String to long in Java
Convert a String to long in Java
The correct way to convert a String to long in Java is to use the parseLong(String x) method of the Long wrapper class.
The benefits of the parseLong(String) method to convert a String to a long or int in Java instead of the valueOf(String) or the deprecated contructor method include:
- The Long.parseLong() method returns a long primitive, not a wrapper.
- The Long.parseLong() method uses the Long class, which is semantically correct.
- The Long.parseLong() method does not require autoboxing.
- The Long.parseLong() method is not deprecated like the Long constructor.
Java String to long example
The following String to long example program converts the text String 90210 to a long, and then performs a math operation on the converted value to prove that it behaves like a primitive long type and not a Java String.
// String to long conversion program String beverly = "90210"; long hills = Long.parseLong(beverly); long twoHills = hills + hills; System.out.println(hills); // prints 90201 System.out.println(twoHills); // prints 180402
Other String to long conversion approaches
There are other, less technically correct ways to convert a text String to a long primitive type in Java. Those String to long conversion approaches include:
- Use the parse method of another wrapper class.
- Use the deprecated constructor of the Long class and autobox into a primitive long.
- Use the parse method of a different wrapper class, such as Integer.
Other parse String to long conversions
Every numeric wrapper class has a parse method that can be used to convert a text String to the associated primitive type:
- Integer has parseInt(String text).
- Short has parseShort(String text).
- Byte has parseByte(String text).
Each of these methods return a whole number that fits within the range of a long in Java. You can use them to convert a String to a long.
The following example uses the Integer wrapper class’ parse method to convert a text String to a long:
// Integer parseLong String to long example String text = "10"; long face = Integer.parseInt(text); long twoFace = face + face ; System.out.println( face ); // prints 10 System.out.println( twoFace ); // prints 20
The parse methods are preferable because they return a primitive type, and not a wrapper class like the valueOf method or the use of a Java constructor.
String to long conversions with valueOf
All wrapper classes in Java have a valueOf method that converts a text String into an instance of that wrapper class:
- Long.valueOf(String) converts a String into a Long instance.
- Integer.valueOf(String) converts a String into an Integer instance.
- Short.valueOf(String) converts a String into a Short instance.
- Byte.valueOf(String) converts a String into a Byte instance.
Note that an instance of the Long wrapper class is different from the lower-case long primitive type. Fortunately, Java’s autoboxing feature typically hides the differences between them, although the extra work Java performs to autobox primitive types comes with a cost.
Example valueOf text to long conversion in Java
Here’s a Java String to long example with the valueOf method:
// Text to Long in Java with valueOf String text = "10"; // Java String to long with autoboxing long face = Integer.valueOf(text); long twoFace = face + face ; System.out.println( face ); // prints 10 System.out.println( twoFace ); // prints 20
Deprecated constructor conversion of String to long
Every Java wrapper class has a deprecated constructor that takes a String as an argument and converts that String to the corresponding type.
- new Long(String text) converts a String to a Long
- new Integer(String text) converts a String to an Integer
- new Double(String text) converts a String to a Double
- new Boolean(String text) converts a String to a Boolean
In Java 17, these methods still work, but they are marked for removal so you should avoid this approach.
String to long program with constructors
The following Java String to long program uses a deprecated constructor and autoboxing to achieve the desired result:
// Text to Long with a deprecated constructor String textString = "20"; // The Long(String s) constructor is deprecated long face = new Long( textString ); long threeFace = face + face + face; System.out.print( face ); // prints out 20 System.out.print( threeFace ); // prints out 60
This approach works, but it generates the following warning message:
Main.java:9: warning: [removal] Long(String) in Long has been deprecated and marked for removal long face = new Long( textString );
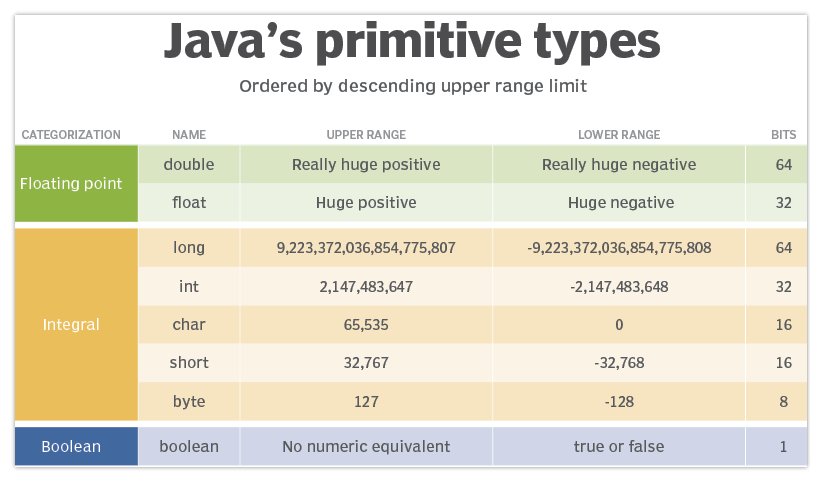
All primitive types provide a wrapper class that will perform functions like the String to long conversion.
By the way, you can also go the other way. Here are several ways to convert a long to String in Java so your Java builds don’t fail.