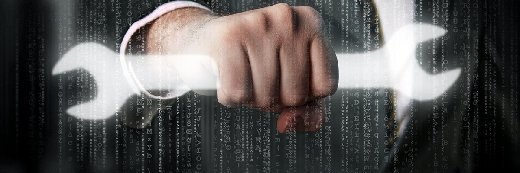
beawolf - Fotolia
Top Java programming tools used in application development
There's a multitude of Java tools that help the software development process. Here's a list of Java tools and technologies that every programmer should be aware of.
If you want to be a productive member of a software development team, it's important to master the key Java programming tools and technologies. These tools reach across a variety of areas, and developers face difficult decisions about which tools to choose because of the multitude of options.
Essential Java programming tools and technologies
In terms of what's essential for software developers to have installed on their local machine or perhaps even have access to in the cloud, the comprehensive Java programming tools list breaks down into the following 15 categories:
- A JDK distribution
- IDEs for Java
- Cloud-based Java IDEs
- Distributed and collaborative version control tools
- Code reconciliation tools
- Java build tools
- Java code quality tools
- Java testing tools and frameworks
- CI tools
- Java deployment tools
- Maven repository managers
- Java profiling tools
- Runtime Java reporting tools
- Virtualization and container tools for Java
- Miscellaneous tools that make your job easier
Choose your JDK distribution
The first thing you'll need for Java development is a JDK distribution. In the past, a JDK distribution simply meant you would agree to the Oracle license and download the software. The JDK landscape is much more complicated these days, with multiple vendor options available, such as the following:
- Amazon Corretto
- IBM
- Azul Zulu
- SAP
- Red Hat
- AdoptOpenJDK
These vendors compete over long-term support, upgrade and repair costs, scalability and license agreement flexibility. Without any specific requirements, an AdoptOpenJDK installation is probably the safest bet. If your company has chosen a different JDK implementation, it's best to use that for local development because production parity in your local environment is always the ultimate goal.
It's important to verify that your JDK installation was successful, and the best way to do so is to run a few of the command-line interface tools packaged in the bin directory of the JDK. Run the javac compiler to make sure you can turn source code into bytecode. Use the java utility to run a simple HelloWorld class. And familiarize yourself with other important JDK tools, such as JDeps and JStat, because they become important when you deal with garbage collection issues or internal API dependencies.
Java IDE considerations
After you install the JDK, the next step is to choose an IDE. In the Java world, there are three key players to choose from:
- IntelliJ from JetBrains
- NetBeans from Apache
- Eclipse IDE from the Eclipse Foundation
IntelliJ is the only option that isn't open source and open licensed. Even so, it tends to be the most popular IDE, which speaks volumes given that most Java developers tend to shun vendor-backed products in favor of open source options. If your organization offers an IntelliJ license, use it.
The choice between Eclipse and NetBeans can be trickier. A developer familiar with Eclipse will like the SpringSource Tool Suite (STS) for any Java Platform, Enterprise Edition or microservices development. STS is based on Eclipse, and it comes with a variety of preinstalled plugins that enable developers to be immediately productive. Someone with a long history with Eclipse will be biased toward that tool. Those more familiar with NetBeans would likely have similar opinions about the Apache option.
Cloud-based Java IDEs
It should be noted that a new trend in the world of Java programming tools is the emergence of cloud-based IDEs. They have been around for a long time, but network latency, limited feature set, and overall superior speed and ease of use of locally installed tools made cloud-based development a hard sell. However, modern networks are much faster and more reliable than in the past. This allowed cloud-based Java IDEs to become increasingly popular. Among the top options are the following:
- Codenvy
- Cloud9
- Eclipse Che
Java version control tools
Code isn't written in a vacuum. Software developers must share, integrate and merge their code with fellow programmers. Organizations need a distributed version control system that enables teams to maintain a history of changes, develop locally in isolated branches, merge code with other developers, and maintain a centralized repository to run builds and perform CI.
There are a number of choices in the source code management space, including Subversion, Microsoft's Team Foundation Server and IBM's ClearCase. For the most part, however, the industry trend is headed toward Git.
Created by Linus Torvalds in 2005, Git is maintained by The Linux Foundation. It can support large projects, but its feature set is intentionally limited to tasks directly related to versioning, sharing and merging source code. As such, most organizations will be interested in a cloud-based offering to simplify the use of Git and also to take advantage of additional features, such as online code edits, user management, branch protection and permissions. The most common competitors include the following:
- GitHub
- GitLab
- Atlassian Bitbucket
- Perforce
- CloudForge
GitHub stays true to the task of source code management in the cloud, and the UX tends to be straightforward. GitLab is similarly easy to use, but it goes beyond simple source code management and provides out-of-the-box connectivity with CI/CD tools. Likewise, Bitbucket is one part of Atlassian's tool suite, which includes Jira, Confluence and Crucible.
