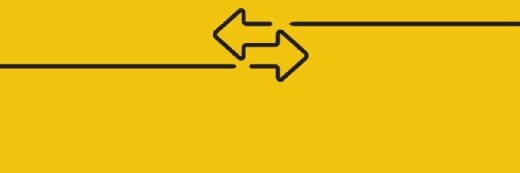
Either log or rethrow Java exceptions, but never do both
When an exception occurs in your Java code, you can log it or you can rethrow it -- but don't do both. Here's why you must avoid that exception handling antipattern.
Logging and exception handling are like two peas in a pod. When a problem happens in your Java code, that typically means you have an exception that needs to be handled, and of course, any time an error or unanticipated event occurs, that occurrence should be logged appropriately.
When performing exception handling in Java, there are really two options the developer has at their disposal:
- Handle the exception when it is thrown and recover from the error as it happens.
- Rethrow the exception so another part of the application can deal with the issue.
Log and rethrow exception example
In layered applications, option number two is particularly common, as there is typically a single layer at the top of the execution stack that is designed specifically for the task of handling and recovering from exceptions. It is very common to see layered applications rethrowing and rethrowing exceptions until they bubble up to the top. It can be a very effective strategy.
However, one of the most common mistakes developers make is logging the exception before it is rethrown. This practice must be regarded as an antipattern of the highest order.
/* log and rethrow exception example */ try { Class.forName("com.mcnz.Example"); } catch (ClassNotFoundException ex) { log.warning("Class was not found."); throw ex; }
Proper exception logging
There is no doubt that logging an exception in every catch block is done with good intentions, but doing so makes tracking down exceptions and resolving issues a complete nightmare.
The exception ends up getting logged multiple times in multiple layers. When quality engineers start tracking through the log files, the experience is suffocating, and troubleshooters have no idea where to start and when to finish.
The problem is significant, but it is easily resolved.
Exception handling best practices
The resolution to the log and rethrow antipattern?
Just get used to logging exceptions when, and only when, they are handled. That puts all exception logging in one place, in one file on one layer of the applications. It makes enterprise applications easier to troubleshoot, and the codebase easier to maintain.