How do I find the Java String length?
How to find the length of a Java String
Follow these steps to find the length of a String in Java:
- Declare a variable of type String
- Initialize the String variable to a non-null value
- Call the Java String length() method
- Hold the value of the String length in a variable for future use
Java String length method()
The Java String class contains a length() method that returns the total number of characters a given String contains.
This value includes all blanks, spaces, and other special characters. Every character in the String is counted.
String Class JavaDoc (Java 17) public int length() - returns the number of characters in a text string
Java String length() example
Here is a simple example of how to find the length of a String in Java and print the value out to the console:
String javaString = " String length example "; int stringSize= javaString.length(); System.out.println(stringSize); //This Java String length example prints out 25
How do I remove whitespace from a Java String’s length?
In the above example of Java’s String length method, the output for the size of the String is 25. The String length method includes the whitespace padding at the beginning and the end of the String.
Quite often, when validating input or manipulating text, you want to eliminate leading and trailing whitespace. This can be achieved through the use of the Java String’s trim method.
String javaString = " String length example "; int stringSize= javaString.trim().length(); System.out.println(stringSize); //This Java String length trim example prints out 21
As you can see with the example above, the whitespaces are not included in the calculation of the length of a String when the trim method is called first.
String method | Method function |
trim() | removes whitespace before the Java String’s length method is called |
charAt(int index) | Returns the character at a given position in a String |
toUpperCase() | Converts all characters in a String to uppercase |
toLowerCase() | Converts all characters in a String to lowercase |
substring(int index) | Returns a subset of the Java String |
String length method vs property
Be careful not to confuse the String length() method with the length property of an array.
The Java String length() method of an array is followed by round brackets, while the Java String length property is not.
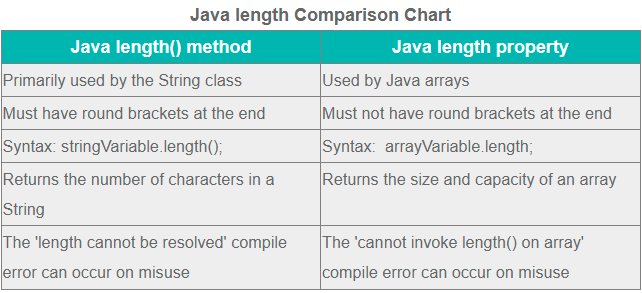
Developers often confuse the Java String length method with the length property of an array.
String length compile errors
If you leave the round brackets off the Java String’s length method, the following compile time error results:
Java String error: length cannot be resolved or is not a field
Also be sure to initialize the Java String before you invoke the length() method, or else a NullPointer runtime exception results.
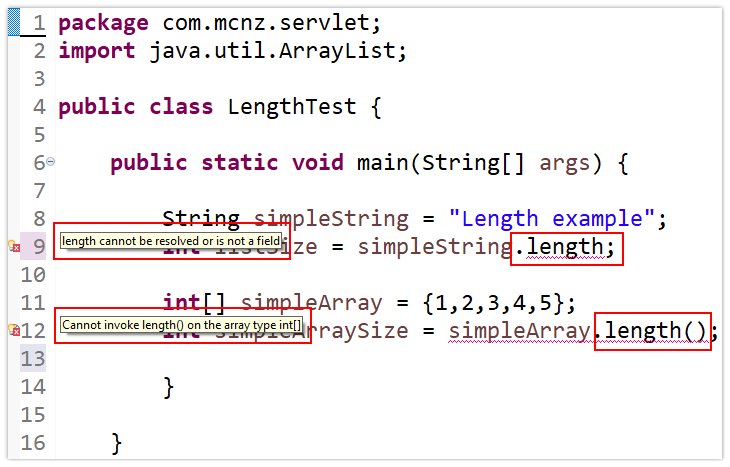
To find the length of a String, make sure you invoke the length() method, not the length property.
Advanced Java String length example
There are many scenarios where a program must first find the length of a Java String.
Here is a rather advanced piece of code that checks to see if a given String is a palindrome. I used a variety of methods from the String class, including length(), charAt() and substring().
package com.mcnz.servlet;
/* Find the length of a Java String example */
public class JavaPalindromeCheckProgram {
public static void main(String[] args) {
boolean flag = palindromeCheck("amanaplanacanalpanama");
System.out.println(flag);
}
/* This code uses the Java String length method in its logic */
public static boolean palindromeCheck(String string){
/* check if the Java String length is zero or one */
if(string.length() == 0 || string.length() == 1) {
return true;
}
if(string.charAt(0) == string.charAt(string.length()-1)) {
return palindromeCheck(string.substring(1, string.length()-1));
}
return false;
}
}
Using Java’s String length method
To get the number of characters in a given piece of text, the Java String length() method is all you need.
Just make sure the String is not null, and avoid any confusion between Java’s length vs length() constructs, and you will have no problem manipulating text Strings in Java.