Build Java SOAP web services in Eclipse with Jakarta EE
In a world of microservices development and Docker-based deployments, RESTful web services tend to grab all the headlines. However, there's still a place in modern enterprise architectures for SOAP-based web services that exchange data in a highly structured XML format. This tutorial shows you how to build them in Java using the Jakarta EE specification.
Java, SOAP, Jakarta EE and Eclipse
The easiest way to build SOAP-based web services is to follow these seven steps:
- Create a Jakarta EE 10 Maven project with the Eclipse Foundation's online starter tool.
- Import the created Maven project into Eclipse.
- Implement your domain model in Java and decorate your POJOs with XML annotations.
- Create a service class with public methods that will act as http endpoints.
- Annotate your service class with @WebService and @WebMethod annotations.
- Deploy to a modern Java application server such as Payara or Wildfly.
- Test your web service with a tool such as SoapUI.
Jakarta EE project initializer
In the past, wizards to build a SOAP-based web service were packaged inside the Eclipse IDE. Today, the recommended way to create an enterprise Java project for Eclipse with the latest Jakarta EE APIs is to use the Eclipse Foundation's online Starter for Jakarta EE.
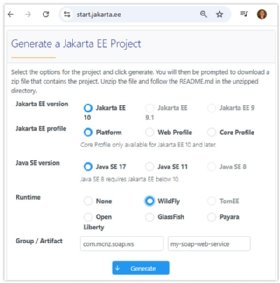
As with Spring's Initializer tool, this Eclipse starter tool will help you create a basic Jakarta EE application with all of the required dependencies preconfigured. Just import the zip file the tool provides into Eclipse, and you'll be ready to start development.
Build your domain model in Java
Jakarta EE promises developers a minimally invasive development experience in which programmers can concentrate on their business logic, while the framework largely supports services including SOAP and REST. This means developers can build JavaBeans and POJOs as they normally would.
For this example, we create a Score class to support a hypothetical Rock, Paper, Scissors application. The score has three properties that represent wins, losses and ties. Note, however, that the class also includes a few annotations that enable the Jakarta EE framework to know that the properties eventually must be marshalled across the network in an XML format.
@XmlType
@XmlAccessorType(XmlAccessType.FIELD)
public class Score {
int wins;
int losses;
int ties;
}
Code the service class
To support SOAP-based web service calls, a developer must build a service class decorated with Jakarta EE @Stateless and @WebService annotations. Optionally, methods can be annotated with @WebMethod to provide the underlying framework with more information on how to configure the SOAP service.
In our example we'll provide two SOAP-based endpoints: one to retrieve the current score and another to update it.
@Stateless
@WebService
public class ScoreService {
public static Score score = new Score();
@WebMethod(operationName="getTheScore")
public Score getScore() {
return score;
}
public Score updateScore(int w, int l, int t) {
score.wins = w;
score.ties = t;
score.losses = l;
return score;
}
}
Deploy and query the WSDL file
With the service class and the business logic completed, simply run your application on an application server configured within your Eclipse environment. In this tutorial we use Wildfly, but any Jakarta EE compliant server will do. Note that Tomcat will not suffice as it only supports the Jakarta EE web profile.
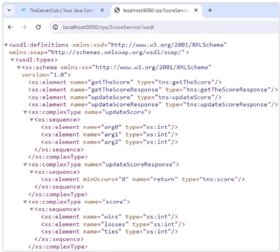
As an initial smoke test, try to view your application's web service definition language (WSDL) file in a web browser. The following image shows the WSDL for the ScoreService, which includes references to the updateScore and getTheScore methods.
Be sure to use a question mark in the URL. A developer's muscle memory often leads them to put .wsdl instead of ?wsdl, which will cause an error. Be careful when typing in the URL.
Test the SOAP web service
With your Java-based SOAP web service deployed, it's time to test it.
Simply paste the URL that points to your public WSDL file into a tool such as SoapUI and test the various endpoints. You can test both how it updates and gets the score from the server. The SoapUI tool shows both the XML sent out to the client, and the XML received when the SOAP-based request is completed.
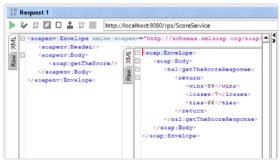
Top-down vs. bottom-up SOAP web services
This example's approach to building a Java-based SOAP web service in Eclipse takes a bottom-up approach to the problem. That means we first start with the code and then use the tools to create the WSDL and deploy the service.
In contrast, Spring Boot's SOAP web service approach is entirely top-down. You must first provide the WSDL and the Spring framework and then create the set of skeleton classes required by your web service.
If you're starting off with a WSDL file, and you haven't committed to Jakarta EE or Spring Boot for development, it's worth looking at Spring's approach to SOAP web services. If you prefer to take a bottom-up approach, Jakarta EE is the way to go.
Cameron McKenzie has been a Java EE software engineer for 20 years. His current specialties include Agile development; DevOps; Spring; and container-based technologies such as Docker, Swarm and Kubernetes.