An introduction to RPA programming with UiPath Studio
The promise of RPA software is that it can create reusable and repeatable tasks quickly and easily.
Companies such as UIPath, Automation Everywhere and Blue Prism provide 'low-code' or 'no-code' development environments to make these products as egalitarian as possible, allowing both experienced and junior robotic process automation (RPA) developers to create and deploy automated robots.
Let's put UIPath's low-code approach to the test by building a number guesser application with its RPA programming tool.
To follow along, first install the IDE and create a basic UiPath Hello World application to become familiar with the tool.
How to learn RPA programming
An RPA programmer must learn the following to master the basic tenets of RPA development:
- Variable initialization techniques;
- Conditional logic with if-then-else statements;
- Iterative logic with the use of for, each and while loops;
- Interactive input and output routines; and
- The use of try catch blocks to implement UiPath error handling.
After an RPA programmer masters the aforementioned building blocks, it won't be difficult to create UiPath robots to perform invoice data extraction, OCR image processing or email automation.
RPA project creation
All RPA programming in a UiPath Studio starts with new project creation. In this UiPath Studio tutorial, we started a new UiPath process project named RPA Number Guesser.
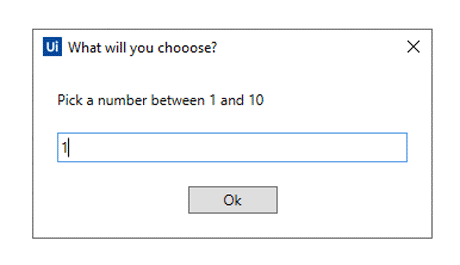
Variable initialization in UiPath Studio
The application requires three variables, which means the Multiple Assign activity needs to be dragged from the Activities tab onto the main workflow window. With this activity, create the following variables:
- A variable named count of type int32 -- initialize it to the number 0;
- A variable named secretKey of type int32 -- initialize it to 5; and
- A variable named guess of type int32.
The secretKey will be the number that the user will try to guess. The count will keep track of the number of guesses. The variable named guess will temporarily store the user's input.
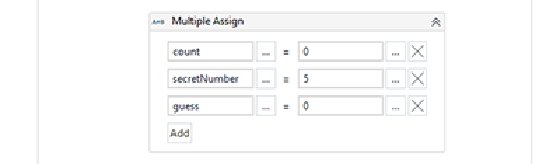
UiPath Studio do-while loops
To iteratively ask the user to guess the secret number, a programmer will need to drag a do-while loop under the Multiple Assign activity. The loop will execute so long as the secretNumber is not equal to the user's guess, which means entering the following logic for the loop's condition:
guess <> secretNumber
Within the do-while loop, add an Input Dialog activity to prompt the user for a number and store the response in the variable guess.
For the input dialog, assign the following values:
- Set the Label to "Pick a number between 1 and 10".
- Set the Title to "What will you choose?".
- Set the Result to guess.
Right after the program asks the user to guess, add an Assign activity and increment the count. In the Assign activity set the count to count+1.
UiPath Studio conditional statements
With the user's guess stored in a variable, the application requires conditional statements to tell the user to guess higher or lower if necessary. Kick off the conditional logic with the addition of the If activity to the sequence.
This first If statement will simply check if the user guessed the correct number. Add the code to the condition of the If activity as follows:
guess <> secretNumber
In the Then clause, add a nested If condition and indicate whether the user should guess higher or lower. For the condition of the nested If, check to see whether the guess is less than the secret. Add a Message Box to both the Then and Else conditions that tells the user to guess higher or lower.
