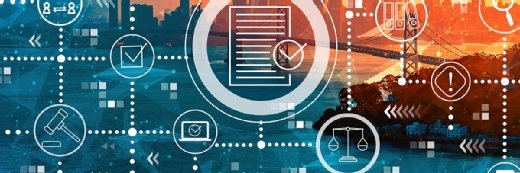
Getty Images/iStockphoto
3 simple Java Hello World examples
Are you a developer who's new to Java? Here are three ways to write a Hello World program in Java on Eclipse and get started with the popular programming language.
There's a lot of excitement in the Java community regarding the latest long-term support JDK release. When that release debuts, Java developers will be welcomed with the familiar Hello World program to get started with the latest distribution.
Here are three Java Hello World examples developers can use to get started on the new release:
- Hello World with a console output
- Hello World with a Java Swing component
- Hello World with a static code block
3 Java Hello World examples
The standard Hello World program in Java writes output to the console window. It's a boring and banal introduction to Java, but it demonstrates the basic syntax, gets developers familiar with curly braces in Java and most importantly, it works.
package com.example.helloworld.java; public class HelloWorld { public static void main(String args[]) { System.out.print( "Hello World!" ); } }
A Hello World program with Java Swing
Visual learners prefer to interact with GUI components as opposed to a terminal window or the command line. A Java Swing-based Hello World program, which uses Java's desktop development APIs, is a nice departure from console-based programming.
One of the goals of a Hello World program is to write the most basic program possible. However, a Hello World program with a Java Swing component requires programmers to add a package statement. While it's not the simplest Java program you could possibly write, it's worth the extra few keystrokes to remind developers that Java is used for more than just Android apps and cloud-native microservices development.
package com.example.helloworld.swing; import javax.swing.*; public class HelloWorld { public static void main(String args[]) { JOptionPane.showMessageDialog( "Hello World!" ); } }
The static Java Hello World program
Here's a way to write a Hello World program in Java without adding any code to Java's main method. Instead, you write your Hello World code in a static code block.
package com.example.helloworld.static; public class HelloWorld { static { System.out.print( "Hello World!" ); } public static void main(String args[]) { } }
Prior to the JDK 1.7 release, developers could completely remove the main method and the static code block would run before the system sent the "no main method was found" error message. If you work with an older version of the JVM, give it a try. Otherwise, the main method is required.
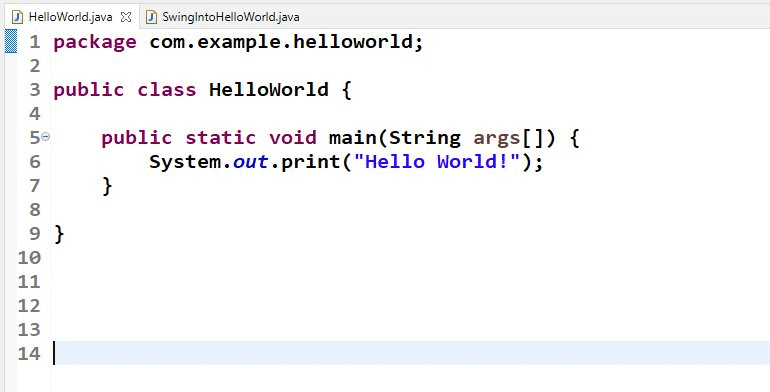
Long-term support releases are rare birds. If you're still working on Java 8 or Java 11, be sure to download the Java 17 LTS release, test your installation with a simple Java Hello World example and explore all the new features and enhancements that have been incrementally added to the JDK.