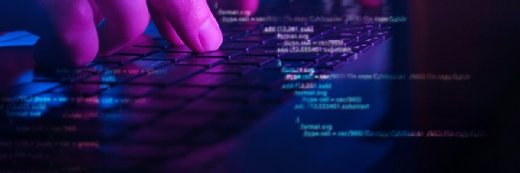
Getty Images/iStockphoto
Your top 4 Java user input strategies
From System.in to the Scanner class, there are many ways to read user input into your Java programs. Find out which Java user input strategy is best for your software needs.
One of the first tasks new developers must learn is how to read user input into the programs they write.
In Java, you can read user input into a standalone application through one of four ways:
- Use the globally accessible Java Console object.
- Create an instance of the Java Scanner class.
- Render a Swing JOptionPane.
- Pass Java's System.in to an InputStream.
Let's explore each of these Java user input strategies in more detail.
1. User input with the Java console
By far, the easiest way to read user input in Java is with the Console class. Here's how it looks:
var input = System.console.readLine();
System.out.println("You typed in: " + input);
The Console class is simple and easy to use. It is accessed through the universally available System object, and it requires no imports or newly created objects. New developers love it.
The main drawback to this approach is that some Java runtime environments turn off the Console feature. Eclipse and the Spring Tool Suite, for example, both disable the Console function, so this class won't work in those IDEs.
2. User input with the Java Scanner
The most common way to get Java user input is with the Scanner class. To use the Scanner class, there are three steps:
- Import the java.util package.
- Create a new instance of the Scanner.
- Pass in to the Scanner's constructor.
Here's how it looks in code:
System.out.println("What is your name?"); Scanner scanner = new Scanner(System.in); String name = scanner.nextLine(); System.out.println(name + " is a nice name!");
The Scanner class works in all environments, including ones where the Console class fails.
The Scanner class also includes several utility methods to process int, float, boolean and string-based input.
The only drawback to the Java Scanner option is that its semantics can intimidate new developers.
3. User input with the JOptionPane
My favorite way to read input from the user is to use the JOptionPane. It's easier to use than the Scanner class, and it generates an interactive dialog box that prompts the user for input.
String prompt = "Will it be rock, paper or scissors?";
var input = JOptionPane.showInputDialog(prompt);
System.out.println("This time you said " + input);
The only drawback to the JOptionPane is that you must import the javax.swing package, but that's not an overly difficult task for a new developer to overcome.

4. User input with Java's System.in
In earlier versions of Java, developers could only obtain user input by chaining Java I/O classes, as follows:
InputStreamReader reader = new InputStreamReader(System.in);
BufferedReader br = new BufferedReader(reader);
System.out.println("What is your name?");
var input = br.readline();
System.out.println("Your input was: " + input);
This is not a recommended Java user input approach, especially for developers who are new to the language. The code requires imports, and it is needlessly verbose and difficult to understand.
Avoid this approach to Java user input, unless the goal is to teach developers how classes in the Java I/O package work.
Learning Java is more fun for new developers if they can build interactive programs. When you need to develop a standalone application, these are the best four Java user input strategies: a Java Console object, Java Scanner class, Swing JOptionPane or Java's System.in.