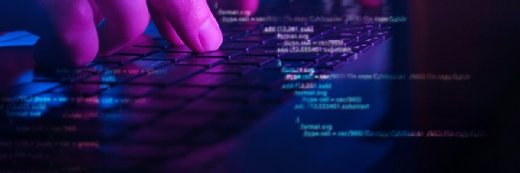
Getty Images/iStockphoto
What does the Python 'if name equals main' construct do?
Ever wonder what Python's if name equals main syntax does? Here we explore what it does and when to use it.
Python's if name equals main construct provides a standard entry point to run scripts and standalone apps.
Python has become a popular programming language due largely to its highly readable syntax and its comparatively forgiving compiler. This makes Python very approachable to new learners. However, as coders dig deeper into the APIs, they occasionally encounter unfamiliar syntaxes that initially seem confusing.
The if __name__ == "__main__": construct is one of these.
What does Python's 'if name equals main' construct do?
Python's if __name__ == "__main__": construct enables a single Python file to not only support reusable code and functions, but also contain executable code that will not explicitly run when a module is imported.
While not required, use of Python's name equals main syntax is considered a best practice, especially in applications that might contain multiple components or several files that contain runnable code.
Python's if __name__ == '__main__': in action
The exact syntax of Python's if name equals main construct, demonstrated in a simple Hello World program, is as follows:
if __name__ == "__main__":
print("Hello World")
If a file containing this code was run directly on the Python runtime, the code associated with the if condition would execute. If the file was imported as a module, the code would not run.
The if name equals main block guards the print statement, to ensure it executes only when the file is intentionally run directly as a script or application.
With the print statement positioned under the if name equals main block, the developer protects the print statement from being executed as unguarded, top-level code.
How does Python's __name__ variable work?
The __name__ variable is a special attribute managed by the Python runtime.
When a developer executes a Python program, the interpreter sets several variables, including __name__. There are two outcomes for the __name__ variable:
- If the script is run as a standalone application, __name__ is set to __main__.
- If the script is imported, __name__ is set to the name of the module.
Example of Python's __name__ variable in action
The following two Python files demonstrate how the __name__ variable differs when a file is executed directly or imported as a module.
The first file, named helper.py, simply prints out the __name__ variable. This Python script is not called directly, but rather imported by the app.py file.
print("__name__ in helper.py: " + __name__)
Next, we see the app.py file import the helper module and then print out its own __name__ variable:
import helper
print("__name__ in app.py: " + __name__)
When the app.py code runs, we see the __name__ variable is set to __main__ in app.py, but in the helper.py file, the variable is set to the name of the module: helper.
$ py app.py
__name__ in helper.py: helper
__name__ in app.py: __main__
Note that if the print statement in both these files were contained in an if name equals main block, the print statement in the helper.py file would be guarded and would not run. The output would simply be as follows:
__name__ in app.py: __main__
This is how Python knows whether to run the code inside the if name equals main block. If the name is set to main, it runs. If not, it doesn't.
Functions, methods and components
Here's a slightly more advanced Python script to demonstrate the use of the if name equals main construct:
def greet(name):
print(f"Hello, {name}!")
if __name__ == "__main__":
# This code will only be executed
# if the script is run as the main program
greet("Alice")
In this example, the greet() function takes a name parameter and prints a formatted message to the console.
The if __name__ == "__main__": statement checks if the script is being run as the main program. If it is, it calls the greet() function with the name "Alice".
If this script were imported as a module in another Python script, the greet() function would be available for use, but it would not execute automatically.
The code block following the if __name__ == "__main__": line only executes if the script is run as the main program. This allows for a clean separation of code that is meant for direct execution, and code intended for import.
When do I use Python's if __name__ == '__main__': construct?
A developer who writes Pythonic code must adhere to a set of best practices and conventions that make the code more efficient, readable and maintainable.
It's considered Pythonic to use the if name equals main construct because it promotes modularity and reusability. It allows your script to serve as both a standalone program and an importable module.
When not to use if name equals main
The if name equals main syntax is not required if all you want to do is run a Python script. New Python developers can code and explore a wide variety of AI, machine learning and statistics libraries and never encounter a need to use the construct.
The use of Python's if __name__ == "__main__": makes the most sense in the following three scenarios:
- You want to write code that executes only when the script is run as a main program and not when it is imported as a module.
- You want to include test routines that run independently to validate functions defined in the file.
- You want to include demonstration code that will not execute upon module import.
Embrace the Pythonic way
The if name equals main syntax might look somewhat esoteric at first. However, it is easily learned and its consistent syntax becomes familiar and less intimidating the more you work with Python.
Embrace the Pythonic way, and you'll become a more effective Python developer, a creator of efficient and versatile applications.
Remember, the key to success in Python lies in understanding and adhering to its principles and best practices. Continue to learn and apply these concepts to your coding journey.
Kevin McAleer has been in IT support and project management since 1998, with a keen interest in programming with Python. He also makes YouTube videos about building robots and bringing them to life with code.