Java's JOptionPane showOptionDialog by Example
How to use the JOptionPane’s showOptionDialog method
Java’s JOptionPane provides a simple way to read input from the user and display information back.
The JOptionPane’s list of methods includes many helpful functions, such as the following:
- showInputDialog
- showMessageDialog
- showConfirmDialog
- showOptionDialog
Each of these functions is relatively straightforward, with the exception of the JOptionPane’s showOptionDialog method.
JOptionPane showOptionDialog examples
To help demystify the most complicated of these, let’s look at a few different JOptionPane showOptionDialog examples.
The JOptionPane’s showOptionDialog method requires an array of text Strings. Each element in the array is displayed as a clickable button. When a button is clicked, the windows closes and the array index of the element selected is returned to the program.
Below is a simple showOptionDialog example, followed by an image of the dialog box this code generates.
import javax.swing.*; public class ShowOptionDialogExample { public static void main(String[] args) { /* Simple JOptionPane ShowOptionDialogJava example */ String[] options = { "rock", "paper", "scissors" }; var selection = JOptionPane.showOptionDialog(null, "Select one:", "Let's play a game!", 0, 3, null, options, options[0]); if (selection == 0) { JOptionPane.showMessageDialog(null, "You chose rock!"); } if (selection == 1) { JOptionPane.showMessageDialog(null, "You chose paper."); } if (selection == 2) { JOptionPane.showMessageDialog(null, "You chose scissors!"); } } }
The Swing JOptionPane showOptionDialog can take custom input from the user.
Java’s showOptionDialog method explained
These are the two most important lines of code from the showOptionDialog example above:
String[] options = { "rock", "paper", "scissors" }; var selection = JOptionPane.showOptionDialog(null, "Select one:", "Let's play a game!", 0, 3, null, options, options[0]);
The array named options
defines the three fields to display in the dialog box.
The array is then passed as the seventh of eight parameters required by the JOptionPane’s showOptionDialog method.
When the dialog box appears, the names of the elements in the array appear as clickable buttons.
The array index of the button that the user clicks is returned to the program.
The showOptionDialog‘s many arguments
The fact that the showOptionDialog function takes eight parameters can make it both intimidating to use and difficult to understand.
Here are the eight fields the showOptionDialog method defines, along with how you can use them in your application:
- The first parameter is the parentComponent. You can set this to null if the dialog box will be rendered independently.
- The second parameter is the prompt that will display inside the dialog box.
- The third parameter is the text to be displayed in the title of the dialog box.
- The fourth parameter is the optionType. This will be the number 0,1,2, or 3, to specify whether yes, no or cancel buttons appear.
- The fifth parameter is the messageType. Similarly, this is the number 0,1 or 2 or 3 to specify whether an error, info, warning, question or default message icon will appear.
- The sixth parameter is an optional image icon to display in the message box.
- The seventh parameter is the array of options to use.
- The eighth parameter is the array object to select as the default value.
Simple showOptionDialog defaults
To simplify the use of the JOptionPane’s showOptionDialog method, the dialog box will function properly even if every parameter except the value of the array is set to null or zero:
String[] options = { "brownie", "pie", "cake" }; int response = JOptionPane.showOptionDialog(null, null, null, 0, 0, null, options, null);
Furthermore, the JOptionPane class defines enum data types for the optionType and messageType parameters, as demonstrated below:
String[] options = { "brownie", "pie", "cake" }; int response = JOptionPane.showOptionDialog(null, null, null, JOptionPane.DEFAULT_OPTION, JOptionPane.QUESTION_MESSAGE, null, options, null);
Advanced JOptionsPane’s showOptionDialog example
To make the most use of the JOptionsPane’s showOptionDialog method, a developer should take advantage of all of the available parameters.
The following advanced JOptionsPane’s showOptionDialog example takes advantage of each parameter. It adds a red JFrame
upon which to display the dialog box, and an image icon featuring the Java mascot Duke. An image of the JOptionPane this code creates is shown below the code.
import java.awt.Color;
import javax.swing.*;
public class JOptionPaneExample {
public static void main(String[] args) {
String[] options = { "brownie", "pie", "cake" };
int x = JOptionPane.showOptionDialog(null, null, null,
JOptionPane.DEFAULT_OPTION, JOptionPane.QUESTION_MESSAGE,
null, options, null);
JFrame frame = new JFrame();
frame.getContentPane().setBackground(Color.blue);
frame.setBounds(300, 300, 500, 350);
frame.setVisible(true);
int dessert = JOptionPane.showOptionDialog(frame,
"Which dessert would you like?", "Select a dessert",
JOptionPane.DEFAULT_OPTION, JOptionPane.QUESTION_MESSAGE,
new ImageIcon("C:\\_tools\\java-duke-sm.jpg"),
options, options[0]);
if (dessert == 0) {
JOptionPane.showMessageDialog(frame, "You chose a brownie.");
}
if (dessert == 1) {
JOptionPane.showMessageDialog(frame, "You chose pie.");
}
if (dessert == 2) {
JOptionPane.showMessageDialog(frame, "You chose cake.");
}
}
}
When the JOptionPane showMessageDialog() function runs, the dialog box appears on top of a blue frame, with Java’s Duke mascot as the icon.
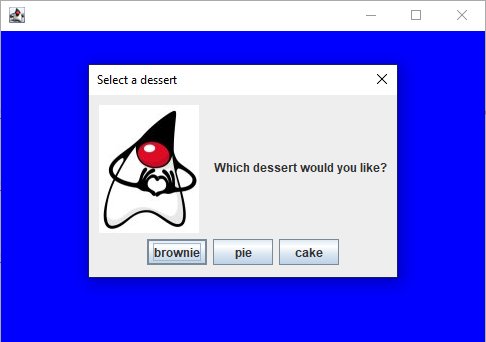
When fully parameterized, the JOptionPane showOptionDialog displays on a frame with a custom icon.
Garnering user input in Java programs can be difficult. The JOptionPane’s showMessageDialog() method makes it possible to create engaging, visual input forms that improves the experience of your users.