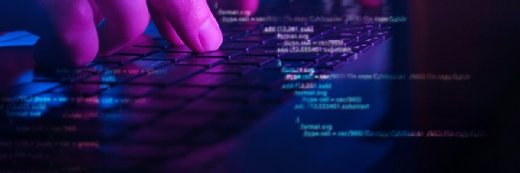
Getty Images/iStockphoto
Fix these 10 common examples of the RuntimeException in Java
Don't let the occurrence of a RuntimeException in Java bring your code to a standstill. Here are 10 examples of how to avoid runtime exceptions in Java.
Runtime errors occur when something goes wrong in the normal execution of a program. When severe enough, these errors abruptly terminate an application.
To help programmers both anticipate and recover from runtime errors, the Java programming language defines a special class named the RuntimeException.
Given their potential to stop an otherwise properly functioning program dead in its tracks, developers should grasp Java's most common RuntimeExceptions.
List of RuntimeException examples
The 10 most common examples of RuntimeExceptions in Java are:
- ArithmeticException
- NullPointerException
- ClassCastException
- DateTimeException
- ArrayIndexOutOfBoundsException
- NegativeArraySizeException
- ArrayStoreException
- UnsupportedOperationException
- NoSuchElementException
- ConcurrentModificationException
Anticipate the ArtithmeticException
Data manipulation is a primary function of most Java applications. When data manipulation includes division, developers must be wary of division by zero. If zero ever becomes the denominator in a calculation, Java throws an ArithmeticException.
int x = 100;
int y = 0; // denominator is set to zero
System.out.println( x/y ); // throws ArithmeticException
In this example, the denominator is explicitly assigned to zero, so it's obvious that a divide-by-zero error will occur. If user input sets the denominator, the problem is less predictable. That's why data cleansing is an important function of every application.
Negate the NullPointerException
The NullPointerException is a very common RuntimeException that Java applications encounter. Any time a developer writes code that invokes a method on an uninitialized object in Java, a NullPointerException occurs.
String data = null;
System.out.println( data.length() ); // throws NullPointerException
In this example, the developer sets the data variable to null, and then invokes the length() method. The invocation of the length() method on the null object triggers the NullPointerException.
How to avoid this RuntimeException in your Java programs? Initialize every object you create before you use it, and perform a null check on any object that an external library passes into your programs.
Conquer the ClassCastException
Java is a strongly typed language. It strictly enforces type hierarchies, and will not cast one object to another unless they share a polymorphic relationship.
In the following code, the attempt to cast the Date object into a Timestamp throws a ClassCastException error at runtime.
Date today = new Date();Timestamp time = (Timestamp)today;
Timestamp inherits from Date, so every Timestamp is a special type of Date. But polymorphism in Java is unidirectional -- a Date is not necessarily a Timestamp. If the cast went in the opposite direction, i.e., a Timestamp cast into a Date, the Java runtime exception would not occur.
long seconds = System.currentTimeMillis(); Timestamp time = new Timestamp(seconds); Date today = (Date)time; // this cast works
Defeat the DateTimeException
Data manipulation is a tricky endeavor. Time zones, datelines and inconsistent date formats can cause Java to throw various DateTimeExceptions at runtime.
For example, the following code compiles, but the LocalDate class' HourOfDay field will cause the attempt to format the date to fail.
LocalDate now = LocalDate.now(); DateTimeFormatter.RFC_1123_DATE_TIME.format(now);
Fortunately, there are numerous different date formats from which to choose. Switch to an ISO_DATE in this case and the Java runtime exception goes away.
LocalDate now = LocalDate.now(); DateTimeFormatter.ISO_DATE.format(now);
ArrayIndexOutOfBoundsException example
An array in Java requires a set size. If you attempt to add more elements than the array can accommodate, this will result in the ArrayIndexOutOfBoundsException. The following code attempts to add an element to the sixth index of an array that was built to contain only five elements:
String[] data = new String[5]; data[6] = "More Data";
Java applies zero based counting to elements in an array, so index 6 of an array would refer to the seventh element. Even the following code would trigger an ArrayIndexOutOfBoundsException in Java, as index 4 is a reference to an array's fifth element:
String[] data = new String[5]; data[5] = "More Data";
The inability of an array to dynamically resize to fit additional elements is a common runtime exception in Java, especially for developers who are new to the language.
Nullify the NegativeArraySizeException
Developers must set array size to a positive integer. If a minus sign slips into the array size declaration, this throws the NegativeArraySizeException.
String[] data = new String[-5]; // throws Runtime Exception data[1] = "More Data";
It's easy to avoid this Java runtime exception. Don't set an array's size to a negative number.
ArrayStoreException explained
The ArrayStoreException shares similarities with the ClassCastException. This Java runtime exception happens when the wrong type of object is placed into an array.
In the example below, a BigInteger array is created, followed by an attempt to add a Double. The Double does not share a relationship with the BigInteger, so this triggers an ArrayStoreException at runtime.
Number[] bigInt = new BigInteger[5]; bigInt[0] = Double.valueOf(12345);
One way to avoid this exception is to be more specific when declaring the array. If the array was declared as a BigInteger type, Java would flag the problem as a type mismatch error at compile time, not runtime.
BigInteger[] bigInt = new BigInteger[5]; bigInt[0] = Double.valueOf(12345); // Java compile error
To avoid the Java RuntimeException completely, declare the array as the least generic type, which in this case would be Double.
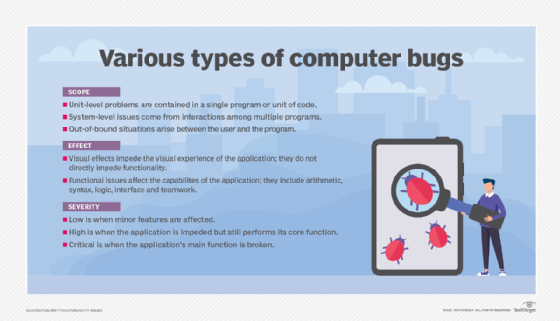
Unravel the UnsupportedOperationException
Java programmers often use the Arrays class to morph a Java array into a more user-friendly List. However, the List that Java returns is read-only. Developers who are unaware of this fact and try to add new elements run into the UnsupportedOperationException. This example shows how this happens:
Integer[] data = {1,2,3,5,8,13,21}; List<Integer> list = Arrays.asList(data); list.add(new Integer(0));
It's simple to avoid this common Java runtime exception. Don't add elements to a read-only List.
Knock out the NoSuchElementException
You can't iterate through an empty iterator. The following code, which attempts to get the next element in an empty HashSet, throws a NoSuchElementException.
Set set = new HashSet(); set.iterator().next(); // Java runtime exception thrown
To fix this Java runtime exception, simply check that the collection class is not empty, and only proceed if there are elements inside the iterator.
if (!set.isEmpty()) { set.iterator().next(); }
Correct the ConcurrentModificationException
The asList method of the Arrays class isn't the only time a collection requires the read-only treatment.
When you iterate over a list, the underlying collection must be fixed and not updated. Thus, the add method within the following snippet of code throws a ConcurrentModificationException.
List servers = new ArrayList(); servers.add("Tomcat"); Iterator<String> iterator = servers.iterator(); while (iterator.hasNext()) { String server = iterator.next(); servers.add("Jetty"); // throws a Runtime Exception }
It's impossible to anticipate every possible error that might occur in an application. However, knowledge of these 10 most common runtime exceptions in Java will help make you a more polished programmer, and your programs more effective and resilient.