Java's print vs println method: What's the difference?
What’s the difference between Java print and println methods
The key difference between Java’s print and println methods is that println appends a newline character (‘\n’) to output, while Java’s print method does not. Unlike println, the print method can be called multiple times and all text sent to the console will appear on the same line. By comparison, all subsequent calls to println place output on new lines.
For example, the following code snippet uses Java’s println method to output the words “Hello” and “World” to two separate lines:
System.out.println("Hello ");
System.out.println("world!");
Execution of this code results in the following output:
Hello world!
Java print method example
The next example is similar to the one above, with the only difference that it uses println vs print.
System.out.print("Hello ");
System.out.print("world!");
Execution of this code results in this output:
Hello world!
Java’s newline character
Java’s print method can be made to behave like the println method. Simply append the newline escape sequence (‘\n’) to the text String passed into the method, like so:
System.out.print("Hello " + "\n"); // newline added with + operator
System.out.print("world!\n"); // newline included in text String
This newline escape sequence example generates the following output when executed:
Hello world!
Should I use print or println in my code?
The choice between print and println hinges largely on what the developer hopes to achieve.
For example, a developer should use print methods if they want to describe the value of two variables with output like this:
The value of x is 25, and the value of y is 50.
A simple Java print method example
The code to achieve the above output is as follows:
long x = 25;
long y = 50;
System.out.print("The value of x is ");
System.out.print(x);
System.out.print(", and the value of y is ");
System.out.print(y);
System.out.print(".");
A simple Java println method example
In contrast to the above example, imagine the developer wants to display output in the following way:
The value of x is currently 25.
The value of y is currently 50.
The code to achieve this output relies on Java println method calls:
long x = 25;
long y = 50;
System.out.println("The value of x is currently " + x + ".");
System.out.println("The value of y is currently " + y + ".");
Java println vs printf methods
For advanced formatting of data and variables, Java provides a printf method that gives much more control to the developer.
Here is an example of how to use the Java printf method to format output that uses two text variables and adds a newline character:
String name = "Cameron"; String site = "TechTarget"; System.out.printf("I like the stuff %s writes on %S. %n", name, site); /* Printf output: I like the stuff Cameron writes on TECHTARGET. */
Java methods such as print, println and printf all offer the developer different ways to control output that gets sent to the console or streamed to the log files. However, for application logging, one should use a properly framework such as log4J. Application logging with Java’s print and println methods is not recommended.
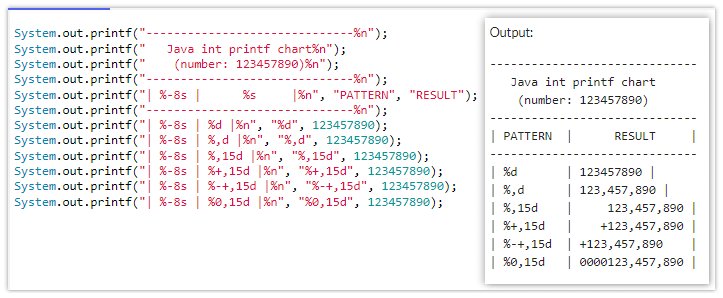
The printf method provides extra functionality not found in Java print and println methods.