Java Scanner import
How do you import the Java Scanner class?
There are two ways to implement the Java Scanner import: explicitly reference the java.util.Scanner
package and class in the import, or do a wildcard import of java.util.*
.
Here is how the two Java Scanner import options look:
-
import java.util.Scanner; // explicit Scanner import
-
import java.util.*; // wildcard Scanner import
The import statement must occur after the package declaration and before the class declaration.
What does import java.util Scanner mean?
The java.util.Scanner
class is one of the first components that new Java developers encounter. To use it in your code, you should import it, although another option is to explicitly reference the package in your code.
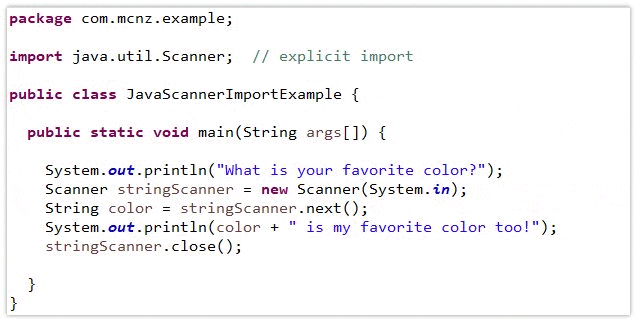
There are multiple ways to import the Java Scanner class into your code.
Java’s Scanner class makes it easy to get input from the user, which allows simple programs to quickly become interactive. And a little bit of interactivity always makes learning how to program a computer just a little bit more fun.
However, there is one minor complexity the Java Scanner class add into the software development mix.
In order to use the Java Scanner class in your code, you must either fully reference the java.util package when you call the Scanner, or you must add a Java Scanner import statement at the start of your class.
To keep your code readable and less verbose, a Java Scanner import is recommended.
When you add an import statement to your code, you are telling the Java compiler that you need access to a class that isn’t accessible by default. The java.util.Scanner
import statement at the top of many Java classes means that somewhere in the code, the Scanner class is being used.
Java user input made easy |
---|
Learn the easiest ways to handle user input in Java, and format any console output with printf.
|
Java Scanner import example
Here’s an example of an application that uses an explicit Java Scanner import so that we can make the program interactive with user input:
package com.mcnz.example; import java.util.Scanner; public class ScannerUserInput { public static void main(String[] args) { // String input with the Java Scanner System.out.println("How old are you?"); Scanner stringScanner = new Scanner(System.in); String age = stringScanner.next(); System.out.println(age + " is a good age to be!"); } }
Why must we import the Java Scanner class?
With no added import statements to your code, your Java app has default access to all of the classes in the java.lang package. This includes classes such as:
- String
- System
- Integer
- Double
- Math
- Exception
- Thread
However, to use any classes in packages other than java.lang in your code, an import is required.
The Scanner class is found in the java.util package, not java.lang.
Since the Scanner class is found outside of java.lang, you must either directly reference the java.util package every time you use the Scanner, or just add a single Scanner import statement to your Java file.
How do you use the Java Scanner without an import?
Here’s an example of how to avoid a Java Scanner import and instead directly reference the package when the Scanner is used:
package com.mcnz.example; // Notice how the Java Scanner import is removed public class ScannerUserInput { public static void main(String[] args) { System.out.println("How old are you?"); // With no Scanner import, an explicit java.util reference is needed java.util.Scanner stringScanner = new java.util.Scanner(System.in); String age = stringScanner.next(); System.out.println(age + " is a good age to be!"); } }
Notice how the code becomes a bit more verbose, as the package reference adds bloat to the line of code where the Scanner is first declared.
Both the Java Scanner import and an explicit package reference are valid options to access the class. Which option a developer chooses comes down to which approach they believe makes their code the most readable and maintainable.
What is a wildcard import in Java?
There are over 100 classes in the java.util package.
When you import the Java scanner with the import java.util.*;
statement, you gain access to each class in the java.util package without adding any more import statements.
In contrast, when an explicit Java Scanner import is performed with the import java.util.Scanner;
statement, only the Scanner class becomes available to your code. To use other classes in the java.util package, you must add explicit imports of those classes.
For the sake of simplicity, I recommend new developers use the wildcard approach wto import the Java Scanner class. It requires fewer keystrokes and reduces the opportunity to introduce compile-timer errors into your code.
package com.mcnz.example; // This example uses the wildcard import syntax import java.util.*; public class ScannerUserInput { public static void main(String[] args) { // String input with the Java Scanner System.out.println("How old are you?"); Scanner stringScanner = new Scanner(System.in); String age = stringScanner.next(); System.out.println(age + " is a good age to be!"); } }
Furthermore, if you use an IDE such as Eclipse or VS Code, an import formatter will convert wildcards imports to explicit imports when you finish development.
Senior developers find that implicit imports lead to more readable code, and also avoid possible import collisions when a class appears in two separate packages. For example, the Date class exists in both the java.util and java.sql packages, which can lead to a great deal of confusion if an application uses both packages.
Does a wildcard import hurt performance?
Some developer think doing a java.util.*;
import might impact the performance of their code because so many classes become available to your program. However, this is not true. The wildcard import simply makes every class in a package available while you develop your app. It has no impact on the size of the application that eventually gets built.
What happens if you don’t import the Scanner?
If you attempt to use the Scanner class but fail to add an import, or don’t explicitly reference the package and the class together, you will encounter the following error:
Error: Scanner cannot be resolved to a type
The “cannot be resolved to a type” compile time error may sound somewhat confusing to a new developer. All it’s saying is that you have referenced a class that is outside of any package referenced through an import statement.
If you get the “Scanner cannot be resolved to a type” error message, just add the Java Scanner import statement to your code, or explicitly reference the package when you use the Scanner class in your code.