Code reconciliation tools
If you use Git, a good GUI tool to simplify Git resets, merges and rebases will save you time. Git comes packaged with a tool named Git GUI, which includes a nice feature set. Many developers tasked with code integration and merge-conflict fixes, however, swear by tools such as Sourcetree, GitKraken, TortoiseGit and Tower.
Java build tools
Just because code can be successfully merged into a Git repository without problems doesn't mean it can compile. After every merge, a compilation should occur, and that means a development team must choose a Java build tool to use. There are three main options in the Java build tool market:
- Apache Ant with Ivy
- Apache Maven
- Gradle
Apache Ant first rose to popularity at the turn of the century. While popular in the developer world, it has been largely supplanted by Apache Maven.
Like Ant, Apache Maven is an XML-based build technology with a rich ecosystem of plugins. Ironically, its XML-based lineage means Maven is less extensible than its Gradle counterpart, which includes tight integration with the Groovy programming language.
Apache Maven is a highly capable tool, but the trend on new projects is to adopt Gradle and embrace its Groovy-based API. On existing projects that already use Maven, however, the benefits of Gradle as a Java build tool aren't compelling enough that they'd justify a migration.
Java testing tools and frameworks
The build process typically follows two distinct stages. The first is to make sure the code compiles; the second is to make sure the code actually works. Code validation typically involves the use of Java testing tools and frameworks. For load, regression, unit, behavioral and integration tests, some of the popular Java programming tools to integrate into your build and development cycle include the following:
- JUnit or TestNG for unit testing;
- Mockito to create mock objects;
- Cucumber for behavioral testing and documentation;
- REST Assured and SoapUI for web service integration testing;
- Selenium and HtmlUnit for webpage testing; and
- Apache JMeter for load testing and to identify performance bottlenecks.
Java code quality tools
Every developer strives to write bug-free code, but tight deadlines and short Agile sprints can strain the best intentions. Fortunately, there are a variety of Java static code analysis programs that will help maintain high standards for code quality, even when the pressure to deliver the next release mounts. There is no reason for a software developer not to include a call to each of these open source Java code quality tools in every build:
- JaCoCo or Cobertura for code coverage;
- Checkstyle for style guide compliance;
- PMD for source code analysis;
- FindBugs for bytecode analysis; and
- SonarQube for evaluating code on seven separate axes of quality.
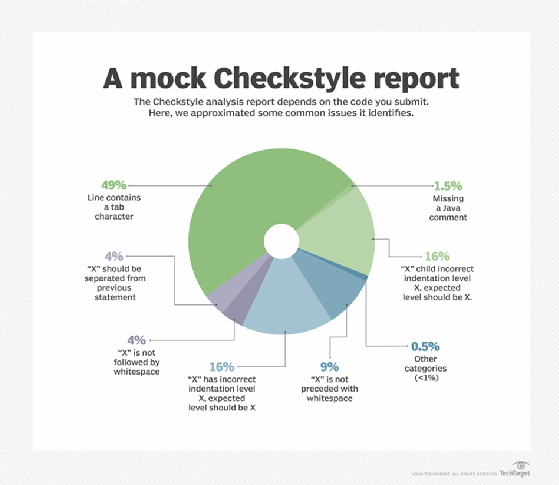
This isn't a comprehensive list of available options either. There are many vendor offerings in the Java static code analysis tools space, such as Coverity, Fortify, IBM AppScan, Klocwork and Veracode.
CI tools for Java programmers
It's a tedious process to perform a Git pull, make sure the code merges successfully, perform a compilation and run a suite of unit tests. That complexity makes CI products especially useful.
There are a number of players in the CI/CD field, including the following:
- Atlassian Bamboo
- GitLab CI
- Travis CI
- JetBrains TeamCity
- Concourse CI
- Codeship
- Jenkins CI.
The primary job of a CI tool is to orchestrate interactions with other Java programming tools. For example, a sample Jenkins build job might pull from a GitHub repository, compile code with Gradle, run a suite of unit tests and push code to a Maven repository.
As such, many of the vendors that have a presence in any of those other Java programming tool categories also offer CI tools. It's no coincidence that the likes of Atlassian, GitLab and JetBrains show up in multiple categories. These vendors believe a suite of tools under the same umbrella that covers many aspects of the software development lifecycle gives them an advantage over tools that are singularly focused.
The sample suite: A possible Java programming tools list | |
Purpose | Product |
JDK distribution | AdoptOpenJDK |
Java IDE | STS |
Cloud IDE | Codenvy |
Version control | Git |
Code history tool | GitKraken |
Code quality tool | SonarQube |
Java build tool | Maven |
Testing tool | Cucumber |
CI/CD tooling | Jenkins CI |
Deployment tool | GitLab |
Maven repo | JFrog Artifactory |
Java profiling tool | Java Mission Control |
Application performance management tool | AppDynamics |
Container tool | Docker |
Simple utilities | Fiddler |
Java deployment tools
Application deployment is obviously an important part of software development. Ironically, it is also a somewhat difficult term to precisely nail down. After all, what exactly does deployment mean?
