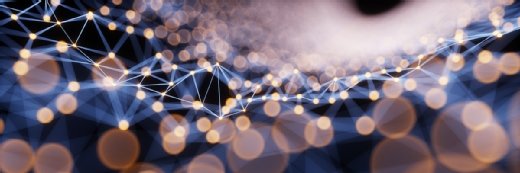
Sergey - stock.adobe.com
Five RESTful web service client examples for developers
Explore your web service client invocation options with these five examples that enable developers to perform this task at any stage of development.
How do you access a RESTful web service? That depends on what you're trying to accomplish.
If you just want to test connectivity, a terminal-based utility like curl is a great RESTful web service client. If you want to inspect the JSON a service returns to you, a browser-based plugin will probably be a better fit. And if you are in the midst of application development, you'll likely need to use JAX-RS, Spring or a similar framework.
RESTful web service clients come in a variety of shapes and sizes. Here are the five that every Java developer should know.
Curl
Curl is a Unix-based utility that enables developers to invoke URLs from a command line to generate information about the results. The results include header data, XML, JSON and various other parameters and they can be rendered as plain text in the command window. Linux users tend to be well acquainted with curl because it is commonly included in most distributions.
On the flip side, curl isn't included as part of the Windows OS, so Microsoft users tend to be less comfortable curling URLs. However, default Git installations always include Bash to issue distributed version control system commands. As more and more Windows users adopt Git and GitHub, more developers will have the curl command at their immediate disposal.
The syntax to use curl as a RESTful web service client for a simple GET request is:
$ curl -X GET --header "text:Easter" --header "language:fr" http://3.19.68.127/translator-1.0/translate
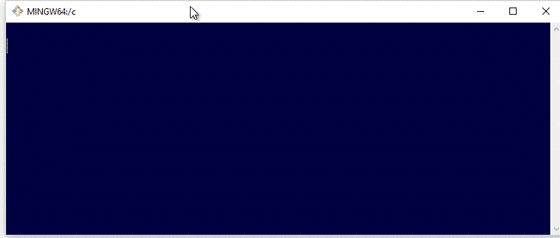
RESTful jQuery client
If you want to access a RESTful web service from a browser-based application, you'll likely want to use a JavaScript framework. All of the popular JavaScript frameworks and libraries, such as AngularJS, Ember.js, React and jQuery, provide capabilities that can simplify REST-based interactions.
Here is an example of a simple RESTful web service client written in jQuery:
<html><head>
<script src="jquery.min.js"></script>
</head>
<body>
<div id="rest-web-service-client"></div>
<script>
$(document).ready(function() {
$.ajax({
url: "http://3.19.68.127/translator-1.0/translate",
headers: {"language":"fr", "text":"rabbit"}
}).then(function(data) {
alert(data); // will display 'lapin'
});
});
</script>
</body>
</html>
Java-based REST client
REST-based services follow all of the semantics of HTTP, so they can be accessed via the networking and I/O packages that come standard with every JDK installation. More often than not, developers will use a web development framework such as Jakarta EE or Spring Boot to access a remote API, as both of these frameworks have built-in libraries to write RESTful web service clients in Java.
But this level of extravagance isn't required. Below is an example of how you can access a RESTful web service without a framework like Spring. This example uses nothing more than the java.net and java.io packages.
Socket requestSocket = new Socket("http://mcnz.com", 8080);
PrintWriter writer = new PrintWriter(requestSocket.getOutputStream(),true);
writer.write("GET language-translator/api");
writer.flush();
while(true) {
int x = requestSocket.getInputStream().read();
if(x==-1) {
break;
}
}
writer.close();
requestSocket.close();
RESTful web service frameworks
All of the popular libraries for developing enterprise applications and microservices come with the ability to write RESTful web service clients. Spring Boot, Jersey and JAX-RS all differ slightly in terms of APIs, but they are similar enough for developers to easily transition from one to another.
Here is a snippet of code that shows how to create a RESTful web client with Spring and its RestTemplate:
HttpHeaders headers = new HttpHeaders();
applyAPIKeyToHeader(key, headers);
headers.setContentType(MediaType.APPLICATION_JSON);
String data = getTextToTranslate(textToTranslate, sourceLanguage, destinationLanguage);
HttpEntity<String> request = new HttpEntity<String>(data, headers);
String url = "www.mcnz.com";
ResponseEntity<String> response = restTemplate.postForEntity(url, request, String.class);
String responseBody = response.getBody();
translationResult = objectMapper.readValue(responseBody, TranslationsResult.class);
Chrome extensions for REST
If you don't have access to curl and you don't intend to code a RESTful web service client, you've always got the option of installing a Chrome or Firefox extension that will invoke a REST-based service. Postman is one of the many extension available.
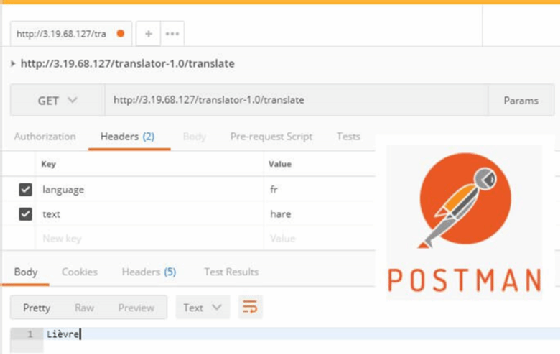
RESTful web services are incredibly popular, especially as the microservices trend takes hold. But every REST service needs a client. There are a variety of ways to create RESTful web service clients for testing, validation and application integration.