How to write your first Java program
How to learn Java quickly
The easiest way to learn Java is to use an online development environment such as Replit or Onecompiler and quickly write your first Java program. Here’s why:
- You don’t need to install the JDK.
- You don’t need to install an IDE.
- You don’t need to compile code.
All you need to do is open an online editor and code away! Which is exactly what we’re going to do here.
How to write your first Java program
The first non-trivial Java program I ever wrote was a number guessing game, and it gave me a good idea of how variables, loops and conditional statements work. I want to show you how to do the same. The steps we’ll follow as you write your first Java program are as follows:
- Navigate to the Onecompiler website in your favorite web browser.
- Switch to Onecompiler’s JShell editor.
- Run the obligatory Java Hello World! app.
- Add two variables to represent user input and a magic number.
- Implement conditional logic to see if the two variables match.
- Garner user input to make our first Java program more dynamic.
- Add a loop so the game continues until the user guesses the magic number.
Run your Hello World! program in Onecompiler
Once you navigate to Onecompiler and select the JShell editor, you’ll find a program that runs the seminal Hello World! app is already coded for you.
Click the big red Run button to run your first Java program. The words Hello World! appear in the output window.
We’re not done yet. My promise was to help you create your first Java program that wasn’t trivial. Hello World! is as trivial as it gets.
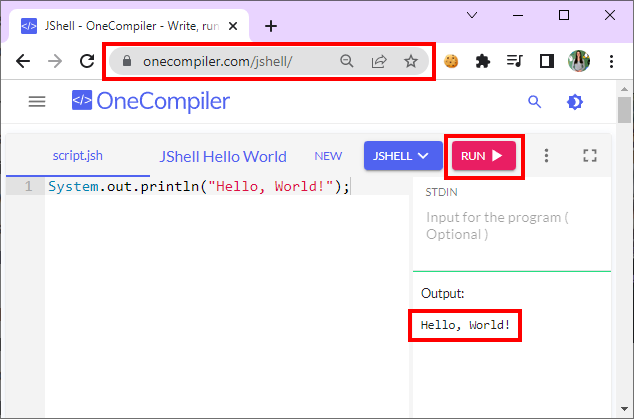
Hello World! should be everyone’s first Java program.
Add variables to your first Java program
Our application requires two variables:
- One variable to represent the magic number to be guessed.
- The user’s attempt to guess the magic number.
We’ll also want to prompt the user to guess the magic number.
If we hardcode values for the magic number, our code looks like this:
var theMagicNumber = 7; var theGuess = 5; System.out.println("Guess the number!");
There are two things to keep in mind at this point in time:
- Java is case-sensitive.
- Statements end in semicolons.
If you violate either of those two Java programming rules, your first Java program won’t compile.
Add some conditional if statements
We want to check if the user’s guess is too low, too high, or exact, so add the following conditional logic to your application:
if (theGuess < theMagicNumber) System.out.println(theGuess + " is too low!"); if (theGuess > theMagicNumber) System.out.println(theGuess + " is too high!"); if (theGuess == theMagicNumber) System.out.println(theGuess + " is too high!");
Run the program with different values for theGuess and watch different results display in the output window.
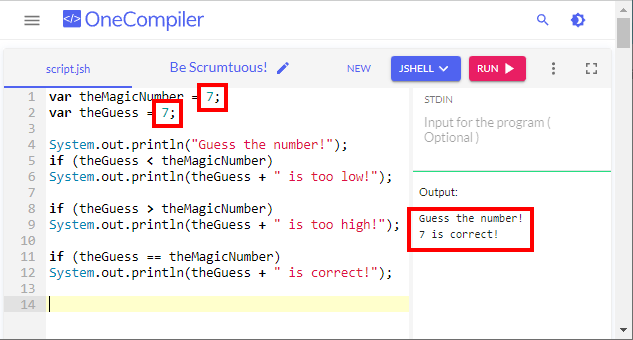
Conditional logic in Java is done with if…else statements.
Iterative logic in our first Java program
We would like to keep asking the user to guess the magic number until they get it right.
That means we must enclose our conditional logic within the scope of a while loop.
