2023 Java roadmap for developers
Beginner to advanced Java roadmap
Software development is one of the most rewarding careers in the world of IT.
Java is one of the most in-demand programming languages around.
If the Java platform calls to you, and you want to immerse yourself in the world of Spring, Jakarta EE, Maven, Jenkins and Android development, here’s the 2023 Java roadmap for developers to follow.
Roadmap for Java
Here are the steps you’ll want to follow on the 2023 Java developer career roadmap:
- Learn the fundamentals.
- Get object-oriented.
- Install the JDK and an IDE.
- Dig deeper into the API.
- Build out your core Java skills.
- Master collections.
- Get Functional.
- Straddle the middle-tier.
- Learn Java DevOps tooling.
- Study the JVM and the Java platform.
Learn the fundamentals of Java
At their core, all programming languages are the same.
All a computer program can really do is:
- Manage data through the declaration of variables.
- Perform conditional logic with if … else statements.
- Do all of this repeatedly and fast with iterative loops.
Your first stop on the Java developer’s roadmap is to learn these fundamentals.
Learn Java fast
It’s easy to get started with Java.
You don’t even have to install an IDE or the JDK. You can learn the basics of Java online with sites like Onecompiler and Replit.
You can make learning Java even easier if you use JShell.
It’s much easier to learn Java through the JShell scripting environment than by creating Java apps with the intimidating public static void main method declaration in them. JShell will make your learning journey much easier.
Once you’re set up on Replit or Onecompiler, create some basic applications that force you to learn loops, conditional logic and variable declarations. Popular projects that drive the learning include:
- The number guesser.
- Rock paper scissors.
- A to-do list.
- A simple calculator.
When you’ve got the fundamentals under your belt, you’re ready to learn about object-oriented analysis and design.
Object-oriented programming concepts
In terms of loops, variables and conditional statements all programming languages are pretty much the same. Where programming languages differ is how they organize data.
Java is an object-oriented programming language, which means it organizes data into components known as objects. That means the next stop on the 2023 Java developer’s roadmap is to learn object-oriented analysis and design.
Java object-oriented analysis and design concepts
In Java, object-oriented programming means you organize your data into several categories:
- Classes.
- Interfaces.
- Enumerations.
- Java Records.
To really understand how objects work, you’ll also want to learn how to apply object-oriented concepts such as:
- Abstraction.
- Encapsulation.
- Inheritance.
- Composition.
- Polymorphism.
With these components and concepts under your belt, you’ll be an object-oriented expert.
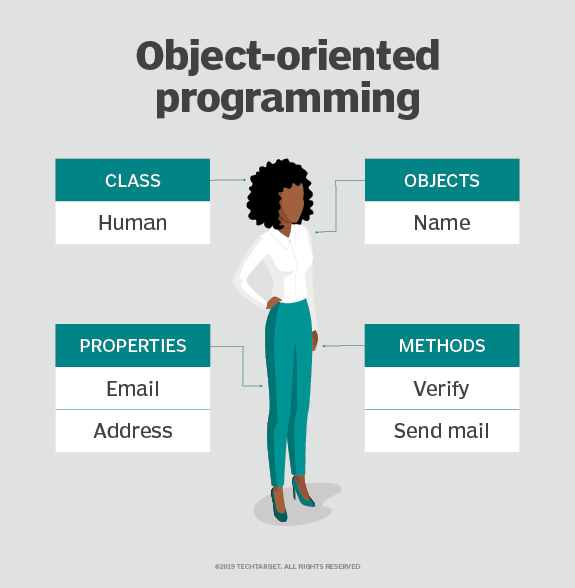
The development of object-oriented programming skills is an important stop on the Java roadmap for developers.
Install the JDK and an IDE
You can delve deep into the fundamentals of Java using online compilers and tools, but there comes a time when you need the power of an IDE. That’s the next stop on the 2023 Java roadmap for developers.
Install the JDK
At the very least, a Java developer should have the latest long-term support (LTS) release of Java installed on their local machines, along with a modern IDE such as:
- Eclipse.
