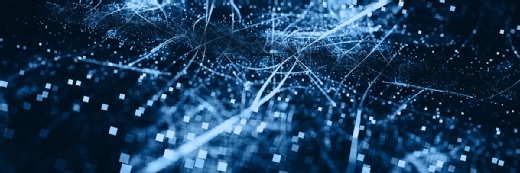
Getty Images
Tips and tricks for TypeScript programming
For those new to TypeScript or for Java developers transitioning into JavaScript, these three TypeScript tips and tricks modern day software developers will come in handy.
TypeScript is growing in popularity as the go-to programming language for many web applications. It enables developers familiar with JavaScript to program according to the principles and practices intrinsic to the object-oriented programming paradigm.
TypeScript is a strong-type language that supports all the features typically found in object-oriented programming languages, such as Java and C#. For example, JavaScript has no support for interface, but you can program to an interface in TypeScript.
Programmers create code in TypeScript, either in an IDE or standalone, and then a TypeScript compiler transforms the TypeScript code into JavaScript. The JavaScript is then deployed for use in browsers and for server-side implementation under Node.js.
The main benefit of using TypeScript is that it helps prevent developers from shipping buggy code. Part of the TypeScript compilation process identifies design-time errors before the code is translated into JavaScript. TypeScript also lets developers take advantage of code practices inherent in a strongly typed language.
Here are three TypeScript tips and tricks that a developer can use to take advantage of the features available in the TypeScript programming language:
- Try an enum or custom type to constrain a value on a string variable.
- Go beyond typeof, and use instanceof.
- Use for(;;) instead of while to infinitely execute a loop.
Let's look at the details of each.
1. Try an enum or custom type to constrain string values
Ensuring that a string variable is assigned proper value can be a laborious task for developers. For example, assigning the value asparagus to the variable animal: string is not only inaccurate, but it might also cause runtime errors depending on the logic of the given program in which the variable is declared.
One way to address the issue in TypeScript is to define an enum of a string, like so:
export enum Fruit {
Apple = 'Apple',
Orange = 'Orange',
Cherry = 'Cherry',
}
Then, the TypeScript compiler throws an error when you to try to run this code:
let goodFruit: Fruit = 'Asparagus';
Another way to constrain the value assigned to a string variable is to create custom types, like so:
export type Fruit = 'Apple' | 'Orange' | 'Cherry';
export type Vegetable = 'Lettuce' | 'Carrot' | 'Radish' | 'Potato'
In that code snip, Apple is a custom type that represents the value Apple or Orange or Cherry, and Vegetable is a custom type that represents the value Lettuce or Carrot or Radish or Potato.
Thus, the following code passes through the TypeScript compiler:
const goodFruit: Fruit = 'Apple';
However, this code does not pass:
const goodFruit: Fruit = 'Lettuce';
Instead, that code generates a compiler error similar to the following:
mycode.ts(13,11): error TS2322: Type 'Vegetable' is not assignable to type 'Fruit'.
Type 'Lettuce' is not assignable to type 'Fruit'.
Defining a custom type to constrain the values assignable to variables of that type is a neat and easy-to-use shorthand trick.
2. Go beyond typeof and use instanceof
The typeof operator is used to determine the type of a variable. You can use typeof in situations when you want to ensure that a variable is a number, for example.
The typeof operator has been part of JavaScript since the beginning. Thus, it is also available in TypeScript because TypeScript is built "on top of" JavaScript.
However, typeof has limitations. For one, it only checks for the basic JavaScript types: Undefined, Null, Boolean, Number, BigInt, String, Symbol and Function. Also, the return value of typeof is a string, as demonstrated in the following example:
const amount = 1.29;
console.log(typeof amount)
The response is: "Number."
The typeof operator also has limitations when used in TypeScript, particularly when applied to a custom class created in TypeScript. Take a look at the following example:
// Create a variable based on the custom class, Bird
const bird = new Bird();
// Use typeof to test of the type of the variable
const result = typeof bird;
// Print the result of typeof using console.log()
console.log(`Here is the result of running typeof bird: ${result}`);
The output of console.log() is the following:
Here is the result of running typeof bird: object
Notice that typeof can only report one of the basic JavaScript types, which, in the case of the bird variable, is object. The typeof operator has no understanding of the custom class Bird created in TypeScript.
However, the TypeScript instanceof operator does understand.
The following example demonstrates how to apply the TypeScript instanceof operator to the variable bird declared above. The statement bird instanceof Bird is executed within a console.log() statement:
console.log(`Is bird an instanceof Bird? ${bird instanceof Bird}`);
The following is the result of the console.log() output:
Is bird an instanceof Bird? true
Notice that the instanceof operator reports that the variable bird is indeed an instance of the class Bird.
The following example demonstrates a negative response from instanceof:
console.log(`Is bird an instanceof Dog? ${bird instanceof Dog}`);
The result printed from console.log() is the following:
Is bird an instanceof Dog? False
The result makes sense because the variable bird is not an instance of the class Dog.
Using the instanceof operator only returns True or False. Executing instanceof like so won't work:
instanceof bird
Instead, you must declare the class against which to test the variable of interest.
Despite this shortcoming, the instanceof operator is a powerful tool to work with a custom class you created in TypeScript. The instanceof operator provides a finer grain of inspection than the JavaScript typeof operator.
3. Use for(;;) instead of while to infinitely execute a loop
This final tip isn't specific to TypeScript, but it is an alternative to using a while loop to execute code continuously.
One technique to run code continuously is to create a while loop, like so:
let x = 0;
while (true) {
console.log(`This is loop ${x}`);
x++;
}
That code is a perfectly good way to run an infinite loop. Still, there is an alternative way, like so:
let x = 0;
for (;;) {
console.log(`This is loop ${x}`);
x++;
}
The example above uses a for loop that has no conditions set in its declaration. It's not better or worse than using a for loop. It's just a different way.
Developers accustomed to JavaScript can apply advanced principles and practices of object-oriented programming to code in TypeScript. As a result, they can access more of the features found in object-oriented programming languages, such as Java and C#.
The tips described in this article demonstrate just a few of the many things you can do in TypeScript to make your programming efforts more effective, efficient and fun. There's always more to learn, but hopefully, you find these tips useful immediately.