abstract class
What is an abstract class?
An abstract class is a template definition of methods and variables in a specific class, or category of objects. In programming, objects are units of code, and each object is made into a generic class.
Abstract classes are classes that contain one or more abstracted behaviors or methods. Objects or classes can be abstracted, which means that they're summarized into characteristics relevant to the current program's operation. Abstract classes are used in all object-oriented (OOP) languages, including Java, C++, C# and VB.NET.
Abstract classes aren't required in programming, but the concept is designed to keep code cleaner and make programming more efficient, as irrelevant details aren't constantly being referred to. Abstract classes are also useful when creating hierarchies of classes.
Declaring a class as abstract means it can't be directly instantiated, which means an object can't be created from it. This protects code from being used incorrectly. Abstract classes require subclasses to further define attributes necessary for individual instantiation. Subclasses that extend from the abstract class all have that abstract class's attributes, as well as their own attributes that are specific to each subclass.
Classes that are derived from abstract classes use a concept called inheritance, which shares a set of attributes and methods. When done many times, this results in a hierarchy of classes where the abstract class is considered a root or base class.
Abstract classes can be created in the following ways, depending on the language:
- using the abstract keyword in the class definition;
- inheriting from the abstract type; and
- including an abstract method in the class definition.
Abstract classes contrast with concrete classes, which are the default type. A concrete class has no abstracted methods and can be instantiated and used in code. Abstract classes can also be compared to a public class -- which is used for information and methods that need availability in any part of a program's code -- as well as a superclass, which is the class from which subclasses are derived.
What is an abstract method?
In object-oriented programming, methods are programmed procedures included as part of a class. Methods can be included in any object in a class, and any class can have more than one method. In an object, methods only have access to the data known to that object, which ensures data integrity among the set of objects in an application. A method can be reused in multiple objects. Methods also provide an interface that other classes use to modify object properties.
Abstract classes can have abstract and regular methods. Abstract methods have a signature with no implementation body. Abstract methods can only be used in abstract classes and are used to specify when a subclass must implement a method. While abstract methods have no code in the base class, code is instead provided by a subclass.
In derived classes, abstract methods are implemented with the same access modifier, type of argument, number and return type as the base class.
Abstract methods can also be used to specify interfaces for some programming languages.
Abstract classes vs. interfaces
An interface is another similar way to create an abstraction. Like abstract classes, interfaces can't be instantiated. But unlike abstract classes, an interface method can be set as abstract.
Abstract classes can also have final, nonfinal, static and nonstatic variables, whereas an interface has only static and final variables. Additionally, abstract methods can define public, protected and private concrete methods, while interfaces have all fields as automatically public, static and final. Interfaces, however, support multiple inheritances where abstract classes don't.
Implementation is done similarly in Java. For example, using the keyword implements will make a Java interface, while the keyword extends makes an abstract class.
Abstraction vs. encapsulation
Encapsulation in object-oriented programming is the process of wrapping all the important information contained inside an object and only exposing select information. The implementation and state of each object are privately held inside a defined class. Other objects don't have access to this class or the authority to make changes; they're only able to call a list of public functions or methods. This characteristic of data hiding provides greater program security and avoids unintended data corruption, while also making code easier to maintain and reuse.
Encapsulation is done by declaring all the variables in a class as private while writing methods declared as public to set and retrieve variable values.
While abstraction is the process of hiding unwanted data, encapsulation is the process of hiding data with the goal of protecting that information. While abstraction is achieved using abstract classes or interfaces, encapsulation is implemented using an access modifier.
Examples of abstract classes and methods
Abstract classes are created in different ways, depending on the language. For example, abstract classes can be created by using a keyword like abstract, by inheriting the abstract type or from including an abstract method.
The following snippet of code has two classes, FileLogger and DbLogger. FileLogger is responsible for logging data to a file, while DbLogger logs data to a database:
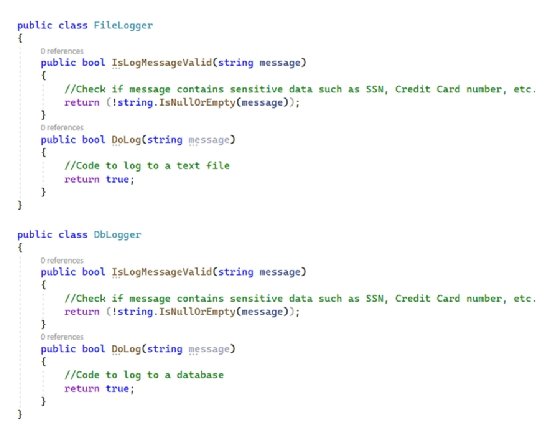
Unfortunately, the code has some redundancies written into it. In this example, programmers would need to write the same logic twice to check for valid log messages -- one for each of the two classes.
The following example creates an abstract class to simplify the code.
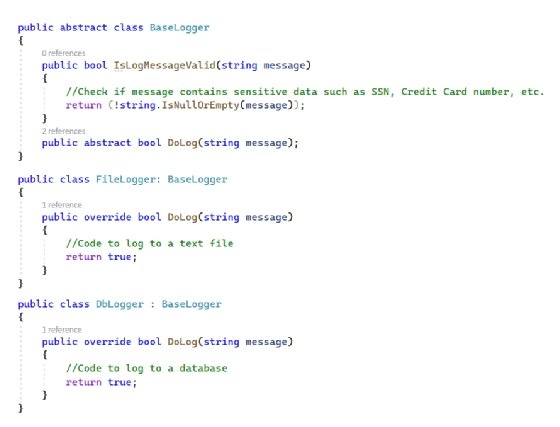
In this example, the IsLogMessageValid method implementation is moved to an abstract class, which helps remove the redundancies.
Learn more about abstraction and how it helps developers avoid possible code mistakes using a concept called refactoring.