How to use JShell in Java
Java and JShell
Java 9 introduced JShell, a read-evaluate-print and loop (REPL) tool that greatly simplifies Java development.
However, despite the great utility JShell provides to Java devs, it has not seen widespread adoption, and most Java developers are unaware of its capabilities. To remedy that situation, here’s a look at five impressive JShell benefits and features that will get the uninitiated wanting to use it.
- You don’t need to compile your Java code.
- Java programs will run without a main method.
- Semicolons can be optional.
- Hundreds of extra classes are imported automatically.
- Exception handling is optional.
No need for javac
With typical, standalone Java programs, developer must compile source code into bytecode with the javac utility, and then run that bytecode on the JVM. That’s not necessary with JShell.
JShell reads your source code and runs it on the fly. Your code runs immediately. There’s no needless suspense to see if your program works or not.
In fact, you don’t even have to code your Java into a file. JShell can execute lines of Java code directly on the command line.
Java without a main method
Historically, Java devs have had to code a main method every time they want to run a tiny snippet of code, a tedious and annoying requirement.
IDEs such as Eclipse and IntelliJ have scrapbook pages where developers can test a couple of lines of code, but those are more of a Java hack than a standard feature.
However, with JShell you can run a full Java program without a main method. Just code your logic into a file and run it at the command line:
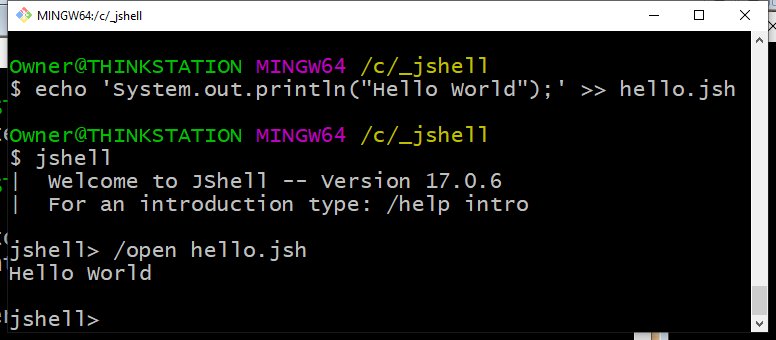
You can run a Java program without a main method in JShell.
Goodbye to superfluous imports
The java.lang package imports automatically into your standalone Java programs, but even the most basic programs typically require java.util or java.io. As a result, import statements clutter the start of your files.
With JShell, the following packages are imported automatically, which means you can reference hundreds of additional Java classes without the need to organize imports.
JShell imports the following packages by default:
- io.*
- math.*
- net.*
- nio.file.*
- util.*
- util.concurrent.*
- util.function.*
- util.prefs.*
- util.regex.*
- util.stream.*
Java without semi-colons?
The software development community has drawn battle lines between languages that require semicolons at the end of the statement and languages that don’t. With JShell, Java doesn’t require semicolons.
Much to the chagrin of traditional Java developers, the following line of code executes successfully in a JShell script:
System.out.println(“Hello JShell World.”)
Furthermore, adding a semicolon to the end won’t cause a problem.
There are limitations to this neat trick.
When you enter multiple statements on a single line, you need to use semicolons. Also, logic within loops and conditional logic blocks require semicolons. Otherwise, feel free to drop the semicolons and pretend for a minute that you’re programming in JavaScript.
No more Java exception handling
Java’s verbosity is a common criticism that proponents of languages including JavaScript and Python often level at Java programmers. The semantics of Java exception handling provides plenty of fodder for such people.
In JShell, exceptions need not be handled.
For example, to output the content of a file in a regular Java program, you must wrap one line of code in an additional three more lines to complete the try…catch exception handling semantics:
try { Files.lines(Paths.get("example.jsh")).forEach(System.out::println); } catch (IOException ex) { ex.printStackTrace();//handle exception here }
To do that in JShell? It’s just one line of code:
Files.lines(Paths.get("example.jsh")).forEach(System.out::println);
More Java in JShell features
These five features of JShell in Java are just the tip of the iceberg.
Developers that dig deeper into the JDK’s new REPL tool will discover other compelling JShell features, including:
- auto code-completion at the command line;
- a navigable history of previously written code;
- automatic variable declaration;
- command-line editing;
- multiple expressions per line; and
- full scripting support.
If you haven’t already checked out JShell in Java, you really should. It will change the way you think about your code.