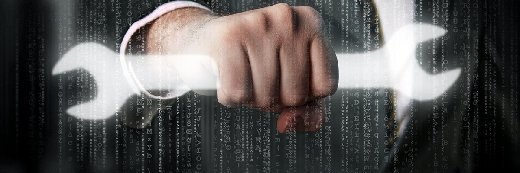
beawolf - Fotolia
Fix broken builds with this log4j Maven dependency example
DevOps professionals need to know how Maven dependency management works. This log4j Maven dependency example demonstrates how to use Maven Central and resolve external libraries.
Apache Maven isn't just a build tool. The true value of Maven is as a dependency manager for Java applications, especially when you need to resolve multiple external libraries at compile time, during test execution and at deployment.
Anyone with experience building enterprise Java software knows that consolidating external libraries and fighting either ClassNotFound exceptions or classloader issues can be a nightmare. But such frustration is a thing of the past, as the elegant Maven dependency management facilities make linking to external libraries a lead-pipe cinch. This Java build tutorial, which uses a log4j Maven dependency example, will demonstrate exactly how to resolve external libraries and pull from a remote repository, like Maven Central.
Create a Maven project
The first step to see Maven dependency management facilities in action is to create a basic Maven project using the mvn command-line tool:
C:\_maven projects>mvn -B archetype:generate
-DarchetypeGroupId=org.apache.maven.archetypes
-DgroupId=com.log4j.maven
-DartifactId=dependency-example
Run on a single continuous line, this command creates a Java-based Maven project named dependency-example. Because of the -DgroupId flag used in the command just issued, Maven will put a file named App.java in the com\log4j\maven folder of the project's source code branch. It is this Java file in which we will code a log4j dependency.
Code in the log4j Maven dependency
Before we add the log4j dependency, the updated App.java code looks as follows:
package com.log4j.maven;
/**The log4j Maven dependencies example code*/
public class App {
public static void main( String[] args ){
System.out.println( "Hello World!" );
}
}
After sprinkling in some log4j, the code takes on this appearance. We've highlighted the lines of code changed to create the log4j dependency:
package com.log4j.maven;
import org.apache.log4j.Logger;
/**The log4j Maven dependencies example code*/
public class App {
static Logger log = Logger.getLogger(App.class);
public static void main( String[] args ){
log.debug( "Maven dependency log4j..." );
}
}
You can try to compile the code with the following Maven command, but the build will fail due to the unresolved log4j Maven dependency.
C:\_maven projects\dependency-example>mvn compile
When the mvn compile command runs, the result is a build failure with the console output indicating that the Java package org.apache.log4j does not exist.
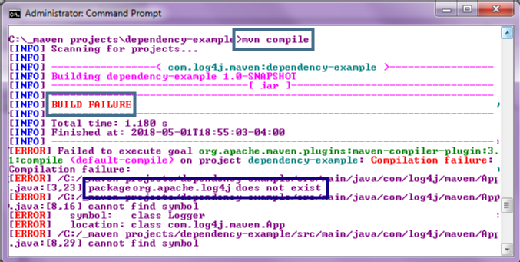
Maven dependency management to the rescue
The solution to the unresolved log4j Maven dependency resides entirely in the pom.xml file.
Placed in the dependencies-example root folder when the Maven project of that name was created, you'll notice upon opening the pom.xml file up for edits that it already has an existing dependency for JUnit:
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>com.log4j.maven</groupId>
<artifactId>dependency-example</artifactId>
<packaging>jar</packaging>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
Resolve external libraries with Maven Central
At this point, we know the inability to reference the log4j libraries is what's breaking the build. But how do you go about figuring out what to put in your POM (Project Object Model) to get the code to compile?
To figure out how to incorporate the log4j Maven dependency, search Maven Central for information about log4j support. When you locate the log4j project page on Maven Central, you'll find a snippet of XML that will enable the log4j Maven dependency to be resolved the next time the build is run.
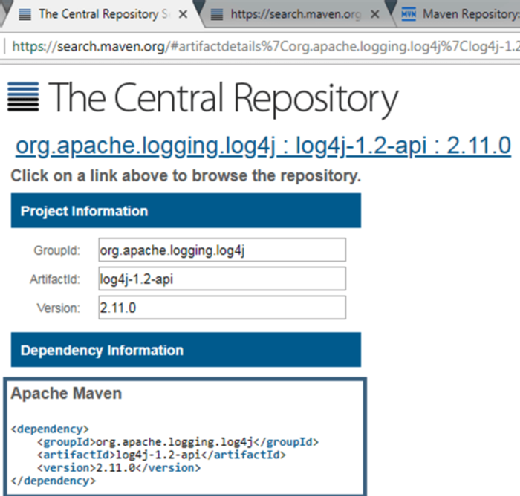
Correct the log4j Maven dependencies example POM
When you add the log4j Maven dependency, the consolidated pom.xml file looks as follows:
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>com.log4j.maven</groupId>
<artifactId>dependency-example</artifactId>
<packaging>jar</packaging>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-1.2-api</artifactId>
<version>2.11.0</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
Maven dependency management at work
With the log4j dependency added and the pom.xml file saved, the mvn compile command will run successfully, fixing the broken Maven build job.
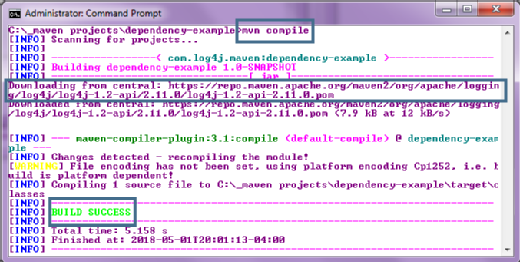
As you can see from the screenshot of the build, when the mvn compile command executes, Maven actually reaches out across the internet to Maven Central in order to locate and download the log4j library locally. When the source code is compiled, the log4j Maven dependency is easily resolved, and the code compiles. The build is a success.
TheServerSide Maven guide
Part 1 -- "How to install Maven and build apps with the mvn command line"
Part 2 -- "Fix broken builds with this log4j Maven dependency example"