What is a bitwise operator?
A bitwise operator is a character that represents an action taken on data at the bit level, as opposed to bytes or larger units of data. More simply put, it is an operator that enables the manipulation of individual bits in a binary pattern.
Bitwise operators vs. other operators
Most operators work with either single or multiple bytes, which in most systems contain eight bits. Examples of such operators include +, - and *. By contrast, bitwise operators can check and manipulate each individual bit within a byte, with each bit carrying a single binary value of either 0 or 1. A single bitwise operator represents the action or operation to be performed on single bits. Examples of bitwise operators include AND (&), OR (|), XOR (^) and NOT (~).
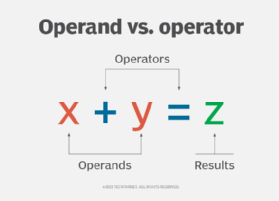
A bitwise operator works with the binary representation of a number rather than that number's value. The operand is treated as a set of bits rather than a single number. The operation then positionally matches individual bits of equal-length bit patterns to return an integer value.
Not all programming languages support the use of bitwise operators; however, C, Java, JavaScript, Python and Visual Basic are among those that do.
There is an order of precedence in bitwise operators. From highest to lowest, the precedence goes as follows:
- Bitwise NOT.
- Left shift and right shift.
- Bitwise AND.
- Bitwise XOR.
- Bitwise OR.
Bitwise operators are similar in many of the languages that support them. For example, the vertical bar symbol (|) represents the bitwise OR operator in C, C++ and JavaScript. Similarly, bitwise AND is a single ampersand (&) in C and C++.
Benefits and uses of bitwise operators
Because they enable greater precision and require fewer resources, bitwise operators can make some code faster and more efficient. Also, the outcome that a bitwise operation produces always lands within the range of possible values for that numeric type, which prevents overflow problems.
Bitwise operators are vital for performing mathematical operations at bit level inside the processor's arithmetic logic unit. Examples of bitwise operation use cases include data encryption, data compression, graphics, communications over ports or sockets, embedded systems programming and finite state machines. Bitwise operators are also used in low-level programming such as device drivers, to convert text case, and to maintain large integer sets for search and optimization.
Bitwise operators common to JavaScript and C++
The most commonly used bitwise operators in JavaScript and C++ are listed in the following table.
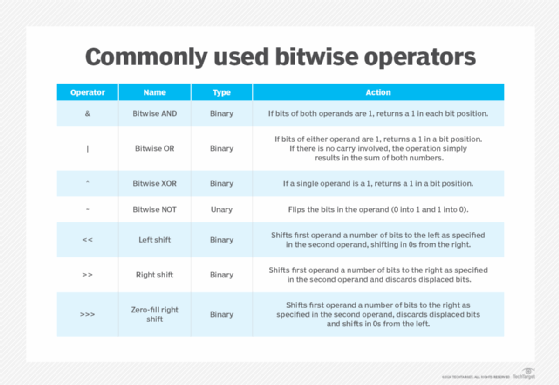
Left shift, right shift and zero-fill right shift are sometimes referred to as bit shift operators. The first two should not be used for negative numbers because it can result in undefined or undesirable behaviors in the program. Undefined behaviors can also result if the number is shifted more than the size of the integer.
Other bitwise operators in C++
Several other bitwise operators are available in C++ in addition to the ones listed above. These include the following:
- Compound operators, which increment or decrement a variable.
- Increment (++).
- Decrement (--).
- Compound arithmetic operators, which perform mathematical operations on a variable with another constant or variable.
- Compound addition (+=).
- Compound subtraction (-=).
- Compound multiplication (*=).
- Compound division (/=).
- Compound bitwise AND (&=), which changes particular bits in a variable to 0 -- also known as resetting bits.
- Compound bitwise OR (|=), which changes particular bits in a variable to 1.
Real-world example of bitwise operators in JavaScript
The following example compares two versions of JavaScript code used to map several form checkboxes to a single number.
var combo = form.elements[0].checked*(Math.pow(2,2))
+ form.elements[1].checked*(Math.pow(2,1))
+ form.elements[2].checked*(Math.pow(2,0));
Bitwise operators can do the same thing, but more efficiently.
var combo = form.elements[0].checked << 2
| form.elements[1].checked << 1
| form.elements[2].checked << 0
C++ is a superset of the C programming language. Learn about the cloud programming languages developers need to know. Explore the differences between scripting vs. programming languages. Also, check out devs discussing when to use TypeScript vs. JavaScript.