returns is given to the AWS Lambda framework after a successful invocation.
Any information you write with the System’s println method is sent to AWS CloudWatch for future analysis.
AWS Lambda in Java example
The following Java code is more than sufficient to act as a fully compliant AWS Lambda function:
/* Example Java-based AWS Lambda function */ public class AwsLambdaFunctions { public String alterPayload(String payload) { System.out.println("We are in the Java AWS Lambda function!"); return payload.toLowerCase().replace('e', '3').replace('l', '1'); } }
Serverless Java code explained
The AWS Lambda code example really couldn’t be simpler. Here’s how it works:
- The Lambda environment passes any payload data to the alterPayload method of the Java class though the payload variable.
- The println statement logs data to the AWS environment.
- The function alters the payload by setting it to lower-case letters and switching various letters to numbers.
- The altered payload returns to the Lambda framework, which concludes the function call.
AWS SDKs are not required
It’s possible to add extra functionality to your Java Lambda function if you link to the AWS SDK library and reference classes such as the RequestHandler and the Lambda Context. However, this is not necessary. Use of the AWS Java core SDK libraries is certainly recommended for non-trivial functions, but for this simple Java AWS Lambda example it’s not needed.
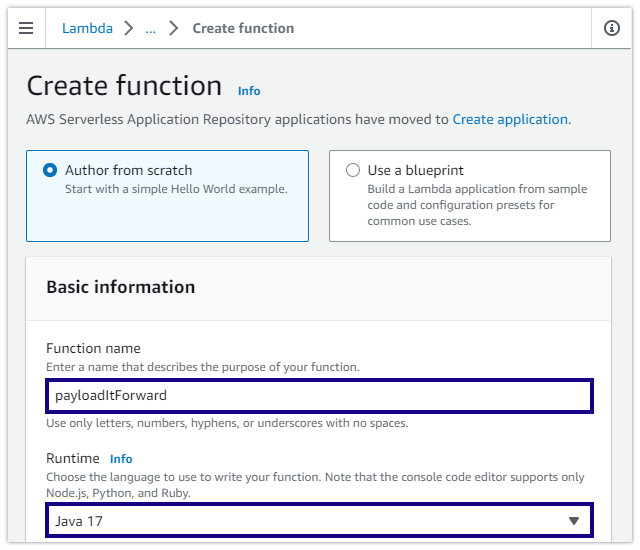
A serverless lambda function is created in AWS to support the Java code.
Java lambda function deployment
To deploy the Java Lambda function on AWS, simply perform the following steps:
- Package the Java class in a JAR file.
- Create the Java Lambda function in AWS.
- Upload the JAR file to AWS Lambda function.
- Point the runtime handler to the uploaded class and method.
- Test the Java-based AWS Lambda function in the management console.
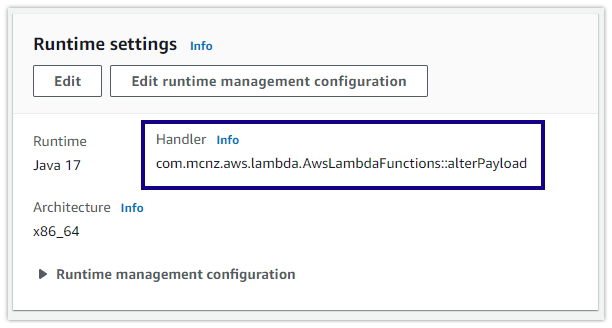
Test the Java code in AWS
When the runtime settings are configured, you can use the AWS Console’s Lambda test tab to invoke the function.
Add the text “Hello World” as event data for the function call, and click Test.
Java Lambda test results from AWS
When the test completes, the output of the Java Lambda function is shown on the screen, and the output from the println statement is displayed in the log output.
If you know what you’re doing, the whole process — create, code, deploy and test — can be done in less than two minutes.
It’s a testament to how simple it is to create an AWS Lambda function in Java.
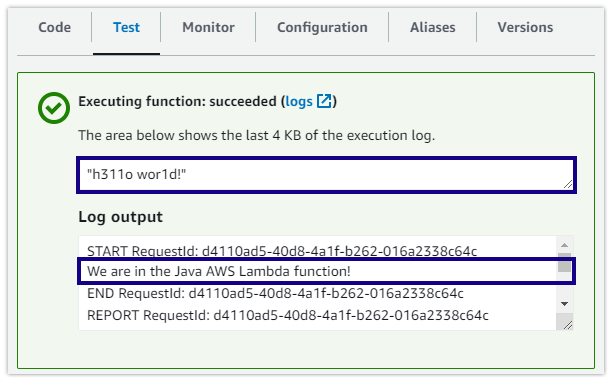
The test tab on the AWS Lambda deployment page makes it easy to test your serverless Java functions.
AWS Java Lambda example on GitHub
You can find the source code for this example on GitHub.