Message Driven Beans Tutorial
The following tutorial illustrates how a Message Driven Bean is written and deployed in an Enterprise JavaBeansTM 2.0 Container. The MDB component is invoked by an inbound message from a Java client.
The following tutorial illustrates how a Message Driven Bean is written and deployed in an Enterprise JavaBeansTM 2.0 Container. The MDB component is invoked by an inbound message from a Java client. This function is demonstrated with a sample application run on Pramati Server 3.0 (Alpha). The Server ships with Pramati Message Server 1.0 and can be downloaded from www.pramati.com. The application sources are freely downloadable to get a better understanding of how MDB components are written and work.
What is a Message Driven Bean?
A message driven bean is a stateless, server-side, transaction-aware component that is driven by a Java message (javax.jms.message
). It is invoked by the EJB Container when a message is received from a JMS Queue or Topic. It acts as a simple message listener.
A Java client, an enterprise bean, a Java ServerPagesTM (JSP) component, or a non-J2EE application may send the message. The client sending the message to the destination need not be aware of the MDBs deployed in the EJB Container. However, the message must conform to JMS specifications.
Before MDBs were introduced, JMS described a classical approach to implement asynchronous method invocation. The approach used an external Java program that acted as the listener, and on receiving a message, invoked a session bean method.
However, in this approach the message was received outside the application server and was thus not part of a transaction in the EJB Server. MDB solves this problem.
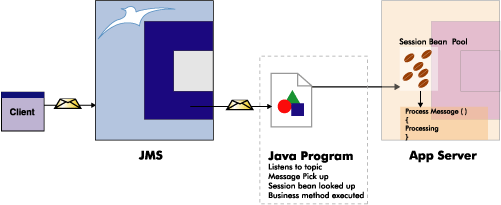
Message processing before (above) and after (below) Message Driven Beans.
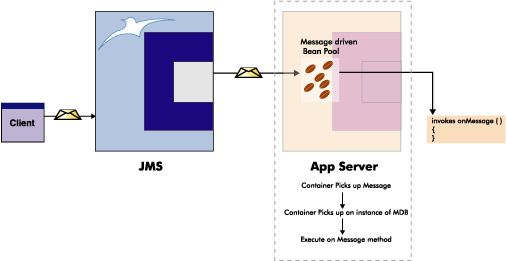
Structure of an MDB
- It has no home or remote interfaces, and is only a bean class.
- It resembles a stateless session bean - that is, it has short-lived instances and does not retain state for a client.
- A client interacts with the MDB in the same way it interacts with a JMS application or JMS server.
- Through the MDB, the EJB 2.0 Container sets itself up as a listener for asynchronous invocation and directly invokes the bean (no interfaces), which then behaves like an enterprise bean.
- All instances of a particular MDB type are equivalent as they are not directly visible to the client and maintain no conversational state. This means that the Container can pool instances to enhance scalability.
Lifecycle of an MDB
The EJB Container performs several tasks at the beginning of the life cycle of the MDB:
- Creates a message consumer (a
QueueReceiver
orTopicSubscriber
) to receive the messages
- Associates the bean with a destination and connection factory at deployment
- Registers the message listener and the message acknowledgement mode
The lifecycle of an MDB depends on the lifespan of the EJB Server in which it is deployed. As MDBs are stateless, bean instances are typically pooled by the EJB Server and retrieved by the Container when a message becomes available on the topic or queue.
Writing an MDB
Writing an MDB involves the following tasks:
- Implement the
javax.ejb.MessageDrivenBean
andjavax.jms.MessageListener
interfaces in the MDB class.
- Provide an implementation of the business logic inside the
onMessage()
.
- Provide a
setMessageDrivenContext()
method that associates the bean with its environment.
- Provide an
ejbCreate()
method that returns void and takes no arguments. This method may be blank.
- Provide an
ejbRemove()
method implementation. This method may be blank, unless certain resources need to be acquired before the bean goes out of scope.
