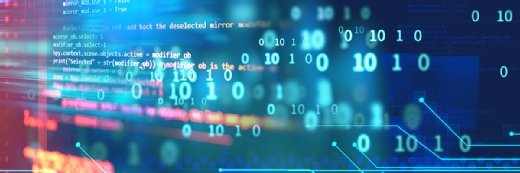
Getty Images/iStockphoto
11 lessons learned from writing my first Java program
You'll be amazed how easy it is to learn Java and write powerful cross-platform applications when writing your first Java program. Some misconceptions about Java just aren't true.
They are many myths and misconceptions surrounding Java in the IT world. These myths are quickly dispelled if you take the time to actually write some Java code.
What my first Java program taught me
Here are the 11 things I learned when I wrote my first Java application:
- You don't need to install the JDK or an IDE.
- Java isn't verbose.
- Java is expressive.
- Whitespace doesn't matter.
- Java supports read-evaluate-print-loop (REPL) capabilities.
- Java is case sensitive.
- Semicolons are required.
- Text goes inside double quotes.
- Java uses dot notation.
- Round brackets are called parenthesis.
- Java is easy to learn!
1. You don't need to install the JDK or an IDE
The tech community told me that to write a Java application, I would need to do the following:
- Choose a version of the JDK to install.
- Choose from one of many distributions.
- Download the JDK and install Java.
- Download and install an IDE like Eclipse.
- Write my first Java application.
I didn't need to do any of that. I simply went to a website that provided an online compiler, and then I wrote some Java code. You don't need to download the JDK or an IDE to start on your Java learning journey.
2. Java is not verbose
The community told me that Java is needlessly verbose. Even a simple Hello World program, I heard, requires a class declaration, a main method and a bunch of matching braces and parentheses.
That may have been true 20 years ago when you had to build a full-blown, desktop application to run a Java program. However, that is not the case today.
I only needed one line of code to write a Hello World app in Java. This hardly seems verbose to me.
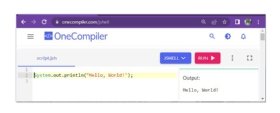
As applications increase in size and complexity, this Java verbosity myth becomes more apparent and even more busted.
3. Java is expressive
Even though Java has more built-in features than languages like Python or JavaScript, these features are easy to identify and access because Java is so expressive.
Even just for a simple Hello World application, a developer has the option to print text to the output log files, to the console screen or even to the Windows dialog box.
Java's expressiveness makes it easy to differentiate between those three options:
- out.print("Hello World"); //print to the System output logs
- console.print("Hello World"); //print to the console screen
- showInputDialog("Hello World"); //Display in a dialog box
Java's extensive feature set can seem overwhelming. But Java's expressiveness lets developers access features and functions in a straightforward manner.

4. Whitespace doesn't matter
YAML and Python are sticklers for whitespace. With YAML, if you use three spaces instead of two or two tabs instead of two spaces, your Docker build files won't run. Python has similar rules.
Java doesn't care about whitespace. Java developers are free to use tabs, spaces and newlines at will. If you don't like the way a fellow developer formats their code, you can use a linter to format it to your own standards.
Java grants developers a degree of freedom that other languages simply don't provide to their users.

5. Java supports REPL
Want to write program that performs a REPL cycle? Java can do that.
The Java 9 release introduced JShell, a REPL-based environment that lets Java developers write scripts. REPL programs require fewer lines of code than a Java-based desktop application with a main method. It's the right environment to use when you write your first Java program.
6. Java is case sensitive
Some programming languages, such as BASIC and SQL, are not case-sensitive. Java is. If you get the case wrong on a method, property or class, your Java code won't compile.
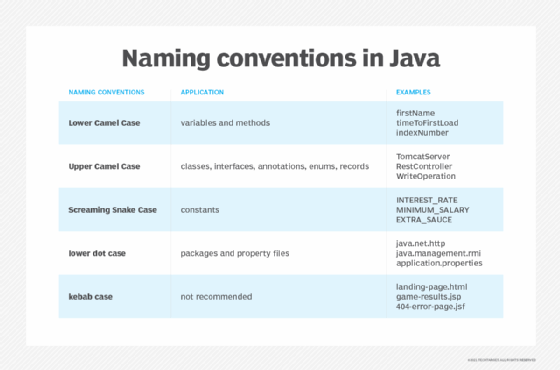
7. Semicolons are required
Programming languages including Python and JavaScript don't require semicolons, but Java does. Every Java statement ends in a semicolon. Structure and flow control elements don't end in a semicolon.
The only exception is JShell, where semicolons are optional.
8. Text goes inside double quotes
In HTML and JavaScript, single quotes are the norm. Not in Java. In Java, text Strings go inside double quotes. If you put text Strings inside single quotes, your code will not compile.
9. Java uses dot notation
To chain objects, properties and methods together, Java uses dot notation. Most other programming languages do that as well. Anyone familiar with JavaScript, C++ or Python will quickly adjust to Java's object-oriented syntax.
10. Round brackets are called parenthesis
Java uses a mixture of round brackets, square brackets and curly brackets. However, round and curly brackets aren't actually called brackets:
- Round brackets ( ) are parentheses.
- Square brackets [ ] are brackets.
- Curly brackets { } are braces.
11. Java is easy to learn!
Perhaps the most important thing I learned when I wrote my first Java program is that Java is fun and easy to learn.
Java is simple, expressive, object oriented and well documented. If I can learn it, you can learn it too!