How to format a Java String with printf example
How to printf a Java String
To use the Java String printf
method to format output text, follow these rules:
- Use
%s
as theprintf
String specifier in the text string being formatted. - Use
%S
as theprintf
String specifier to output upper-case text. - Precede the letter
s
with a number to specify field width. - Put a negative sign after the
%
to left-justify the text. - Add
%n
any time you wish to include a line break.
Java String printf example
Here is a simple example of how to format a Java String with printf
:
public class JavaPrintfStringExample { /* Java String printf example code. */ public static void main(String[] args) { String name = "Cameron"; String site = "Tss"; System.out.printf("I like the articles %s writes on %S. %n", name, site); /* Output: I like the articles Cameron writes on TSS. */ } }
The example above outputs the following text:
I like the articles Cameron writes on TSS.
This is a simple example of how to format a Java String with printf
, but it also demonstrates two interesting features of the syntax:
- The use of
%s
(lowercase s) preserves the casing of the name ‘Cameron’ - The use of
%S
(uppercase S) makes the casing of Tss uppercase.
The use of %n
forces a line break, which isn’t seen in the output of this example, but it is an important element to include when formatting paragraphs of text.
Benefits of the Java String printf method
The Java String printf
method can be confusing at first, but it greatly simplifies how you format complex Strings. This is especially true when creating complicated database, NoSQL or Hibernate queries.
Compare the Java String printf
example above with how we would have to format a text String without printf
. The following code snippet demonstrates the difference between a String formatted with printf
, and one created through String addition:
Format output with Java printf tutorials |
---|
Here are some great tutorials and examples on how to use printf to format Java output.
|
String name = "Cameron";
String site = "Tss";
/* The following line uses the Java String printf syntax. */
System.out.println( "I like the articles " + name + " writes on " + site + "." );
/* The following line uses the Java String printf syntax. */
System.out.printf( "I like the articles %s writes on %S.%n", name, site );
Even with this simple example, you can see how the Java printf
statement formats Strings that are much easier to digest. As Strings become increasingly complicated, Java’s printf
method makes these Strings significantly easier to build.
Java String printf syntax
The format for the Java printf
function is as follows:
% [flag] [width] specifier-character (s or S)
The flag most typically used with a Java String printf
statement is the negative sign, which left-aligns the text.
The width specifier is an integer that specifies how many spaces should be dedicated to displaying the String. If the String is shorter than the number specified, whitespace is displayed adjacent to the String.
printf flag | Purpose |
%s | Display the text with upper and lowercasing preserved |
%S | Display the text in all uppercase letters |
%-s | Alight the text to the left, with String casing preserved |
%20s | Allocate 20 spaces to display the right-aligned text |
%-10S | Allocate 20 spaces to display the left-aligned text and use uppercase lettering |
Java String printf table
A common way to demonstrate advanced Java printf
String usage is to format a table of data.
The advanced Java String printf
example below will generate the following table of data:
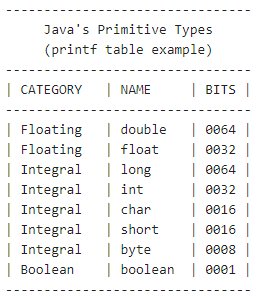
The data table generated from the Java printf method example.
Java String printf table example
Here is the full code for the advanced Java String printf
table example:
System.out.printf("--------------------------------%n"); System.out.printf(" Java's Primitive Types %n"); System.out.printf(" (String printf example) %n"); System.out.printf("--------------------------------%n"); System.out.printf("| %-10s | %-8s | %4s |%n", "CATEGORY", "NAME", "BITS"); System.out.printf("--------------------------------%n"); System.out.printf("| %-10s | %-8s | %4s |%n", "Floating", "double", "0064"); System.out.printf("| %-10s | %-8s | %4s |%n", "Floating", "float", "0032"); System.out.printf("| %-10s | %-8s | %4s |%n", "Integral", "long", "0064"); System.out.printf("| %-10s | %-8s | %4s |%n", "Integral", "int", "0032"); System.out.printf("| %-10s | %-8s | %4s |%n", "Integral", "char", "0016"); System.out.printf("| %-10s | %-8s | %4s |%n", "Integral", "short", "0016"); System.out.printf("| %-10s | %-8s | %4s |%n", "Integral", "byte", "0008"); System.out.printf("| %-10s | %-8s | %4s |%n", "Boolean", "boolean", "0001"); System.out.printf("--------------------------------%n");
Output with Java String printf
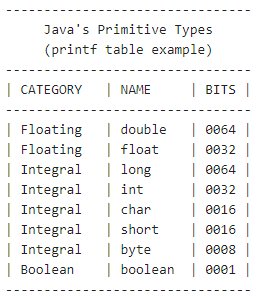
How to format a String with Java printf method calls.
When the code runs, the output is a simple table that displays information about the 8 Java primitive types.
It also provides a great example of how to use the Java printf
method to format String-based output.