Step-by-step RESTful web service example in Java using Eclipse and TomEE Plus
TheServerSide has published a number of articles on the tenets of effective RESTful web service design, along with examples of how to actually create a cloud-native application using Spring Boot and Spring Data APIs. In this JAX-RS tutorial, we will go back to basics by developing the exact same application, except this time we'll use standard Java EE APIs and the extended, enterprise version of Tomcat, TomEE Plus, as our deployment target. This step-by-step JAX-RS RESTful web service example in Java using Eclipse and TomEE Plus will get you up to speed on modern web service development techniques in less than 15 minutes.
Prerequisites
This tutorial uses Eclipse Oxygen as the development environment, the underlying JDK is at version 1.8, and the deployment target is TomEE Plus. You can download TomEE Plus from the project's Apache home page.
Why are we using TomEE Plus, and not Tomcat or the standard TomEE offering? Sadly, the basic Java web profile, which Tomcat 9 implements, does not support JAX-RS, it does not include the javax.ws.rs.* packages, and without playing with POM files or adding JAR files to the Eclipse project's \lib directory, RESTful web services simply won't work. The standard TomEE offering doesn't include JAX-RS libraries either. On the other hand, the TomEE Plus server does include various enterprise packages, including JAX-RS, so RESTful web services will deploy right out of the box, making this RESTful web services example much simpler.
Step 1: The dynamic web project
The first step in this JAX-RS tutorial is to kick off the dynamic web project creation wizard in Eclipse.
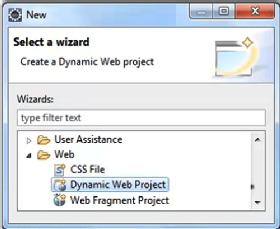
When the dynamic web project wizard appears, name the project restful-java, choose Apache Tomcat 8.5 as the target runtime (even though we are using TomEE Plus, not Tomcat), specify 3.1 as the dynamic web module version and choose a minimal configuration for the project. When these options are set, click Finish.
Note that you need to install TomEE Plus prior to doing this JAX-RS tutorial. You can also use any other application server that supports Java EE and JAX-RS for this RESTful web service example in Java using Eclipse.
Where is the web.xml file?
If you look at this project in GitHub (link below), you'll notice that there isn't a web.xml file. That makes traditional enterprise developers nervous, but so long as everything is annotated, there's no need for one in version 3.x of the Servlet and JSP spec. In older REST implementations you would need to configure a Jersey Servlet and perform a REST Servlet mapping, but that is no longer necessary. In this case, TomEE Plus will process all of the annotations on the classes in the Java web project and make RESTful web sevices available accordingly. It should be noted that on some servers, you do need to reference your JAX-RS classes explicility, which you can do through an Application class. That process is addressed in the JAX-RS problems section towards the end.
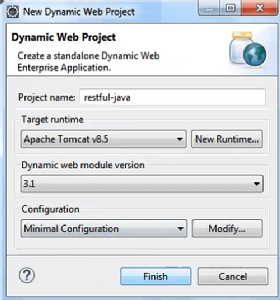
Step 2: Create the Score class
This restful web service example in Java using Eclipse models a score counter for an online rock-paper-scissors application, so the first requirement is to create a class named Score that keeps track of wins, losses and ties.
package com.mcnz.restful.java.example;
public class Score {
public static int WINS, LOSSES, TIES;
}
To keep things simple, we won't add any setters or getters. Furthermore, we are going to make the properties of the Score class static, as that will enable the Java virtual machine (JVM) to simulate persistence between stateless calls to the web service. This approach will enable us to run and test the application on a single JVM. However, you should manage application state in this way only as a proof of concept. It's better to persist data with Hibernate and Java Persistence API or save information to a NoSQL database, but that is beyond the scope of this JAX-RS tutorial.
Step 3: Code the JAX-RS Service class
A class named ScoreService is the heart and soul of this RESTful web service example in Java using Eclipse. As such, decorate it with an ApplicationPath annotation that defines the base URL of the web service.
package com.mcnz.restful.java.example;
import javax.ws.rs.*;
@ApplicationPath("/")
public class ScoreService { }
This class will contain three getter methods that enable RESTful web clients to query the number of wins, losses or ties. These methods are invoked through an HTTP GET invocation and return the current win, loss or tie count as plain text. As such, these methods each have a JAX-RS @GET annotation, a @Produces annotation indicating they return a text string and a @Path annotation indicating the URL clients need to use in order to invoke the method:
@GET @Path("/score/wins")@Produces("text/plain")
public int getWins() {return Score.WINS;}
@GET @Path("/score/losses")@Produces("text/plain")
public int getLosses() {return Score.LOSSES;}
@GET @Path("/score/ties")@Produces("text/plain")
public int getTies() {return Score.TIES;}
The increase methods of this JAX-RS tutorial's ScoreService follow a similar pattern, with the exception of the fact that each method is triggered through an HTTP POST invocation:
@POST @Path("/score/wins")@Produces("text/plain")
public int increaseWins() { return Score.WINS++; }
@POST @Path("/score/ties")@Produces("text/plain")
public int increaseTies() { return Score.WINS++;}
@POST @Path("/score/losses")@Produces("text/plain")
public int increaseLosses() {return Score.LOSSES++;}
The final two methods of the ScoreService class enable users to get the JSON-based representation of the complete score or pass query parameters to the web service to update the static properties of the Score class. Both methods use the /score path, and both produce JSON. But the getScore method is invoked through an HTTP GET request, while the update method is invoked through a PUT.
