long to String in Java
How to convert a long to a Java String
The easiest way to convert from a long to a String in Java is to add the long to an empty set of double quotes with the plus operator.
// long to String in Java example code long longToConvert = 90210L; String longAsString = "" + longToConvert; System.out.println( "Converted long to String length " + longAsString.length() );
Convert from long to String in Java
If the empty String addition trick is not to your liking, rest assured there are many ways to convert a long to a String in Java. Options include the following:
- Add it to an empty String, “” + longToConvert.
- The String class’ valueOf method, String.valueOf( longToConvert ).
- The Long class’ toString method, Long.toString( longToConvert ).
- The String class’ format method, String.format( “%d”, longToConvert ).
- Use DecimalFormat, new DecimalFormat(“#”).format(longToConvert).
The best solution is the simplest one
The best solution, as is often the case, is the simplest solution. There is no shame to simply use the plus operator and add a long to an empty String to get the JVM to convert a long to a String for you.
Use the String class or the Long class to perform a long to String conversion, and you’ll create unwanted code bloat for your fellow programmers.
It might look more object-oriented, but unless you’re doing really complex String manipulations that demand the use of the StringBuilder class, a quick concatenation of an empty String with a long primitive type is the way to go.
Example long to String conversion code
Here’s a JShell script you can run that demonstrates the five approaches to the long to String conversion problem listed above:
// Five ways to convert a long to String in Java long longToConvert = 90210L; String longAsString = ""; longAsString = "" = longAsString; longAsString = String.valueOf( longToConvert ); longAsString = Long.toString( longToConvert ); longAsString = String.format( "%d", longToConvert ); longAsString = new java.text.DecimalFormat("#").format(longToConvert); // Print out the length of the long converted to a String in Java System.out.println( "Converted long to Java String length " + longAsString.length() );
Add a long inside a text String
The easiest way to convert a long to a String is to append it to double quote. However, for complex output where a long must be embedded in a Java String, use the printf or String.format method.
Compare the JShell program below, which first uses the plus operator to add a long inside a String, and then the printf option second:
// long to String conversion with printf long longToConvert = 90210L; String longAsString = ""; System.out.println("The long is " + longToConvert + " now in the String"); System.out.printf("The long is %d now in the String", longToConvert);
The use of printf makes embedding primitive types like a long in a String much easier.
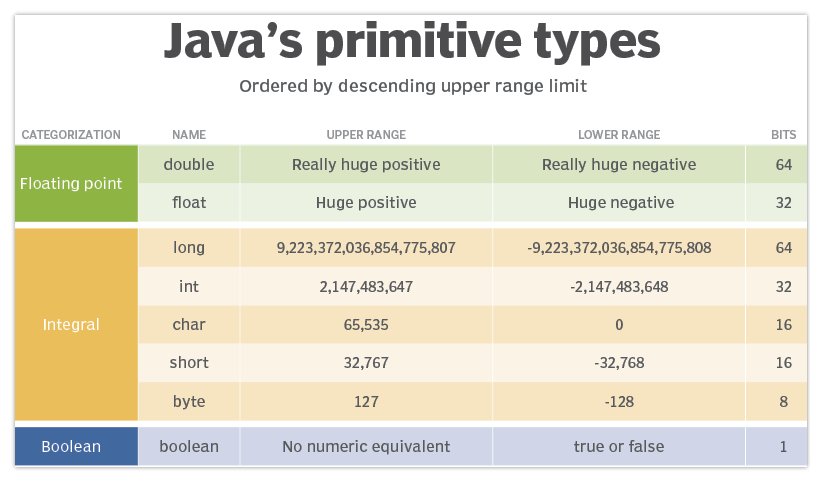
The conversion of a long to a String with the plus operator works with any of the eight Java primitive types.
By the way, you can also go the other way. Here are several ways to convert a String to a long in Java.