Sample application
The tutorial uses a sample application, StockTrader
, to illustrate the writing of an MDB. The sample uses a simple message driven bean buyAgentMDB
that is contacted by a client which wishes to buy shares. The client looks up the BuyQueue
and implements the javax.jms.MessageListener
. It provides a private method buy()
that takes two arguments: a double value that holds the price and a string (stockSymbol
) that holds the scrip symbol.
|
|||||||||||||||||||||||||
Defining the MDB ClassAn MDB implements two interfaces: javax.jms.MessageListener and javax.ejb.MessageDrivenBean. The MDB class is defined as public and cannot be defined as abstract or final. The MDB class looks like this: public class BuyAgentMDB implements MessageDrivenBean, MessageListener { private MessageDrivenContext mdbContext; The class must consist of:
The class must not contain the Implementing business logicBusiness logic for the EJB is triggered by the The signature for the method is: public void onMessage(Message msg) {} In the sample, when there is a message on the The following code of the public void onMessage(Message msg) { // Retrieves the text message TextMessage priceMessage = (TextMessage) msg; try { } catch(JMSException ex) { ex.printStackTrace(); } } Implementing the ejbCreate methodThe The following code of the BuyAgentMDB performs this function: public void ejbCreate () throws CreateException { } Providing methods for transactional managementThe setMessageDrivenContext provides the methods for transaction management. The BuyAgentMDB implements setMessageDrivenContext. This method is called by the EJB Container to associate the BuyAgent instance. The context is maintained by the Container. The input parameter for setMessageDrivenContext is an instance of the MessageDrivenContext interface. It gives the MDB access to information about the runtime environment. In Container-managed transactions, de-queuing occurs out of the onMessage(). The only methods on the MessageDrivenContext that are accessible to the MDB are transaction-related methods. Removing the instanceThis method is invoked when an MDB is being removed from a pool. Application server vendors may implement an arbitrary algorithm that decides when to remove the MDB instances from the pool. The following code of the BuyAgentMDB performs this function: public void ejbRemove() { //Removes the bean from the pool } Acknowledging messagesFor MDBs that use container-managed transactions, the Container automatically acknowledges a message when the EJB transaction commits. The Container overrides the acknowledgement mode given in the deployment descriptor. If the EJB uses bean-managed transactions, both the receipt and the acknowledgement of a message occur outside the EJB transaction context. Setting up the responseSome business cases may require responding to the Sender of the message that triggered the MDB. To register the "reply to" destination of the Sender, the client can use getJMSReplyTo header. The JMSReplyTo header field contains a destination supplied by the client along with the message. It is the destination where a "reply to the message" may be sent. Messages sent with a JMSReply value typically expect a response. The code to use the JMSReplyTo header looks like this: public void onMessage(Message msg){ try{ destination d = msg.getJMSReplyTo() } catch(JMSException jmse){} } Deploying the MDBThe destination associated with an MDB is specified in the deployment descriptor of the MDB in the ejb-jar.xml file. A destination is a JMS-administered object accessible via JNDI. Here is a sample destination entry in the deployment descriptor: <message-driven-destination> <destination-type>javax.jms.Topic</destination-type> <subscription-durability>NonDurable</subscription-durability> </message-driven-destination> Elements in the deployment descriptorThe description of a MDB in the EJB 2.0 deployment descriptor contains the following specific elements:
The MDB deployment descriptor specifies whether the bean is intended for a Topic or a Queue. A bean set for a Topic can act as a durable subscriber guaranteeing that the listener receives all messages even if the listener is unavailable for some time. The deployment descriptor also sets transaction demarcation and security. If the transaction type is "container", the transactional behavior of MDB methods is defined as for other enterprise beans in the deployment descriptor. Here is the entry for the transaction type in the deployment descriptor: <transaction-type>Container</transaction-type> <acknowledge-mode>Auto-acknowledge</acknowledge-mode> For the onMessage method, only the Required or NotSupported transaction attribute must be used as there are no incoming transaction context. The important thing to notice is that the deployment descriptor contains all the information except the destination name required to deploy a message-driven bean. The destination name is set in an application server's vendor-specific configuration file or as a system property (deploy time mapping). Running the sampleConfiguring the Message ServerBy default, the following destinations are entered in the configuration file of the Message Server:
The configuration file is located in the directory Deployment stepsThe following steps will deploy the application on Pramati Server:
Deploying the applicationWhen the Server starts, click on the Select [install_dir]/samples/mdb/where [install_dir] is the installation directory of Pramati Server 3.0. Running the clientSet the classpath as follows: Windows Starting the stock repositoryThe stock repository is run by the client java com.pramati.samples.mdb.StockServer This brings up the stock repository panel which displays the scrip and the price along with a Buy/Sell advisory message. Observe the price fluctuates every time buying or selling happens. SummaryMDBs overcome the limitations of synchronous messaging using session and entity beans in the EJB 1.1 container. MDBs can be deployed in the EJB 2.0 Container, which acts as a message listener and uses inbound messages as a trigger to invoke business methods in an asynchronous manner. This tutorial outlined the steps involved in writing an MDB using a sample application that may be downloaded from www.pramati.com. For further information, contact [email protected]. |