Just for the record, there is an easier way to return JSON from a RESTful web service than by using the String.format call. You can use @Producer annotations and simply return JavaBeans, but because we are using static variables in our Score class, doing that gets a bit messy. We will save that for a future RESTful web services tutorial with Eclipse.
@GET
@Path("/score")
@Produces("application/json")
public String getScore() {
String pattern =
"{ \"wins\":\"%s\", \"losses\":\"%s\", \"ties\": \"%s\"}";
return String.format(pattern, Score.WINS, Score.LOSSES, Score.TIES );
}
@PUT
@Path("/score")
@Produces("application/json")
public String update(@QueryParam("wins") int wins,
@QueryParam("losses") int losses,
@QueryParam("ties") int ties) {
Score.WINS = wins;
Score.TIES = ties;
Score.LOSSES = losses;
String pattern =
"{ \"wins\":\"%s\", \"losses\":\"%s\", \"ties\": \"%s\"}";
return String.format(pattern, Score.WINS, Score.LOSSES, Score.TIES );
}
Step 4: Deploy the JAX-RS web service
Now that you've coded the JAX-RS tutorial's ScoreService, it's time for this RESTful web service example in Java using Eclipse to move into the testing stage. Remember that we are using TomEE Plus as our target server, not Tomcat. Tomcat doesn't provide built in JAX-RS support.
To test the application, first right-click on the restful Java project, and choose Run As > Run on server. This will deploy the web project and start the Apache TomEE Plus server that hosts the application.
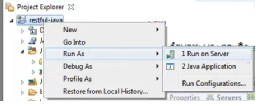
Step 5: Test the JAX-RS web service example
When you deploy the JAX-RS tutorial app, there are a number of different ways to test it. One way is to simply type the URL of the RESTful web service example into a web browser. A call to the following URL will trigger a GET invocation and a JSON string representing the initial score should be displayed:
http://localhost:8080/restful-java/score
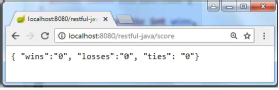
To test the increaseTies method, run the following two curl commands in a Bash shell:
$ curl -X POST "http://localhost:8080/restful-java/score/ties"
$ curl -X GET "http://localhost:8080/restful-java/score/"
The JSON string returned from the second command indicates that the number of ties has indeed been incremented by one:
{ "wins":"0", "losses":"0", "ties": "1"}
Now, use curl to trigger a PUT invocation with query parameters:
$ curl -X PUT "http://localhost:8080/restful-java/score?wins=1&losses=2&ties=3"
This PUT invocation will return the following JSON string:
{ "wins":"1", "losses":"2", "ties": "3"}
Fixing common JAX-RS problems
In this example, the ScoreService class is annotated with @ApplicationPath. This works fine with TomEE Plus, but on other servers or older implementations, the @ApplicationPath annotation is placed on a separate class that extends the JAX-RS Application class. This often solves the problem of RESTful URLs simply not being recognized and triggering a 404: The origin server did not find a current representation for the target resource error when an attempt is made to invoke them.
import javax.ws.rs.core.Application;
@ApplicationPath("/")
public class ScoreApplication extends Application {
public Set<Class<?>> getClasses() { return new
HashSet<Class<?>>(Arrays.asList(ScoreService.class));
}
}
On servers where the implementation is Jersey based, the class can be replaced with one that is a bit easier to understand, although it calls on Jersey APIs explicitly, so it will only work with a Jersey based implementation. You just tell it the names of the various packages where JAX-RS annotated web services reside, and it ensures they are loaded:
import javax.ws.rs.ApplicationPath;
import org.glassfish.jersey.server.ResourceConfig;
@ApplicationPath("/")
public class ScoreApplication extends ResourceConfig {
public ScoreApplication() {
packages("com.mcnz.restful.java.example");
}
}
And of course, you must ensure you are using TomEE Plus and not Tomcat. As was mentioned earlier, a standard Tomcat installation will not run RESTful web services without a JAX-RS implementation added to the \lib directory, Gradle build script or Maven POM.
And that's a complete, step-by-step JAX-RS RESTful web service example in Java using Eclipse and TomEE Plus.
The full source code for this example can be downloaded from GitHub.
Create a JAX-RS web service with Tomcat, Eclipse
If you used TomEE in an attempt to create a JAX-RS web service and ran into issues, watch this new video that instead uses Tomcat and Eclipse to create this RESTful web service.