- NetBeans.
- Visual Studio.
- IntelliJ by JetBrains.
Unlike the other three, IntelliJ isn’t free, but it’s widely used in the corporate world, and there are trial licenses available.
If an open-source alternative is more your style, try any one of Eclipse, NetBeans or Visual Studio.
Explore the JDK
Once you’ve installed the JDK, you should dig around in the \bin directory and explore the various tools packaged there, such as:
- The javac compiler.
- The Javadoc generator.
- The JShell editor.
- The javap class disassembler.
- JStat for metrics gathering.
- The Java Flight Recorder.
You’ll be amazed at how many full-featured performance, monitoring and troubleshooting tools come bundled with the JDK.
Explore the standard Java APIs
With the assistance of a full-featured IDE, the next stop on the Java developer roadmap is to explore the many standard Java APIs that come packaged with the JDK.
There are hundreds of packages and classes that come standard with the JDK. With these, you can accomplish many tasks, including:
- Create your own web server with the java.nio package.
- Access a database with JDBC components.
- Create simple windows apps with Java Swing.
- Create threaded apps with the concurrency APIs.
Very few programming languages come with as many APIs as Java.
Use these APIs to further build your knowledge of Java programming.
Strengthen your Core Java skills
After rushing through the standard APIs with an IDE, it’s time to get back to basics and focus on some core Java constructs including:
- Generics.
- Modules.
- Exception handling.
- Regular expressions.
Java generics
Java’s type system can be overwhelming at the start, and most developers gloss over Java generics at the onset of their learning journey.
However, a strong knowledge of generics in Java will give you a deeper understanding of how Java works — and more importantly, how Java differentiates itself from languages such as Python and JavaScript.
Java modules
Java’s module system is relatively new, and developers who are learning the language often skip modules as an unnecessary distraction. Yet, they are an incredibly powerful feature of the Java platform, and developers are wont to learn it.
Java exception handling
Exception handling is another topic that new Java learners often put off at the start.
Developers need to learn the fundamentals of the try…catch…finally block in Java, along with helpful syntactic sugar such as:
- Try with resources initializations.
- Closeable interfaces.
- Suppressed exceptions.
- Checked vs unchecked exceptions.
- Errors and RuntimeExceptions.
Regular expressions
To search and parse text, nothing beats Regular Expressions, or RegEx. However, they do require a day or two of practice to get the syntax right.
Learn RegEx.
Every software development team needs at least one member who knows how to find all the email addresses and phone numbers in a gigabyte of unstructured text.
Master the Java Collection classes
Much of the day-to-day work we do as Java developers boils down to list processing.
Java has an extensive Collections API with a variety of specialty components that solve unique data processing problems.
Collection-related concepts you’ll want to familiarize yourself with include:
- ArrayLists vs. Vectors.
- HashMaps vs. Hashtables.
- Lists vs. Sets.
Individual collection classes you’ll want to learn about include:
- Iterator.
- Dictionary.
- LinkedList.
- Queue.
- Dequeue.
- ListIterator.
- SortedSet.
- TreeSet.
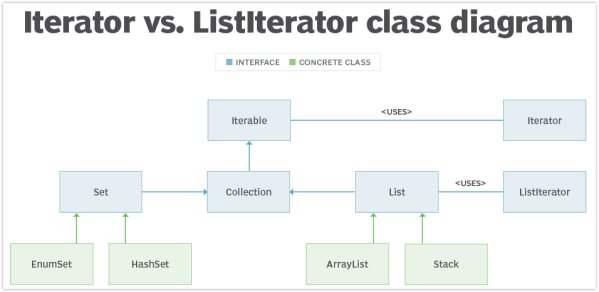
List processing is an important topic for Java developers and must be included on any roadmap for Java programmers.
Get functional
The introduction of functional programming into the Java programming language, along with a variety of components that take advantage of the functional programming paradigm, is the most drastic change to come to the Java programming language in decades.
To get familiar with functional programming, first learn how lambda expressions work in Java.
