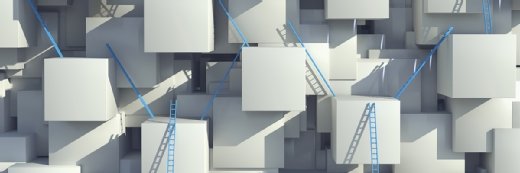
Getty Images
Fix the top 10 most common compile time errors in Java
Flummoxed why your Java code won't compile? Here are the 10 most commonly encountered Java compile errors, along with the fixes that will get your code working in no time.
The types of errors encountered when a software developer develops a Java application can be split into two broad categories: compile time errors and runtime errors. As the name implies, compile time errors occur when the code is built, but the program fails to compile. In contrast, Java runtime errors occur when a program successfully compiles but fails to execute.
If code doesn't compile, the program is entirely unable to execute. As such, it's imperative to fix compile time errors in Java as soon as they occur so that code can be pushed into an organization's continuous delivery pipeline, run and tested.
Compile time errors in Java are a source of great frustration to developers, especially as they try to learn the language. Compile time error messages are notoriously unclear, and troubleshooting such errors can be overwhelming.
To help alleviate the frustrations that compile time error often evoke, let's explore the most commonly encountered compile time errors in Java, and some quick ways to fix them.
Top 10 common Java compile errors and how to fix them
Here are the 10 most commonly encountered Java compile time errors:
- Java source file name mismatch
- Improper casing
- Mismatched brackets
- Missing semicolons
- Method is undefined
- Variable already defined
- Variable not initialized
- Type mismatch: cannot convert
- Return type required
- Unreachable code
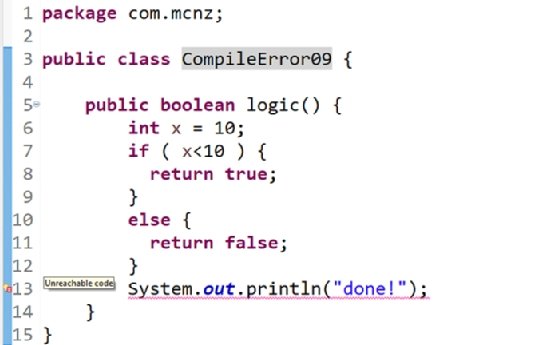
1. Class and source file mismatch
The name of the Java source file, as it resides on the filesystem, must exactly match the name of the public Java class as it is defined in the class declaration. If not, the compiler generates the following Java compile time error:
The public type problemcode must be defined in its own file
A Java class declaration looks like this:
public class ThisWillCompile { }
This Java class must be saved in a file named ThisWillCompile.java -- or else it won't compile.
Java provides no lenience here -- the source filename must exactly match the name used in the class declaration. If the first letter of the file is lowercase but the class declaration is uppercase, the code will not compile. If an extra letter or number pads the name of the source file, the code will not compile.
Many developers who learn the language with a text editor and DOS prompt often run into this problem of name mismatches. Fortunately, modern IDEs, such as Eclipse and IntelliJ, are designed to keep the Java class and the underlying file name in sync.
2. Improper casing
When I first learned to code, nobody told me that Java was case-sensitive. I wasted untold hours as I tried to figure out why the code I meticulously copied from a study guide did not compile. My frustration was palpable when I learned letter casing can be the only difference between compiler success and coding failure.
The Java compiler treats uppercase and lowercase letters completely differently. "Public" is different from "public" which is different from "puBliC." For example, this code will not compile:
Int x = 10;
system.out.println(x);
To make matters worse, the associated Java compile error poorly describes the source of the problem.
Int and system cannot be resolved to a type
To fix the problem, simply make the "I" in "Integer" lowercase and make the "s" in "system" uppercase:
int x = 10;
System.out.println(x);
Java's stringency with upstyling and downstyling letters can frustrate coders who are new to the language. Nevertheless, casing of Java classes and variables means a great deal to other developers who read your code to understand what it does, or what it is supposed to do. As developers deepen their understanding of Java they appreciate the important nuance of properly cased code, such as the use of camel case and snake case.
3. Mismatched brackets
A mismatched brace or bracket is the bane of every programmer. Every open bracket in the code, be it a square bracket, curly bracket or round bracket, requires a matching and corresponding closed bracket.
