Hello World in Python vs Java
Java and Python Hello World apps compared
A simple application that prints nothing more than the words Hello World is the seminal start to learn any programming language.
Furthermore, the relative verbosity and expressiveness with which a given language prints these eleven Unicode characters has become a popular flex between platform advocates.
Python and Java developers often go toe-to-toe on the Hello World debate, which is why a thorough comparison of how to write Hello World in the two languages is necessary.
Java’s Hello World
To print Hello World in Java’s JShell, it’s one line of code:
System.out.print("Hello World")
This one line of code prints Hello World to the system’s default output stream, which Java’s expressiveness makes fairly obvious.
If you wanted to print to just the console window and not the default output stream, the code would be this:
System.console().writer().print("Hello World")
Windows components in Java
A Java developer could even print Hello World to a Linux or Windows dialog box with a single line of Java code:
javax.swing.JOptionPane.showInputDialog("Hello World")
In fairness, this option should be broken into two statements:
- An import for the Swing package.
- The call to display the input dialog box.
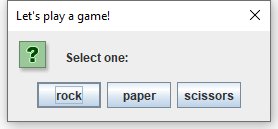
A single line of code enables you to create complex Windows components in Java.
Nevertheless, it’s certainly impressive to see how easy it is to create windowing components with a default installation of the JDK.
I was told it would take a hundred lines of code to write 'Hello World' in Java.🤔
It didn't. 🤷♀️
It took one.🤦♀️ pic.twitter.com/sSIzq6zmwg— Darcy DeClute || DevOps || Git || Scrum || Python (@scrumtuous) April 25, 2023
Hello World in Python
In the world of software development, Python developers can’t wait to flex how easy it is to print Hello World in the popular scripting language.
Here’s how most Python tutorials demonstrate a Hello World:
print("Hello World")
It’s impressively terse, and contrasts directly with the expressive nature of Java. It may actually be too terse.
Python’s lack of expressiveness
In the prior Java examples, it was expressively clear where the output would be displayed. With Python, it’s not clear where Hello World is being printed to.
- Is it printed to the log files?
- Is it printed to standard output?
- Is it printed to a terminal window?
- Is it printed to a printer?
- Will it display in a Windows dialog box?
Python definitely lacks the expressiveness of Java, but there’s a much more serious problem with this code.
Do requirements matter?
In fact, this Python code doesn’t print out Hello World. It actually prints out Hello World\n.
The clearly stated requirement was to print out eleven Unicode characters. The Python code prints out 12, as the print method insists on always adding \n to every print statement, regardless of whether or not it’s part of the requirements. (At runtime, the \n combination prints as a single newline character in the output, which is why 12 characters are printed, not 13.)
Freedom vs control
This is actually an interesting contrast between Python and Java.
New developers love Python because it often presupposes what a developer needs and makes arguably intelligent decisions for the programmer.
In contrast, Java provides developers with a pure, unadulterated set of powerful APIs and trusts the developer to make their own intelligent and informed decisions about how to use them. There’s no hand-holding in Java.
Who decides what matters?
Many Python developers argue that the result is close enough, and that the specific requirements matter less than successfully accomplishing a task.
The it doesn’t matter refrain is a common mantra amongst Python developers:
- Why is Python 300-500% slower than Java? Performance doesn’t matter.
- Why does Python consume 300% more energy than Java? The environment doesn’t matter.
- Why did it take almost 30 years to get a switch statement in Python? Language features don’t matter.. until they do.
- Why can’t Python thread across multiple cores? Multithreading doesn’t matter.
It may be true that individual requirements such as performance and language features, and even global warming, might matter less in the broader scope of successful real-world project completion.
However, requirements do matter. And a developer’s first program certainly isn’t the right time to be teaching aspiring young programmers that it’s ok to brush them aside.
Hands on with the Python plumbing
In fact, there are actually multiple ways to write Python code that meets requirements despite the code’s shortcomings listed above.
The easiest fix is to simply call the standard Python APIs, which requires two lines of code:
import sys
sys.stdout.write("Hello World")
Unlike Java, Python does not automatically import the system object, which results in twice as many lines of code to accomplish what Java achieved earlier in just one.
Python’s built-in functions
The other way to fix the problem is to mess around with Python’s print method, which means learning about Python’s collection of built-in functions.
One of the most fundamental concepts in object-oriented programming is SOLID’s single-responsibility principle which says that a component should have one, single, clearly defined purpose.
However, to avoid the extra lines of code produced through interaction with the standard Python APIs, Python instead creates a single, top-level component that provides a dog’s breakfast worth of cryptic and unrelated functions including:
- __import__()
- abs()
- globas()
- str()
- repr()
- chr()
- dict()
These methods do come in handy, are easy to use and can help new developers become quickly proficient with the Python language.
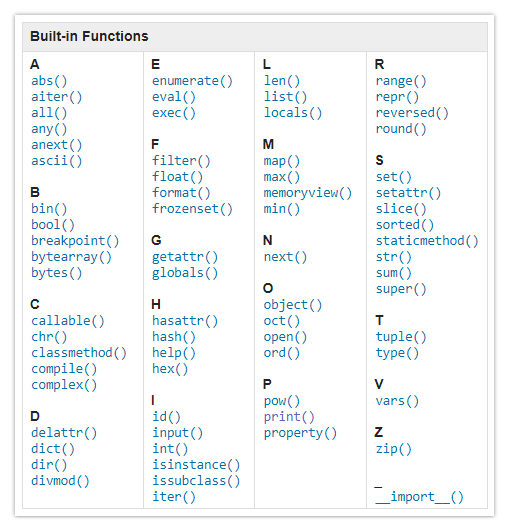
Python provides a variety of built-in functions to hide the complexity of the underlying API.
Playing with Python’s porcelain
When you dig into the Python porcelain, you see that the print method is defined as such:
print(*objects, sep=' ', end='\n', file=None, flush=False)
You can see from this definition that a newline character automatically appends to whatever is printed out. So, according to the documentation, to use Python’s print method without adding the newline is coded like this:
print("Hello World", sep=' ', end='', file=None, flush=False)
Variable arguments in Python
A powerful Python feature that helps curb verbosity is the ability to vary the number of arguments passed to a function. The above method call can be trimmed down to the following line of code:
print("Hello World", end='')
The final result is that Hello World, without the newline escape sequence, prints out to Python’s standard output stream.
Python GUI components
Earlier I demonstrated that Java generates a Hello World dialog box in a single line of code.
Python can similarly generate dialog boxes with the tkinter module, although the code required is significantly more verbose:
import tkinter as tk from tkinter import messagebox messagebox.showinfo("Hello World")
Python vs. Java compared
The simplicity, expressiveness and ease with which a programming language prints out eleven simple characters is a common comparison made to evaluate a platform’s user-friendliness.
This tutorial provides a side-by-side comparison of how to write a Hello World application in both Java and Python.
Both languages approach the task in a unique manner, which is why the programming world is so vibrant. Developers can find the language whose uniqueness works best, for themselves and the problem domain they are in.
It empowers programmers to develop solutions in an environment that makes them the most productive and engaged, which is the desired end result for all software development teams.