Then get familiar with the functional interfaces often used in a lambda expression, including the following:
- Consumer.
- Supplier.
- Predicate.
- Function.
These interfaces, and derivatives of them, pepper the entire Java landscape. Become familiar with them and you’ll find it much easier to pick up more advanced Java concepts such as Streams.
Master Streams
As mentioned earlier, much of what a developer does is manipulate collections of data. Also, the introduction of functional programming was a giant change in the Java platform.
Combine the two, and you get the Java Streams API.
Java Streams API
A Java Stream is a special pipeline for data processing, filtering and aggregation.
Java streams are incredibly efficient and powerful. They’re also incredibly intimidating when you first encounter them.
However, Java streams are self-explanatory when you understand the concepts behind them.
Some of the key Streams API methods you’ll want to learn to solidify your understanding of Java Streams include:
- count and distinct.
- findFirst and findAny.
- peek and filter.
- map and reduce.
- noneMatch.
- min and Max.
Modern Java development requires an in-depth knowledge of Streams. This is an important stop on the 2023 Java roadmap for developers.
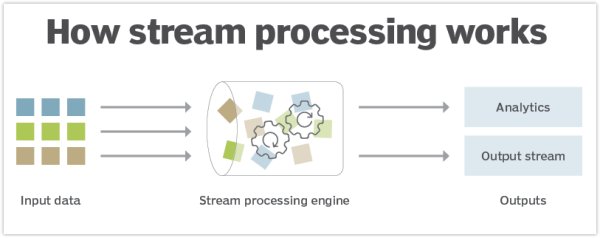
The Java roadmap for developers is incomplete without a strong understanding of the Java Streams API.
Jakarta EE and Spring Boot
Java has always been a major contender in the middle-tier space, and the industry-wide shift to microservices makes Spring Boot and the Jakarta EE frameworks more relevant than ever.
Jakarta EE is the evolution of the Java EE middle-tier development framework. Oracle ultimately handed Java EE over to the Eclipse Foundation, which is why it is now known as Jakarta.
Within Jakarta EE you will find important enterprise APIs, some of which data back to the days of J2EE, including:
- Context and Dependency Injection
- The Java Persistence API (JPA)
- Servlets and JSPs
- Web APIs for RESTful web services
- JavaServer Faces
- The Validation API
Spring Boot microservices
Spring Boot is an open-source framework designed to help Java developers quickly build cloud-native microservices.
Some Spring Boot packages simplify integration with JakartaEE APIs such as JPA. Others simplify access to core Java APIs like JDBC, Others, like WebMVC, provide an entirely independent alternative to JakartaEE APIs including JavaServer Faces and JavaMVC.
Spring vs Jakarta
Jakarta EE and Spring Boot solve similar problems. With knowledge of one of these, a Java developer can quickly get up to speed on the other.
Which one a developers chooses to learn first isn’t an issue, so long as a developer commits to learn one of them.
Due DevOps diligence
DevOps is all about motivated developers who share an Agile mindset using the best tools available to create new processes that aid in the continuous delivery of software.
A successful Java developer must know the tools that drive DevOps on the Java platform.
The most important Java DevOps tools in use today include:
- Git and GitHub for source code management.
- Apache Maven and Gradle build tools.
- Jenkins for continuous integration.
- JUnit and Mockito for testing.
- SonarQube for static code analysis.
- Artifactory for repository management.
- Docker for containerization.
- Java Mission Control for runtime monitoring.
- Cloud computing with GCP, AWS and Azure.
The combination of these tools and technologies allows developers to move beyond continuous integration into the modern sphere of continuous delivery, and eventually continuous software deployment.
