User input with a Java JOptionPane example
Java JOptionPane input example
The best way to teach the concept of Java user input to new software developers is to show them how to use the highly visual and user-friendly JOptionPane class from the Swing package.
With one simple line of code, Java’s JOptionPane enables a program to prompt the user with a Windows-based input dialog box, and return any user input as a String.
var name = javax.swing.JOptionPane.showInputDialog("What is your name?");
If the javax.swing package is imported, the JOptionPane code becomes even more succinct. The import also simplifies the use of other fun JOptionPane methods including:
- showConfirmDialog();
- showMessageDialog(); and
- showOptionDialog().
JOptionPane import and methods
For example, the following Java class uses JOptionPane’s showInputDialog()
method to prompt for user input, and then displays a message back to the user with the showMessageDialog()
function. As you can see, the javax.swing
import greatly reduces the code’s verbosity:
import javax.swing.*;
public class JOptionPaneExample {
public static void main(String[] args) {
/* JOptionPane Java user input example */
var name = JOptionPane.showInputDialog("What is your name?");
var output = name + " is such a nice name!";
JOptionPane.showMessageDialog(null, output);
}
}
JOptionPane vs Console vs Scanner input in Java
It has always been a mystery to me: Why do instructors teach Java always subject students to console-based input with the JDK’s Scanner, Console or InputStream classes?
Using a command prompt or terminal window to input text into a computer program is not intuitive, nor is it fun. Actually, console input is a great way to discourage new learners and push them away from the Java platform.
In contrast to the Console or Scanner classes, the JOptionPane provides immediate visual feedback, which makes writing Java code satisfying and fun. Furthermore, it teaches newcomers to the Java platform some important lessons about the JDK, including:
- Java user input does not have to be terminal- or console-based.
- Java supports Windowing components out of the box, unlike languages such as Python or Rust.
- Java is cross-platform, as the JOptionPane works on Linux, Windows and Macs.
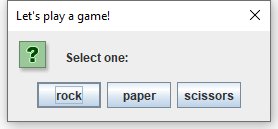
The Java JOptionPane showOptionDialog can take custom input from the user.
JOptionPane showConfirmDialog example
The showInputDialog and showMessageDialog functions are great at garnering user input and displaying a response. Alternatively, to limit a user’s response to just yes, no or cancel, you can use the JOptionPane’s showConfirmDialog() method.
This function displays a message box with only three options, and returns one of the following int values to the program:
- 0 if the user selects ‘Yes’
- 1 if the user selects ‘No’
- 2 if the user selects ‘Cancel’
The following example demonstrates the JOptionPane’s showConfirmDialog()
method in action:
import javax.swing.*; public class JOptionPaneExample { public static void main(String[] args) { /* JOptionPane Java user input example */ var yesOrNo = JOptionPane.showConfirmDialog(null, "What will it be?"); if (yesOrNo == 0) { JOptionPane.showMessageDialog(null, "You chose yes!"); } if (yesOrNo == 1) { JOptionPane.showMessageDialog(null, "You chose no."); } if (yesOrNo == 2) { JOptionPane.showMessageDialog(null, "You chose to cancel!"); } } }
JOptionPane showOptionDialog method
To limit input, but not restrict the user to a ‘yes’, ‘no’ or ‘cancel’, the JOptionPane showOptionDialog method can be used.
This method allows you to supply an array of objects to the dialog box. Each object is rendered, and the calling program receives the array index position of the option selected.
For example, the following JOptionPane showOptionDialog example asks the user to select a brownie, pie or cake as a dessert option.
import javax.swing.*; public class ShowOptionDialogExample { public static void main(String[] args) { /* JOptionPane Java user input example */ String[] options = { "brownie", "pie", "cake" }; var dessert = JOptionPane.showOptionDialog(null, "Which dessert?", "Select one:", 0, 3, null, options, options[0]); if (dessert == 0) { JOptionPane.showMessageDialog(null, "You chose a brownie!"); } if (dessert == 1) { JOptionPane.showMessageDialog(null, "You chose pie."); } if (dessert == 2) { JOptionPane.showMessageDialog(null, "You chose cake!"); } } }
If the user selects the pie button, then the code returns an index of 1, which generates a JOptionPane message dialog box that displays the text, “You chose pie!”
JOptionPane error message dialog boxes
As you can see, the JOptionPane’s showOptionDialog box is highly parameterized. The options available are:
- The parentComponent, which can be left null unless the program will display a dialog box on a custom panel
- The title of the dialog box
- The text to display inside the dialog box
- An option type to include yes, no or cancel buttons
- The message type, be it warning, informational or a question
- An optional custom icon to display
- The array of values to display
- The item in the array to initially highlight
How to use Java’s JOptionPane for user input
New users to the Java language should be encouraged to use the JOptionPane for user input. To use a JOptionPane, simply follow these steps:
- Import javax.swing.*;
- Choose the type of JOptionPane dialog box to use
- Parameterize the showXyxDialog method appropraitely
- Store any data returned from the JOptionPane in a variable
It’s fun to do windowing programming with Java.
Java’s Swing package has a variety of powerful classes with which to create desktop applications that run well on all platforms. And the JOptionPane is a great way to introduce yourself to the world of Swing based programming in Java.