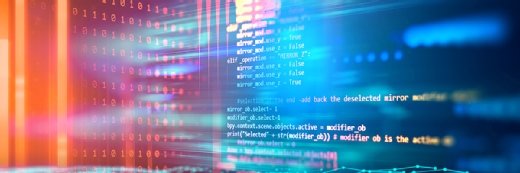
Getty Images/iStockphoto
JavaScript vs. TypeScript: What's the difference?
TypeScript and JavaScript are two complementary technologies that are driving both front-end and back-end development. Here are the similarities and differences between the two.
Difference between TypeScript and JavaScript
JavaScript and TypeScript look very similar, but there's one important distinction.
The key difference between JavaScript and TypeScript is that JavaScript lacks a type system. In JavaScript, variables can haphazardly change form, while TypeScript in strict mode forbids this. This makes TypeScript easier to manage and maintain, especially with a large codebase.
JavaScript vs. TypeScript example
TypeScript's type system has a minor impact on how variables are referenced and declared, yet it has a huge impact on maintainability and consistency.
For example, here is how to declare numeric- and text-based variables in JavaScript:
let foo = 1
let bar = "text"
bar = 123 // allowed in JavaScript
Here are the same variables declared and initialized in TypeScript:
let foo: number = 1
let bar: string = "text"
bar = 123 // not allowed in TypeScript
The difference in syntax is minor.
However, the use of number and string types allows TypeScript to enforce the rule that: If a variable is declared as a given type, it is not allowed to arbitrarily change its type later on in the program.
This rule is why strict TypeScript does not allow the variable bar to switch from a text string to a number. JavaScript would allow it.
The introduction of a type system doesn't seem like a big deal, but it has a major impact on how to manage large applications.
With TypeScript, when a variable is assigned a new value, IDEs and validation tools can quickly check that change against tens of thousands of lines of code. The same type of check is difficult to perform with JavaScript. This increases the risk of error when extending, updating and troubleshooting JavaScript.
The presence of type checking also allows IDEs to include validation and refactoring capabilities that are not possible with a language that is not type checked. There are powerful IDEs for both TypeScript and JavaScript, but the TypeScript IDEs tend to be packed with more features that help build, troubleshoot and refactor code.
From TypeScript to JavaScript
TypeScript is not a replacement for JavaScript.
TypeScript is simply a more feature-full and technically sound way to write JavaScript.
To run an application written in TypeScript, the first step is to compile the code into JavaScript. TypeScript is simply a better way to write JavaScript code that is ECMAScript-compliant to ensure interoperability across web browsers.
You can think of TypeScript as just a JavaScript generator. TypeScript helps you create JavaScript code that can run in any environment that supports the JavaScript standard.
The shortcomings of JavaScript
JavaScript was originally invented to run inside a circa 1995 version of Netscape Navigator. At that time, developers made design decisions to address what today seem like unimaginable constraints, such as the following:
- Computers with single-core processors.
- CPU speeds measured in double-digit megahertz, not gigahertz.
- Devices with less than 1 MB of RAM.
- Typical download speeds of 14.4-56 Kbps.
- No access to network ports or the file system.
- Execution entirely within the confines of a webpage.
The JavaScript language was built tactically to provide simple, core functionality to web developers.
Thirty years later, cracks in the JavaScript armor now show, especially as developers use the language to build progressive web apps with Angular and React, or sophisticated back-end microservices with NodeJS.
To address the shortcomings of JavaScript, Microsoft invented TypeScript in 2012.
TypeScript was designed from the ground up to be an elegant, fully object-oriented programming language that provides functional features and optional design-time type-checking.
With TypeScript, developers can use a language that's as elegant, philosophically sound and feature-rich as C# or Java. Upon compilation, TypeScript turns into cross-platform JavaScript code guaranteed to work in any ECMAScript-compliant runtime.
JavaScript and TypeScript compared | ||
Criteria |
JavaScript |
TypeScript |
First release |
1995 |
2012 |
Created by |
Netscape |
Microsoft |
Trademark owner |
Oracle |
Microsoft |
Standard |
ECMAScript 5 |
ECMAScript 2015 |
Typing |
Dynamic |
Optional strong typing |
Community and adoption |
Larger than TypeScript |
Smaller than JavaScript |
Compilation |
Not required |
Compiled into JavaScript |
File extension |
.js and .jsx |
.ts and .tsx |
IDE support |
Limited validation and refactoring capabilities |
Extensive validation and refactoring capabilities |
Complexity |
Easy scripting language for new developers to learn |
Additional features require an investment of time to learn |
Readability |
Large code bases are harder to read and decipher |
Type system and object-oriented approach improve readability |
Compatibility |
All JavaScript code is also valid TypeScript code |
TypeScript code gets compiled into fully compliant JavaScript |
ECMAScript vs. TypeScript
ECMAScript is the standard to which all JavaScript code must comply.
A vendor such as Microsoft cannot arbitrarily add features to the JavaScript language without breaking ECMAScript compliance.
In contrast, with TypeScript, Microsoft can add any new features it wants, so long as the generated JavaScript is ECMAScript-compliant. That gives Microsoft all the freedom in the world to make TypeScript as feature-full as any other programming language, including Java, Rust, Golang and C.
TypeScript and JavaScript compared
In terms of features, here are 10 significant differences between JavaScript and TypeScript:
- TypeScript can be strongly typed, while JavaScript is dynamically typed only.
- TypeScript is more readable and maintainable than JavaScript.
- TypeScript supports abstraction through interfaces, while JavaScript does not.
- TypeScript allows developers to annotate code with decorators, while JavaScript does not.
- TypeScript supports the ability to modularize and organize components through the use of namespaces, which is not supported in JavaScript.
- TypeScript is more expressive than JavaScript, through the use of syntax elements such as optional and named parameters.
- TypeScript supports generics and a type inference feature that is not available in JavaScript.
- TypeScript IDEs have more features, as it is easier to build plugins and tools for a statically typed language.
- TypeScript code is easier to debug as the codebase expands, because type errors can be discovered at compilation time rather than runtime.
- TypeScript implements additional features beyond the limited ECMAScript specification to which JavaScript complies.
TypeScript makes JavaScript better
TypeScript isn't a competitor to JavaScript. Instead, TypeScript complements JavaScript.
TypeScript provides the community with a more dynamic, full-featured and safer way to develop enterprise-grade applications where the target runtime requires JavaScript.
TypeScript isn't designed to replace JavaScript. Instead, its purpose is to encourage the proliferation of JavaScript-based platforms by making it easier to write, integrate, manage and maintain code.
JavaScript-driven platforms such as NodeJS on the server and ReactJS on client side continue to gain in popularity. The ability to write code in TypeScript and turn it into JavaScript is one of the reasons adoption rates for both languages continue to climb.