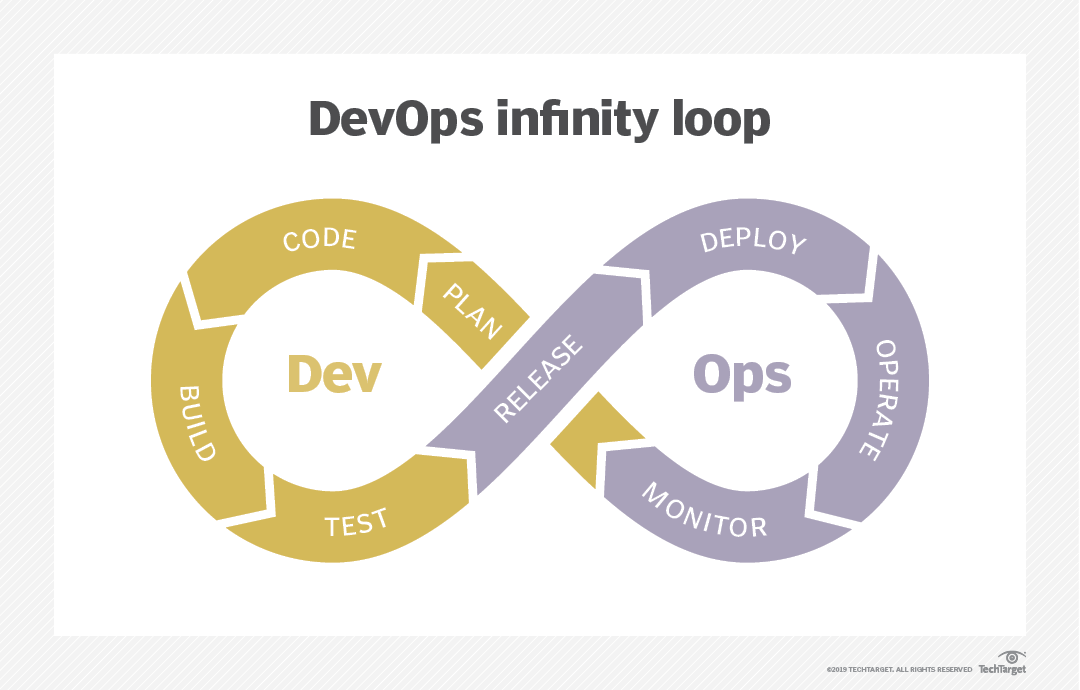
An understanding of DevOps is an important part of the career roadmap for Java developers.
Understand the Java platform
Good Java developers know the Java programming language. Great Java developers understand the Java platform.
To be a great Java developer, you must learn about the intricacies of the Java platform, including the following:
- How Java allocates memory.
- How garbage collectors work.
- How to convert Java to WebAssembly.
- How to decompile Java code.
- How the JVM schedules threads.
- How object serialization works.
An understanding of these topics will give you a greater appreciation of how the Java platform works, which will help you troubleshoot low-level JVM issues when they arise.
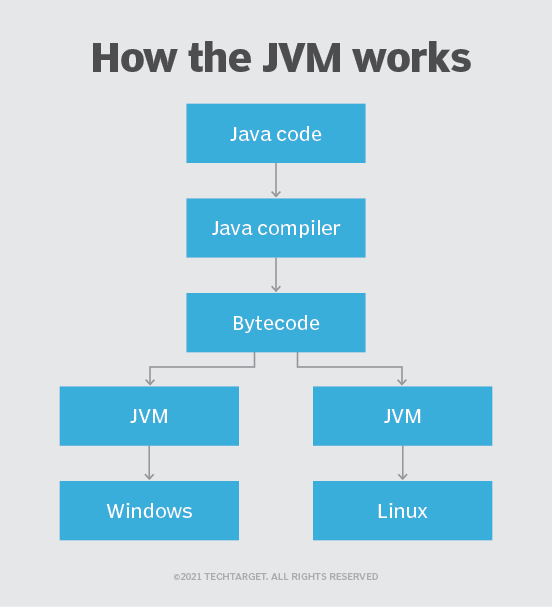
The last stop on the Java roadmap for developers is to understand how the JVM works.
The 2023 roadmap for Java
There’s a lot to cover on the 2023 Java roadmap for developers, but it’s worth it if you want a rewarding career in the software development industry as a Java programmer.