Simple Java 8 Predicate example with lambda expressions and interfaces
A great deal of Java programming -- from conditional statements to iterative loops -- deals with the evaluation of true or false values. When you work with the JDK's Streams API and Lambda functions, you can use the ever-popular Java Predicate interface to help simplify the evaluation of boolean conditions.
Also known as the Java 8 Predicate -- which stems from the JDK version where functional programming was introduced to developers -- this simple interface defines five methods, although only the Java Predicate's test method is evaluated when it's used in a Stream or a lambda call.
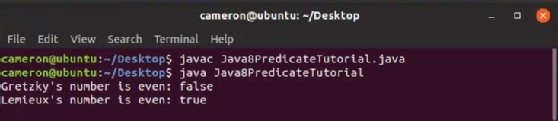
Traditional Java 8 Predicate example
While the Java 8 Predicate is a functional interface, there's nothing to stop a developer from using it in a traditional manner. Here's a Java Predicate example that simply creates a new class that extends the Predicate interface, along with a separate class that uses the Predicate in its main method:
import java.util.function.*;
public class Java8PredicateTutorial {
public static void main(String args[]) {
PredicateExample example = new PredicateExample();
System.out.printf("Gretzky's number is even: %s", example.test(99));
boolean value = example.test(66);
System.out.printf("\nLemieux's number is even: %s ", value);
}
}
class PredicateExample implements Predicate<Integer> {
public boolean test(Integer x) {
if (x%2==0){
return true;
} else {
return false;
}
}
}
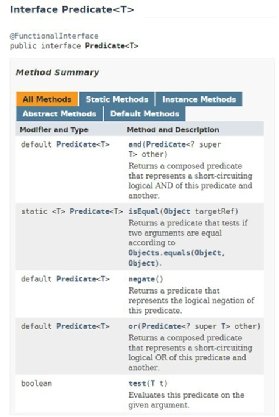
Figure 2 shows the results when you compile and execute this Predicate interface tutorial:
Java Predicate as an inner class
If you're a developer who prefers inner classes, you could clean this up a bit and reduce the verbosity of the example. But, the resulting Java 8 Predicate example doesn't quite qualify as an example of functional programming:
import java.util.function.*;
public class Java8PredicateTutorial {
public static void main(String args[]) {
Predicate predicateExample = new Predicate<Integer>() {
public boolean test(Integer x) {
return (x % 2 == 0);
}
};
System.out.printf("Gretzky's number is even: %s", predicateExample.test(99));
System.out.printf("\nLemieux's number is even: %s ", predicateExample.test(66));
}
}
Java Predicate lambda example
Of course, if you're learning about the Java 8 Predicate interface, you're most likely interested in how to use it in a lambda function.
The goal of a lambda expression is to reduce the verbosity of Java code, especially for those situations where you need to override an interface with only one functional method. Here is how the code creates a Java Predicate with a lambda expression:
Predicate<Integer> lambdaPredicate = (Integer x) -> (x % 2 == 0);
Compared with the traditional methods for interface creation, there's no debate that lambda expressions are much more concise.
Here is the full Java Predicate example implemented with a lambda expression:
import java.util.function.*;
public class Java8PredicateTutorial {
public static void main(String args[]) {
/* Java predicate lambda example */
Predicate<Integer> lambdaPredicate = (Integer x) -> (x % 2 == 0);
System.out.printf("Gretzky's number is even: %s", lambdaPredicate.test(99));
System.out.printf("\nLemieux's number is even: %s ", lambdaPredicate.test(66));
}
}
Java Predicate and lambda streams
The use of functional expressions has been peppered throughout the Java API ever since the release of JDK 8. The Streams API uses lambda expressions and the Java Predicate extensively, with the filter expression as one such example. Here's a look at a lambda, stream and Predicate example in which all the even numbers are pulled out of a list of Integer objects:
import java.util.function.*;
import java.util.*;
import java.util.stream.*;
public class LambdaPredicateStreamExample {
public static void main(String args[]) {
List<Integer> jerseys = Arrays.asList(99, 66, 88, 16);
/* Java predicate and lambda stream example usage */
List<Integer> evenNumbers =
jerseys.stream()
.filter( x -> ((x%2)==0))
.collect(Collectors.toList());
/* The following line prints: [66, 88, 16] 8 */
System.out.println(evenNumbers);
}
}
As you can see, the combined use of lambda functions, streams and the Java Predicate interface can create very compact code that is as powerful as it is easy to read.