In the classical sense, deployment would involve packaging an application and pushing it to a production server. But no enterprise organization deploys directly into production without a variety of stages first, including development stage deployment, UX testing deployment, preproduction hardening and, finally, a production push. So, which part of that process would you consider the deployment?
Furthermore, the live production server is actually not the typical deployment target for the software developer's write. More often than not, Java developers work on modular code libraries, which get pushed to an artifact repository, and that artifact repository is the final destination. Other programs may link to that code and include it as part of an application that gets deployed to a production server, but that deployment is a completely separate process.
Several of the aforementioned build tools , either on their own or with the help of a plugin or two, will enable users to stage code, push to artifact repositories and deploy applications to a production server, such as IBM's WebSphere or Red Hat's JBoss server.
Maven, Gradle, Jenkins CI, Atlassian Bamboo and GitLab can perform these deployment tasks. It's also worth mentioning that CA Technologies' Release Automation tool performs release management, and its Qubeship product helps with Docker-based microservices deployments to Kubernetes and OpenShift-based systems.
Maven repository manager for artifact hosting
The final target of deployment for most Java development isn't a live production server, but instead an artifact repository. The two key players in Java repository management are JFrog Artifactory and Sonatype Nexus.
Traditionally, we refer to these products as Maven repositories, because the artifacts deployed were mapped with Maven's GAV (group, artifact, version) syntax, and it was typically Maven build jobs that pulled those artifacts onto a developer's local machine when needed. However, Artifactory and Nexus are no longer exclusively tied to Java code and Maven builds. These artifact management tools now have the capacity to act as repositories for Docker images, JavaScript libraries and any other type of binary resource you can think of.
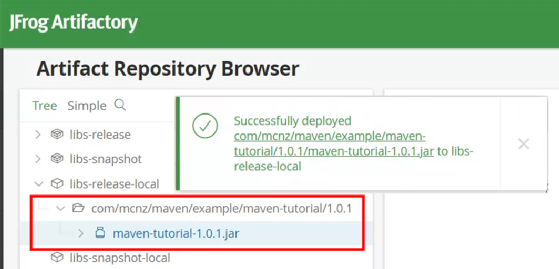
Java profiling tools
As applications grow larger, troubleshooting becomes more difficult. Hence, developers need to become comfortable with tools that can profile the application as it runs and identify Java instances and methods that slow down the application or even cause it to crash.
A developer really needs to learn only one Java profiling tool because they all tend to perform the same basic tasks. Some of the most popular Java profiling tools include the following:
- Java Mission Control
- JProfiler
- VisualVM
- YourKit
It should also be noted that the JDK tool JStat is packaged with every Java installation and is an effective way to garner metrics about garbage collection and memory consumption. Furthermore, Memory Analyzer for Eclipse can be integrated directly into the IDE; it enables programmers to do quick, local analysis of their Java applications as code is developed.
Runtime Java reporting tools
A step beyond a simple profile of the Java virtual machine is Java application performance management tools that provide full reports of how the application behaves on each request that goes through the system.
These Java reporting tools provide exceptional detail in terms of which parts of the application path act as bottlenecks and which objects consume too much memory when created. Runtime Java reporting tools are essential to limit trouble with application performance. Some options include the following:
- AppDynamics
- Dynatrace
- New Relic
- SiteScope
- AutoPilot
Virtualization and container tools for Java
In this world of virtualization and microservices development, you will need access to various virtualization tools. These will let you run applications on OSes other than your own or to perform a deployment to a container-based platform, such as Kubernetes or OpenShift. At the very least, a Java developer should have a local installation of VMware or VirtualBox, along with a Docker or Kubernetes installation to test microservices.
Simple tools for Java developers
There are also a variety of tools that may not be specific to Java, but can certainly make your life easier, such as the following:
- Fiddler, as a web proxy to help troubleshoot your applications;
- WinMerge or Beyond Compare to figure out what has changed between two versions of a file;
- Notepad++ when you want to quickly inspect a text-based document;
- XMLSpy to quickly inspect XML files;
- Toad to inspect your Java Database Connectivity and Java Persistence API mappings;
- PuTTY to connect to your remove servers and AWS instances;
- FileZilla to move FTP files from one server to the next; and
- JS-Beautify to clean up how your code is formatted and spaced.
The right Java tools and technology
The Java ecosystem is filled with both vendors and open source contributors that make it difficult to put together a list of Java programming tools and technologies without feeling that many worthy vendors and projects have been left out. But, overall, this Java programming tools list should give you a reasonable overview and help you to become a productive programmer.