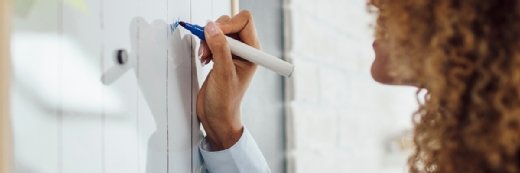
Getty Images
Java naming conventions, explained
You could start a Java variable with a dollar sign, but no one does. When you write Java code, always follow these standard Java naming conventions.
The rules for Java variable naming are fairly lax.
The first letter of a variable must be either a letter, dollar sign or an underscore. After that, any combination of valid Unicode characters or digits is allowed. It's not very restrictive.
But rules, on the other hand, are different from conventions. Java naming conventions for variables, methods and reference types are where things get a little more complicated.
For example, you can start a Java variable with a dollar sign or an underscore, but nobody does. Sometimes machine-generated code will prepend a dollar sign or underscore a Java variable to emphasize the non-human origins, but that's about it.
Java variable naming conventions
For variables, the Java naming convention is to always start with a lowercase letter and then capitalize the first letter of every subsequent word. Variables in Java are not allowed to contain white space, so variables made from compound words are to be written with a lower camel case syntax.
Here are three examples of variables that follow the standard Java naming convention:
- firstName
- timeToFirstLoad
- index
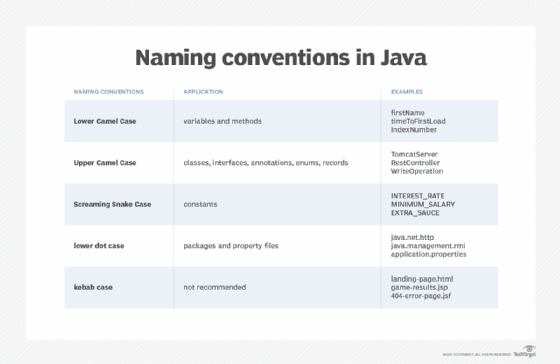
Java method naming
Lower camel case, also known as dromedary case, is also the Java naming convention for methods. Here are three examples of properly named Java methods from the String class:
- compareToIgnoreCase(String str)
- copyValueOf(char[] data)
- equalsIgnoreCase(String anotherString)
The only exception to the lower camel case rule is for variables with a constant value. Any variable decorated with a static final keyword combination should be written in screaming snake case. That is, all letters are uppercase, and compound words are separated by an underscore.
Here are three examples of constants defined within the Spring Boot API:
- NESTED_PROPERTY_SEPARATOR
- DEFAULT_TASK_EXECUTOR_BEAN_NAME
- GLOBAL_SUFFIX
Java reference type names
There are five reference types in Java: classes, interfaces, annotations, enums and the recently added record. The standard Java naming convention demands that all reference types be written in PascalCase, also known as upper camel case.
Here are three examples of reference types defined within the JAX-RS API for building RESTful web services:
- QueryParam
- ServerErrorException
- RuntimeType
Java package names
The convention for packages is different from the Java naming conventions used for variables, methods and reference types. Packages are always written in lowercase letters, with a dot between words. A Java package is actually a reference to the file system. The dot in a package name maps to the set of folders and subfolders in which a given Java class or interface resides.
An example of three package that are part of a standard JDK installation include:
- net.http
- management.rmi
- transaction.xa
Java and kebab case
There is no artifact in the programming language in which kebab case is recommended. The dash in a kebab-cased variable can often be confused with a minus sign, which can make reading and troubleshooting difficult. The Java naming conventions have decided to steer clear of any kebab case controversy. Having a dash in a variable, method or reference type in Java is not recommended.
Review the most popular variable naming conventions.
By following Java naming conventions, developers make their source more readable, as seasoned developers can infer details about the code simply by looking at how variables are cased. When you write Java code, always follow the standard Java naming conventions. It helps ideas flow faster and more naturally between developers.