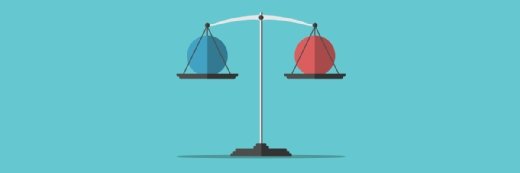
Java Iterator vs. Enumeration: Why Iterator is the right call
Do you need to loop through a collection of objects and need to decide between a Java Iterator or an Enumeration? Here's why you should choose an Iterator almost every time.
Developers have two options when they're faced with the need to loop through the contents of a Java collection class. They can use either the Java Iterator or the Enumeration. However, when it comes to modern applications, developers should almost always defer to the Iterator and leave the Enumeration type alone.
The Enumeration was originally part of the JDK 1.0 release and was designed to aid developers who needed to loop through Vectors, process HashTable keys or pass data to a SequenceInputStream. While an Enumeration still works for these functions, it has largely been replaced by the Iterator.
The entire collections API was revamped in Java 2, with new collection classes and interfaces added to the JDK. All these new classes can transform into an Iterator, which has usability improvements over the Enumeration.
All the classes released in Java 2 that implement the collection interface contain an iterator() method, which returns an instance of the Iterator. There's nearly universal support for the Iterator interface in Java, which serves as one of the primary reasons developers should chose it over the Enumeration.
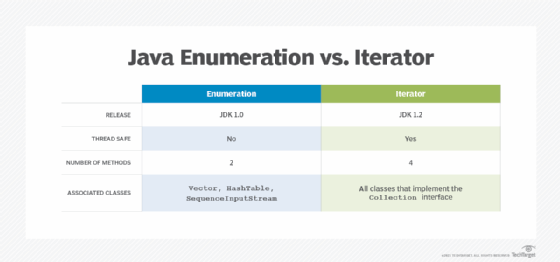
Java Iterator vs. Enumeration methods
Another reason why developers most often choose the Iterator is its ease of use and its shorter method names. For reference, the two key methods of the Enumeration are:
hasMoreElements() -- checks to see if more objects exist in the underlying collection class
nextElement() -- extracts the next queued object in the Enumeration
The methods of the Iterator are:
hasNext() -- checks to see if more objects exist in the underlying collection class
next() -- extracts the next queued object in the Enumeration
remove() -- allows an element to be removed from the collection after next() is called
forEachRemaining() -- performs a function on all remaining items in the collection
The remove() method in the Iterator allows items to be removed from the underlying collection in a fail-safe operation manner and will avoid unexpected exceptions.
The Enumeration doesn't have a remove() method. While a lack of methods might not seem like a positive attribute, the lack of this method allows a developer to treat the underlying data as a read-only collection. From my perspective, this is the Enumerator's only possible application in modern programming.
In all other cases, the Java Iterator is your best choice.
About the author
Dmytro Vezhnin is CEO and co-founder at CodeGym.cc, an interactive educational platform where people can learn Java programming language from scratch to Java Junior level.