How to format a Java table with printf example
Print and format a table with printf
Sometimes it’s nice to format the output of a console based Java program in a friendly way.
The java.lang package has no built-in table generators, but you can easily present data in a chart-based format if you creatively use Java’s printf
method with dashes, pipelines and printf
field specifiers.
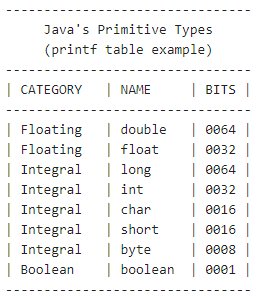
A simple, Java printf table showing primitive type details.
To demonstrate how to format a table with Java printf
statements, let’s create a chart that displays information about Java’s 8 primitive types.
Above is how the finished printf
table will look when we are done. Let’s build toward that as the goal.
Java printf table specifications
We will format the Java printf
table according to the following rules:
- Dashed lines act as row separators.
- Pipelines separate columns.
- Each cell have a single space of padding on the left and right.
- The first column is 10 spaces wide, plus padding.
- The second column is 8 spaces wide, plus padding.
- The third column is 4 spaces wide, plus padding.
- Integer values in the 3rd column are zero padded to four digits.
Create the printf table title
We can easily hardcode the title:
System.out.printf("--------------------------------%n"); System.out.printf(" Java's Primitive Types %n"); System.out.printf(" (printf table example) %n"); System.out.printf("--------------------------------%n");
The only printf
placeholder used in the first four lines is %n
, which simply causes a carriage return that puts subsequent output on a new line.
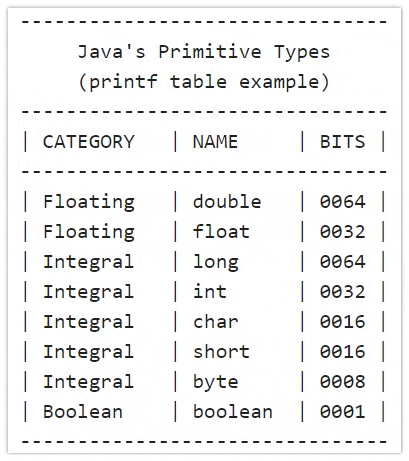
This image shows the values used to create our table with Java printf statements.
Code the printf table’s heading
The guts of the printf
table will use the following three placeholders:
%-10s
to allocate 10 spaces for text String display. The minus signs forces left justification.%-8s
to allocate 8 spaces for text String display. Again, the minus signs forces left justification.%04d
, to allocate 4 spaces to display the digits. The 0 in%04d
causes zero fills when a number is not 4 digits long.
With the added pipeline characters and padding, the Java printf
line to format the table heading looks as follows:
System.out.printf("| %-10s | %-8s | %4s |%n", "CATEGORY", "NAME", "BITS");
Java printf table body example
The following is the complete code for the Java printf
table example’s body:
System.out.printf("--------------------------------%n"); System.out.printf(" Java's Primitive Types %n"); System.out.printf(" (printf table example) %n"); System.out.printf("--------------------------------%n"); System.out.printf("| %-10s | %-8s | %4s |%n", "CATEGORY", "NAME", "BITS"); System.out.printf("--------------------------------%n"); System.out.printf("| %-10s | %-8s | %04d |%n", "Floating", "double", 64); System.out.printf("| %-10s | %-8s | %04d |%n", "Floating", "float", 32); System.out.printf("| %-10s | %-8s | %04d |%n", "Integral", "long", 64); System.out.printf("| %-10s | %-8s | %04d |%n", "Integral", "int", 32); System.out.printf("| %-10s | %-8s | %04d |%n", "Integral", "char", 16); System.out.printf("| %-10s | %-8s | %04d |%n", "Integral", "short", 16); System.out.printf("| %-10s | %-8s | %04d |%n", "Integral", "byte", 8); System.out.printf("| %-10s | %-8s | %04d |%n", "Boolean", "boolean", 1); System.out.printf("--------------------------------%n");
When the code runs, a handsome Java printf
table displays, showing a chart of the eight Java primitive types, along with interesting details about each of them.
Format output with Java printf tutorials |
---|
Here are some great tutorials and examples on how to use printf to format Java output.
|