exception handler
What is an exception handler?
An exception handler is code that stipulates what a program will do when an anomalous or exceptional event occurs and disrupts the normal flow of that program's instructions.
A software program, regardless of the language it is written in, is meant to run a specific way and generate a certain outcome. However, if an unplanned event occurs during the program's execution, it can disrupt the normal flow of its instructions and result in an unexpected or undesirable output. This is called an exception. Generally, in computing and software engineering contexts, an exception occurs while a program is executing and disrupts the flow of its instructions.
The program must be able to handle exceptions so its current operation -- disrupted due to the exception -- can finish without further problems or errors. Almost all popular programming languages, including Java, C# and Python, support this ability, known as exception handling. C is among the few languages that does not support it, though it does offer many ways of error checking.
Checked and unchecked exceptions
Some programming languages like Java provide or recognize two types of exceptions: checked and unchecked.
Checked exceptions are those that must be handled (mandatory) and are caught at compile time. Usually, checked exceptions, also known as logical exceptions, are potentially recoverable because the compiler forces the user to handle it. If the exception is not handled, it will result in a compilation error.
Unchecked exceptions are runtime exceptions rather than compile time exceptions. It usually indicates that something has gone wrong with the program. These exceptions are often unrecoverable and can lead to serious problems and erroneous outcomes, including unexpected application stops or crashes. Errors are one type of unchecked exception.
Checked and unchecked exceptions are both types of built-in exceptions; that is, they are available in the libraries of various programming languages. Users can also create exceptions (user-defined exceptions) to convey situations that cannot be adequately described by built-in exceptions.
How exception handling works
Programs communicate with the operating system and other software systems through various layers.
In all programming languages with built-in exception handling capabilities, the process generally follows the same path.
When a checked exception occurs, the method where it happened creates an exception object that contains information about the exception, such as what type of exception it was and the state of the program when it happened. The creation and subsequent passing of this object to the runtime system is called throwing an exception.
Once the runtime system receives the exception object, it searches through the next layers, seeking exception handler code that matches what is specified in the exception object. Here, the system's goal is to find the code (or rather, the block of code) through an ordered list of methods that can handle the exception. The list is known as a call stack and the runtime system proceeds through it in the reverse order in which the methods were called (after looking at the method in which the error occurred).
If an exception handler that can handle the type of the exception object is found, the exception handler chosen is said to "catch" the exception. If the runtime system cannot find an appropriate exception handler even after searching through the entire call stack, the operating system generates a fatal exception message, which means that the program must close and perhaps the system must shut down as well.
Exception handling in Java
In Java, exceptions might occur due to invalid user input, device failure or coding errors. Out-of-disk memory, a user trying to open an available file and a loss of network connection might also throw an exception that disrupts the program.
Both checked and unchecked exceptions can occur in Java. Java programs can generally recover from checked exceptions, with exception handlers written into each program to define what it should do according to specified conditions. Examples of checked exceptions in Java include the following:
- ClassNotFoundException
- FileNotFoundException
- IOException
- SQLException
- RemoteException
- InterruptedException
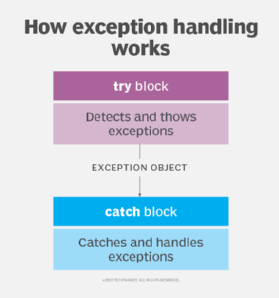
Users can handle checked exceptions in Java by wrapping the code that throws the exception within a "try catch" block or by using the keyword "throw" to throw a checked exception up the call stack for the calling method to handle.
In addition to try, catch and throw, Java provides some other keywords to handle checked exceptions. One is "finally," which is used to execute the program's necessary code, regardless of whether an exception is handled or not. Another is "throws," which is always used with method signature to declare a checked exception and specify that an exception might occur in the method.
Unchecked exceptions are less foreseen events that occur when a Java program runs. Common examples of unchecked exceptions in Java include the following:
- NullPointerException
- ArithmeticException
- ClassCastException
- ArrayStoreException
- IllegalThreadStateException
These exceptions -- and errors like stack overflows, infinite recursions or memory leaks -- can be handled at times, but usually they cannot. That's why it's important to identify, track and analyze errors in real time and before runtime. Ongoing error monitoring and triaging can help identify exceptions early, before they cause serious issues in the program.
Example of exception handler in Java
Here's a Java pseudocode example of an exception handler written for a previously declared exception type, EmptyLineException:
try {
line = console.readLine();
if (line.length() == 0) {
throw new EmptyLineException("The line read from console was empty!");
}
console.printLine("Hello %s!" % line);
console.printLine("The program ran successfully");
}
catch (EmptyLineException e) {
console.printLine("Hello!");
}
catch (Exception e) {
console.printLine("Error: " + e.message());
}
finally {
console.printLine("The program terminates now");
}
Exception handling in C#
Exceptions might occur in C# programs for many reasons: trying to connect to a nonexistent database, opening a corrupt file and so on. As with other languages like Java, the system raises an exception when it detects such events in a C# program. The exception is then handled by defining a block of exception handling code that will execute when an exception is thrown.
In this language, exceptions are usually generated in one of four ways:
- By common language runtime (CLR).
- By .NET libraries.
- By third-party libraries.
- By application code.
Exceptions are created by using the "throw" keyword and handled using the "try," "catch," and "finally" keywords. If an exception handler is not present for a particular exception, the program stops executing and displays an error message.
Three of the most common exceptions in C# are ArgumentException (a method argument receives an invalid argument value), ArgumentNullException (a non-allowed null value is passed to a method argument) and ArgumentOutOfRangeException (the argument's value is outside the allowable range).
Learn about the best practices behind exception handling for secure code design, including the process of throwing and handling different exceptions. See also: error handling