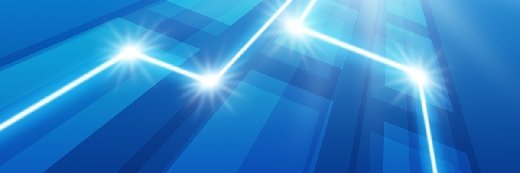
kiko - Fotolia
Why the 8 Java primitive data types are not objects
What's the difference between Java primitive types and objects? To start, don't classify primitive types as objects. Let's explore the 3.5 categories that primitive types fall into.
One of the tautological rules of Java programming is that everything in Java is an object except the things that aren't Java objects. The language defines eight Java primitive data types: boolean, float, double, byte, short, int, long and char. These eight Java primitive data types fall into the category of things that aren't objects.
In a Java program, data always manifests itself as one of the eight primitive data types.
Primitives simply represent a value, like the number seven or the boolean value of false. They have no methods and no complementary properties. Also, you can't do much with them other than inspect their value or perhaps change the value that they hold. But they are not, by any means, objects.
Java primitives vs. objects
Of course, Java is an object-oriented programming language, which means that developers can create all sorts of handsome assortments of these data types and organize them in logical and easily managed components. But even the most complex objects in Java can be condensed until all that's left is a rag-tag assortment of these eight primitive data types. Think of these eight Java primitive types as the atoms that form the base of everything that matters in a program.
The eight data types can be broken down into roughly three and a half separate categories. Yes, I said three and a half. I'll dive more into this logic in a bit.
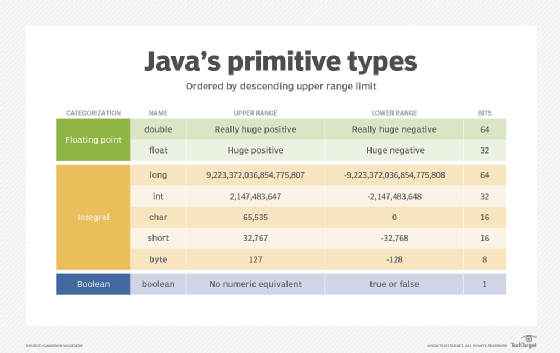
Category one: True or false values
The boolean primitive type is as simple as it gets. It can take on one of two literal values: true or false.
/* declare and initialize Java's boolean primitive type */ boolean colonel = true; boolean flag = false;
Key boolean type takeaway: In some languages, a boolean value can take on the value of a one or a zero -- but not in Java. A boolean must be initialized to either the literal value of true or the literal value of false -- not a one or a zero.
Category two: Decimal number types
The second category into which the primitive data types fall is the decimal or floating-point type. The float and double primitive types fall into this category, as they are able to take on fractional values.
/* how to declare and initialize Java floating point types */ float float f = 1.1F; double double d = 1.1D;
Key floating-point type takeaway: Double is both the larger and the default data type for floating-point values. Unless otherwise indicated, if the Java compiler sees a decimal, it views it as a double.
Category three: Signed whole numbers
Starting from the smallest to the largest, the following four primitives can be characterized as signed integral types: byte, short, int and long. The fact that these numbers are 'signed' simply means that they can take on both positive and negative values.
/* how to declare and initialize Java's integral types */ byte b = 127; short s = 32000; int x = 5; long l = 5L;
Key signed integral takeaway: Long wasn't part of the first incarnation of Java. By default, when the Java compiler sees a whole number, it assumes it is of type int -- not a long -- as one might otherwise expect.
Category 3.5: The weird type
The char primitive type is the really messed up one. Officially, char -- which is pronounced sometimes as 'char' like charcoal, 'car' or 'care' -- is an unsigned integral type that represents a positive number between zero and 65,535. Note that since the char is unsigned, it cannot take on any negative values: the char is all about positivity.
/* ways to declare and initialize the char primitive type */ char char t = 64000; char u = '1'; char x ='\u00A9';
If you think of char as a whole number type, it usually confuses people. A char typically represents an individual character, such as a letter in the alphabet or a punctuation symbol. That's the thing, a char represents a number, but that number is mapped back to a 16-bit Unicode character.
Technically, the char is an integral type, but in practice it is thought of as a representation of a single character. All of this makes the char rather quirky, which is exactly why I like to place it in its own half-baked category.
Java is indeed an object-oriented language. And when we build Java programs, the objects we create can be thought of as logical aggregations of commonly used values. At the lowest level, these values always break down into one of the eight Java primitive data types.