Sometimes a programmer forgets the closing bracket for a method, or remembers to put in a closing bracket for a method but forgets to close the class. If the brackets don't all match up, the result is a compile time error.
Not only is a mismatched bracket difficult to identify within a complex class or method, but the associated Java compile error can be outright misleading. For example, the following line of code is missing a round brace after the println:
int x = 10;
System.out.println x);
When the compiler attempts to build this code, it reports the following errors, neither of which properly describe the problem:
Duplicate local variable x
System.out cannot be resolved to a type
The fix to this compile error is to add a leading round bracket after the println to make the error go away:
int x = 10;
System.out.println (x);
One way to help troubleshoot mismatched brackets and braces is to format the code. Online lint tools do this, and most IDEs have built-in formatters. It is much easier to identify mismatched brackets and braces in formatted code.
4. Missing semicolons
Every statement in a Java program must end with a semicolon.
This strict requirement can trip up some developers who are familiar with other languages, such as JavaScript or Groovy, which do not require a semicolon at the end of every line of code.
Adding to potential confusion, not every line of Java code is a statement. A class declaration is not considered a statement, so it is not followed by a semicolon. Likewise, a method declaration also is not considered a statement and requires no semicolon.
Fortunately, the Java compiler can warn developers about a missing semicolon. Take a look at the following line of Java code:
System.out.println (x)
The Java compiler points directly at the problem:
Syntax error, insert ';' to complete Statement.
And the code below shows the semicolon is correctly added:
System.out.println (x);
5. Method is undefined
Another issue that often befuddles new developers is when and when not to use round brackets.
If round brackets are appended to a variable, the compiler thinks the code wants to invoke a method. The following code triggers a "method is not defined" compiler error:
int x();
Round braces are for methods, not variables. Removal of the braces eliminates the problem:
int x;
6. Variable is already defined
Developers can change the value of a variable in Java as many times as they like. That's why it's called a variable. But they can only declare and assign it a data-type once.
The following code will generate a "variable already defined" error:
int x = 10;
int x = 20;
Developers also can use the variable "x" multiple times in the code, but they can declare it as an int only once.
The following code eliminates the "variable already defined" error:
int x = 10;
x = 20;
7. Local variable not initialized
Can you tell what's wrong with the following code?
int x ;
System.out.println (x);
Class and instance variables in Java are initialized to null or zero. However, a variable declared within a method does not receive a default initialization. Any attempt to use a method-level variable that has not been assigned a value will result in a "variable not initialized" error.
The lack of variable initialization is fixed in the code below:
int x = 0;
System.out.println (x);
8. Type mismatch
Java is a strongly typed language. That means a variable cannot switch from one type to another mid-method. Look at the following code:
int x = 0;
String square = x * x;
The String variable is assigned the result of an int multiplied by itself. The multiplication of an int results in an int, not a String. As a result, the Java compiler declares a type mismatch.
To fix the type mismatch compile error, use datatypes consistently throughout your application. The following code compiles correctly:
int x = 0;
int square = x * x;
9. Return type required
Every method signature specifies the type of data that is returned to the calling program. For example, the following method returns a String:
public String pointOfNoReturn() {
return "abcdefu";
}
If the method body does not return the appropriate type back to the calling program, this will result in an error: "This method must return a result of a type."
There are two quick fixes to this error. To return nothing from the method, specify a void return type. Alternatively, to specify a return type in the method signature, make sure there is a return statement at the termination of the method.
10. Unreachable code
Poorly planned boolean logic within a method can sometimes result in a situation where the compiler never reaches the final lines of code. Here's an example:
int x = 10;
if ( x < 10 ) {
return true;
}
else {
return false;
}
System.out.println("done!");
Notice that every possible pathway through the logic will be exhausted through either the if or the else block. The last line of code becomes unreachable.
To solve this Java compiler error, place the unreachable code in a position where it can execute before every logical pathway through the code is exhausted. The following revision to the code will compile without error:
int x = 10;
if ( x < 10 ) {
System.out.println("done!");
return true;
}
else {
System.out.println("done!");
return false;
}
Be patient with Java compile errors
It's always frustrating when a developer pushes forward with features and enhancement, but progress is stifled by unexpected Java compile errors. A little patience and diligence -- and this list of Java compile time errors and fixes -- will minimize troubleshooting, and enable developers to more productively develop and improve software features.