Anything within the curly braces {} that follow a while statement will run until the condition of the while loop is met.
Here’s how our while loop works:
- The while loop keeps running if the user’s guess is not equal to the magic number.
- When the user’s guess is equal to the magic number, the loop exits.
The code for the while loop is as follows:
while(theGuess != theMagicNumber) { /* our conditional logic goes here */ }
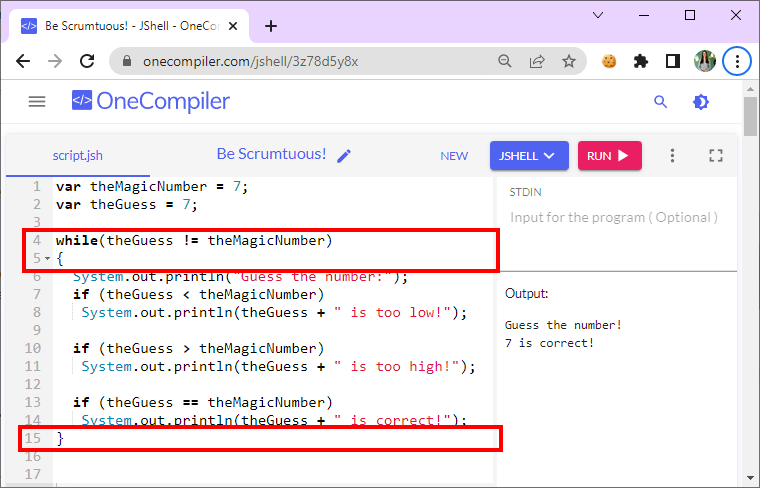
A while loop allows our first Java program to perform iterative logic.
Add user input to the Java program
To take input from the user and make our program interactive, we must do three things:
- Add a //–execution local statement to the top of our file.
- Create a new instance of a class named Scanner.
- Assign theGuess variable to input from the user.
If this was a normal Java application run locally, we wouldn’t need to add the //–execution local statement at the top of the file. Nevertheless, it’s a small price to pay to run Java code online.
The statement to create the Scanner can go at the top of the file
Scanner input = new Scanner(System.in);
With this code added, we can set the value of theGuess by calling the nextInt() method of the Scanner class:
theGuess = input.nextInt();
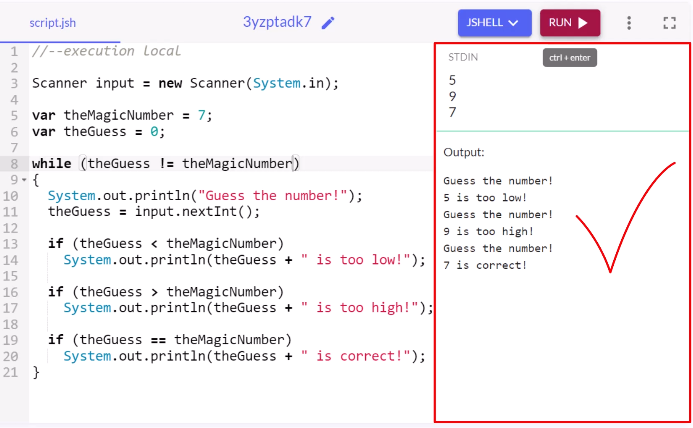
Provide values for input and run your first program.
Provide input values and run the program
Before you run the JShell program, enter some values under STDIN to be used as input.
I added the values 5, 9 and 7 as input.
Make sure the last number you provide as input matches your magic number, or else your program will loop forever.
Run your first Java program!
When you run your first Java program, you should see output that proves your variables, conditional logic and iterative statements all work.
Along with the prompts to input data, the conditional logic of my program generated the following output with the values of 5,9 and 7:
- 5 is too low!
- 9 is too high!
- 7 is correct!
That’s it! That’s how easy it is to program in Java!
What’s next?
There are a number of improvements we could make to this game, including the following:
- Randomize the magic number.
- Take input from Windows dialog boxes.
- Use if..else statements in the logic.
We will leave all of those for the next installment of how to learn Java. For now, if you followed along, pat yourself on the back for a job well done, and revel in the fact that you successfully coded your very first Java program.