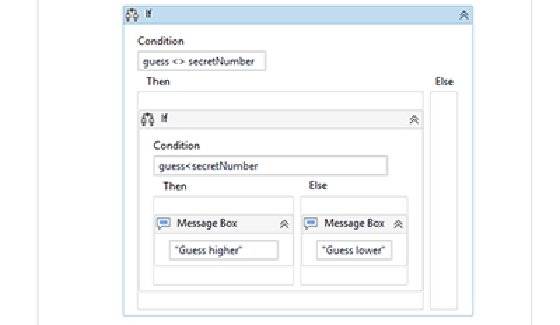
Complete the RPA programming app
The last operation to add to our application is to put a Message Box activity after the Do While activity that tells the user how many times it took them to guess correctly. The content of the Message Box would be as follows:
"Correct! It took you " + count.ToString + " guesses."
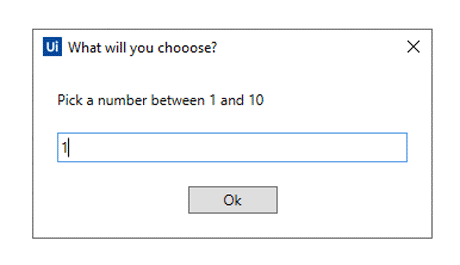
With all this complete, a programmer can run the RPA program. The number guessing game will play out, and when the user guesses the number five, the program will trigger a window that tells the user they correctly guessed the secret number.
Here's how the completed RPA programming project looks when it is completed.
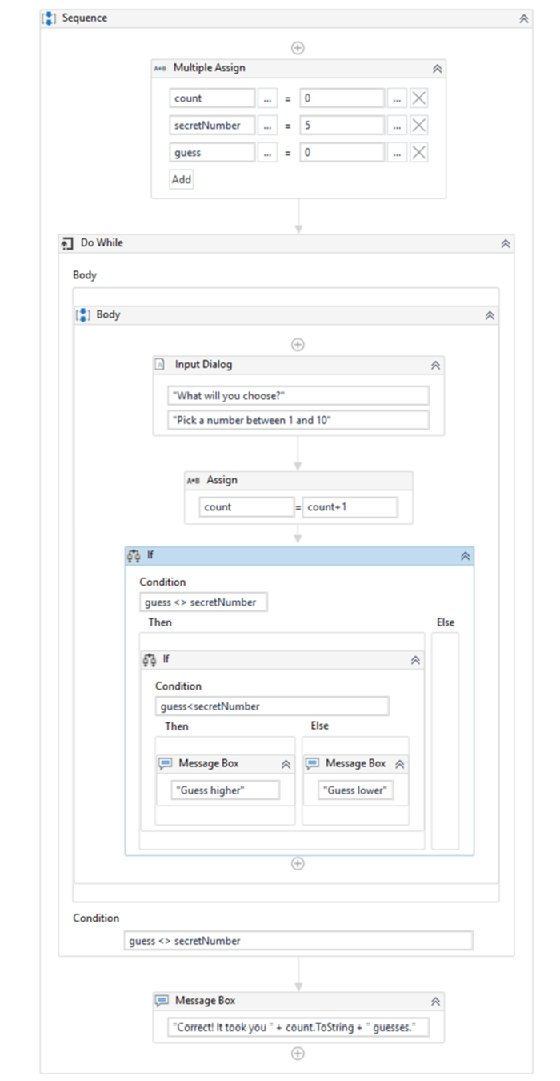
UiPath exception handling
This application has one small bug. If a user provides non-numeric input to the published UiPath app, it triggers an exception. There are many ways to perform input validation, but a simple solution for this example is to put the entire do-while loop in a try catch block. A programmer can accomplish this by adding the UiPath Try Catch activity to the page. Move the loop into the body of the try, and then catch the System.Exception.
Continued learning
If you would like to improve your RPA programming skills, build on this UiPath Studio tutorial by creating a few projects of your own. I always recommend developers use the concepts taught here and see if they can write a rock-paper-scissors type of application. The completion of such a project on your own will help reinforce the software development fundamentals and take you one step further in your journey to master and learn RPA programming skills.
You can find the source code for this UiPath Studio tutorial on GitHub.