User input with Java's Console class
Java Console class input and ouput
The easiest way to garner user input in a Java program is to use the System’s Console class.
Introduced in Java 6, Java’s System Console class provides two simple methods to obtain user input:
readLine() readPassword()
Java user input with readLine
The readLine()
method takes user input through the console window, stores the input data in a String, and echoes the input back to the user as he or she types.
The Console’s readPassword()
method performs the same function as readLine()
, with the following two exceptions:
readPassword()
does not echo text back while the user typesreadPassword()
encrypts the user input so it cannot be read as plain text
User input in Java example
Here’s a quick example of how to use the System Console to get user input in Java:
public class JavaUserInput { public static void main(String[] args) { System.out.print("Enter some user input: "); String input = System.console().readLine(); System.out.print("You entered: " + input); } }
Output with System’s Console printf method
To use the Java Console class for both input and output, replace all the System.out.print
statements with the Java Console object’s printf
method:
public class JavaUserInput { public static void main(String[] args) { System.out.prinf("Enter some user input: "); String input = System.console().readLine(); System.out.prinf("You entered: " + input); } }
How to format console output in Java
Note the letter ‘f’ in the Java Console’s printf
method.
The difference between print()
and printf()
is the ability to easily output formatted text.
To demonstrate, replace the line which adds the input String to the “You Entered: “ text and use a special, formatted output String instead, like this:
Java user input made easy |
---|
Learn the easiest ways to handle user input in Java, and format any console output with printf.
|
public class JavaUserInput { public static void main(String[] args) { System.out.print("Enter some user input: "); String input = System.console().readLine(); System.out.print("You entered: %s", input); } }
When you need to output complex text Strings to Java’s console window, the printf
method can be extremely helpful.
Scanner vs Console for user input in Java
Many “introductions to Java” tutorials often use the Scanner class or even worse, the InputStream class.
Both of those approaches work, but they are needlessly verbose and confusing to Java newcomers.
Sadly, many of the tutorials you see online are stuck in 2005, when AWS had only one service and 10 years before Kubernetes was even invented.
If you are a course designer, put Java’s Scanner and InputStream classes on hold. Respect the learner and make their introduction to the language easier through the use of Java’s Console class.
When you present the concept of Java user input, introduce the System Console object first. It will make the learner’s experience much more pleasant.
Cannot invoke java.io.Console.readLine() Eclipse error
If you try this example in the Eclipse IDE, you may run into the following error:
Eclipse causes a java.lang.NullPointerException: Cannot invoke "java.io.Console.readLine()" because the return value of "java.lang.System.console()" is null Console Java User Input Example Failed
This is because Eclipse uses the javaw.exe program to run Java code, not the java.exe command.
The ‘w’ in ‘javaw’ indicates that the program will run without access to the Java Console. As a result, a NullPointerError ensues.
If you use an online editor such as Replit, or run the code from the command line, Java Console-based user input and output won’t be a problem.
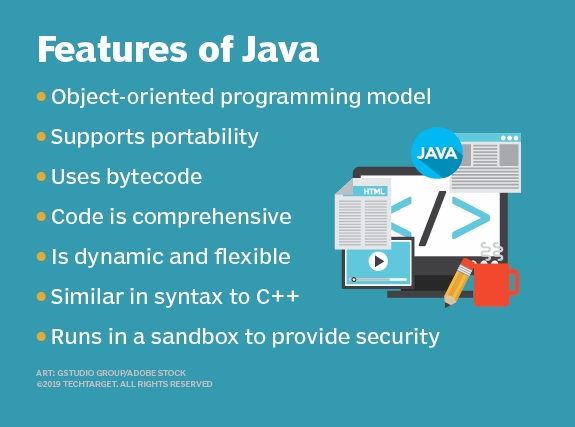
Java has many great features. Simplified console-based user input is now one of them.